Write a Function to Check Whether the Given Number is Even or Odd
In this program, we will learn to check whether the given number is odd or even. If it is even then a print list of event numbers if it is odd then print all odd numbers.
Algorithm and Flowchart Exercises
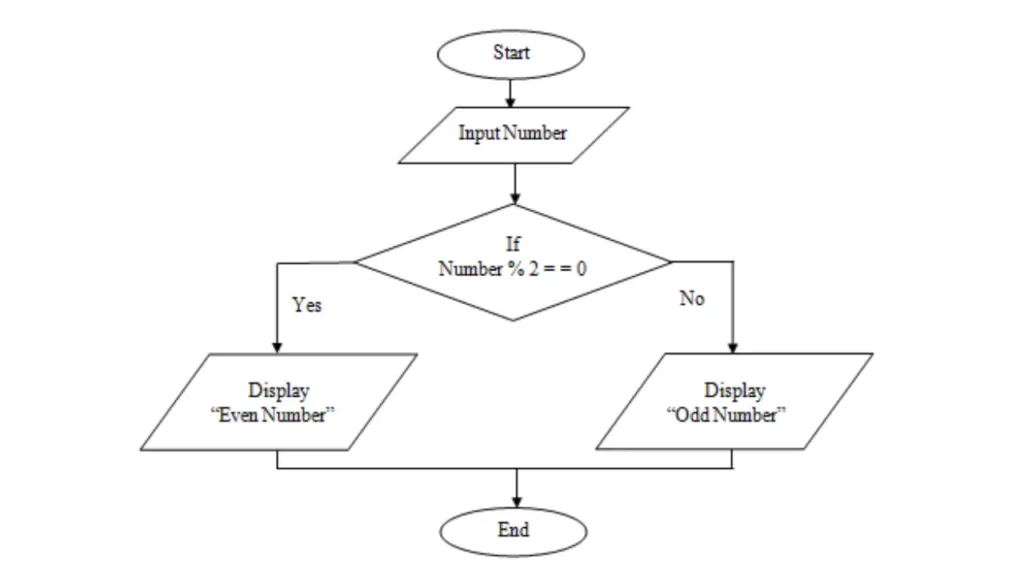
Odd and Even Number: Algorithms for beginners
- Start.
- Get value from a user or assign value statically.
- num=list(input(‘number’).split(“,”)) # num = [1,2,3,4,5,6,7,9]
- if num % 2 == 0 then
- Print Even Number
- Else:
- Print Odd Number
- end
Even Odd Program in Python using Function
def odd_even(num):
odd=[]
even=[]
for i in num:
if i%2 == 0:
even.append(i)
else:
odd.append(i)
print(f"odd number are {odd} and even number are {even}")
# Your can Use return also but format of out will be different
# output_num = odd, even
# return output_num
number=[1,2,3,12,15,6,7,8,9]
print(odd_even(number))
Output:
odd number are [1, 3, 15, 7, 9] and even number are [2, 12, 6, 8]
None
Odd or Even Program in Python using For Loop
number2=[1,2,3,4,5,6,8,9]
for i in number2:
if i % 2==0:
print("even number are :",i)
else:
print("odd number are :",i)
Output:
odd number are : 1
even number are : 2
odd number are : 3
even number are : 4
odd number are : 5
even number are : 6
even number are : 8
odd number are : 9
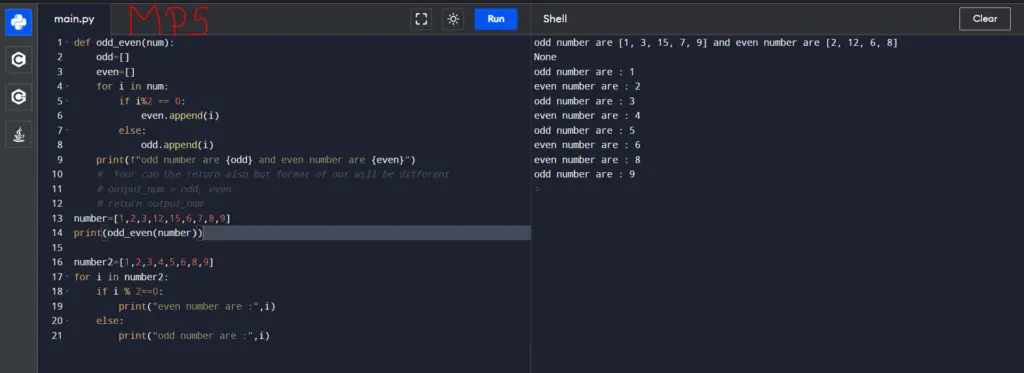
To know more about odd and even number you can watch this video
FAQ of add and even
How do you check if the number is odd or even in Python?
To check whether the given number is even or odd, we have to divide the number. Example: Number % 2 == 0 then we can say that is an even number else odd number.
How do you define odd and even numbers in Python?
If a Number is divisible by 2 then we can say an even number and if a number is not divisible by 2 then we can say an odd number.
What is an example of an odd number?
The example of odd numbers are 1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29, 31, 33, 35, …… and so on
Is 0 an odd number?
No, 0 is not an odd number. it is an even number. Let me show you an example:
number2=[0,1,2,3,4,5,7]
for i in number2:
if i % 2==0:
print(“even number are :”,i)
else:
print(“odd number are :”,i)
Output:
even number are : 0
odd number are : 1
even number are : 2
odd number are : 3
even number are : 4
odd number are : 5
odd number are : 7What is the sum of odd numbers?
The sum of some odd numbers is odd and some odd number is even also. Example: 3 + 3 = 9 (odd) and 5 + 5 = 10 (even)
Which is the first even number?
The first even number is 0,2,4,6,8 and so on. The event lies between 0 to 20 are 0,2,4,6,8,10,12,14,16,18 and 20. In the same way, you can see 2 and 4, and -6 and -4 are consecutive even numbers.
can an odd function have a constant
Yes, you can.
Suppose you have an odd function that takes one parameter ( x ) and returns a number.
Let’s say that the function is f(x) = 3x^2 + 6x – 7.
The graph of this function looks like this:
We can write it as f'(x) = 3(3x^2 + 6(3x – 7)) = 9x – 5Why is the sum of an odd and even number odd?
The sum of edd and even number is always odd because if you add 0 and 1 is 1 and this is odd, if you add 2 and 3 is equal to 5 and this is also even.
Recommended Posts:
- How to Become a Machine Learning Engineers – easy Step by Step Guide
- Python Difference Between Two Lists using Python Set and Without Set
- How to Open file in python|Read File Line by Line with Examples
- Python String Find() Method Explained with Examples
- How to Generate Random Numbers in Python with Examples
Get Salesforce Answers