How to reverse a number in python?
In this program, we will learn to find the reverse of the given number.
Algorithm and Flowchart Exercises
First, we will see the algorithm to find the largest number, and then we will see exercises
A flowchart:
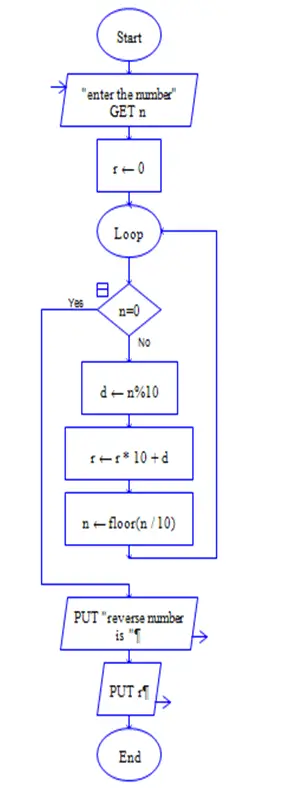
Algorithms for beginners
- Step 1: Start
- Step2: Declare the variable n and read n.
- Step3: R R R with 0.
- Step4: Perform the following steps in the loop until the condition n = 0 is false.
- d = n% 10
- R = r * 10 + d
- N = floor (n / 10)
- Step 5: Print reverse number r if the condition is correct.
- Step 6: End
Source Code: Display the reverse of a number in python
In this we have to find the reverse of the given number example: number = 12345 then output is number_2 = 54321
x = input ( " enter the number :" ) r = list(reversed(x)) print ("Number :" ,x ) print ("Reversed :" ,r )
Output:
enter the number: 12345 Number: 12345 Reversed: ['5', '4', '3', '2', '1']

Reverse the number using for loop in python
x = input ( " enter the number :" ) y = list(x) for i in y[::-1]: print(i)
Output:
enter the number :12345 5 4 3 2 1
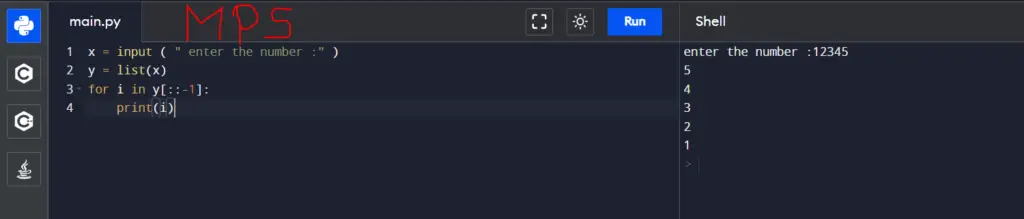
FAQ:
What does reverse() do in Python?
In python reverse count the element from the last ( value[:-1] ) and print the value one by one. It means reversing the value which is passed as an argument in the reversed() function.
What is the reversed word in Python?
Reversed is a function in python, which is used to reverse the data. Example:
input value = ‘Apple’ then output = elppA
How do you reverse a set in Python?
To reverse a set first, we have to take input as a string and perform type conversion as a set. then reversed the set and then print it.
x = input ( ” enter the number :” )
y = set(reversed(x))
print(y)
Can you reverse a string in Python?
Yes, we can reverse a string in python, and to reverse we have to follow this way:
x = input ( ” enter the number :” )
y = reversed(x)
print(y)
# 2nd way
x = input ( ” enter the number :” )
print(x[::-1])
Recommended Posts:
- How to Become a Machine Learning Engineers – easy Step by Step Guide
- Python Difference Between Two Lists using Python Set and Without Set
- How to Open file in python|Read File Line by Line with Examples
- Python String Find() Method Explained with Examples
- How to Generate Random Numbers in Python with Examples
Get Salesforce Answers