In this tutorial, we’ll describe a number of methods in Python to learn & open files in python line by line with examples such as utilizing readlines(), context supervisor, while loops, and so on. After this, you possibly can undertake one of these strategies in your initiatives that matches the perfect circumstances.
Python has made File I/O super simple for programmers. However, it is for you to determine what’s probably the most environment-friendly approach for your scenario. It would depend upon many parameters, such as the frequency of such an operation, the scale of the file, and so on.
Let’s assume we have a logs.txt file that resides in the identical folder alongside the Python script.
Various approaches to Open Files in Python Line by Line
We’ll now go over each of the strategies to learn a file line by line.
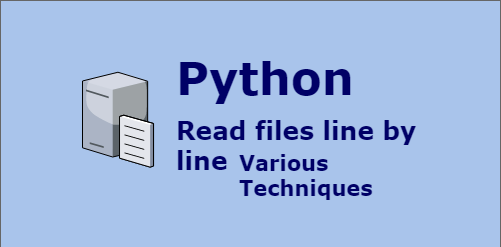
Readlines() to learn (open file in python) all strains collectively
We advocate this answer for information with a smaller dimension. If the file dimension is massive, then it turns inefficient as it masses your complete file in reminiscence.
However, when the file is small, it is simpler to load and parse the file content material line by line.
The readlines() return a sequence of all strains from the file containing the newline char except the last one.
We’ve demonstrated using readlines() to operate in the beneath instance. Here, you possibly can see that we’re additionally utilizing the Python while loop to traverse the strains.
Example
""" Example: Using readlines() to open file in python and learn all strains in a file """ ListOfLines = ["Python", "CSharp", "PHP", "JavaScript", "AngularJS"] # Function to create our take a look at file def createFile(): wr = open("Logs.txt", "w") for line in ListOfLines: # write all strains wr.write(line) wr.write("n") wr.shut() # Function to demo the readlines() operate def learnFile(): rd = open ("Logs.txt", "r") # Read record of strains out = rd.readlines() # Close file rd.shut() return out # Main take a look at def primary(): # create Logs.txt createFile() # learn strains from Logs.txt outList = learnFile() # Iterate over the strains for line in outList: print(line.strip()) # Run Test if __name__ == "__main__": primary()
The output is as follows:
Python CSharp PHP JavaScript AngularJS
On the opposite hand, the above answer will trigger excessive reminiscence utilization for massive information. So, you must select a unique strategy.
For occasion, you might like to try this one.
Readline() to Open file in Python line by line
When the file dimension reaches MBs or GB, then the suitable concept is to get one line at a time. Python readline() technique does this job effectively. It does not load all information in one go.
The readline() reads the textual content till the newline character returns to the road. It handles the EOF (finish of file) by returning a clean string.
Example
""" Example: Using readline() to open file in python and learn a file line by line. """ ListOfLines = ["Tesla", "Ram", "GMC", "Chrysler", "Chevrolet"] # Function to create our take a look at file def createFile(): wr = open("Logs.txt", "w") for line in ListOfLines: # write all strains wr.write(line) wr.write("n") wr.shut() # Function to demo the readlines() operate def learnFile(): rd = open ("Logs.txt", "r") # Read record of strains out = [] # record to save strains while True: # Read subsequent line line = rd.readline() # If line is clean, then you definitely struck the EOF if not line : break; out.append(line.strip()) # Close file rd.shut() return out # Main take a look at def primary(): # create Logs.txt createFile() # learn strains from Logs.txt outList = learnFile() # Iterate over the strains for line in outList: print(line.strip()) # Run Test if __name__ == "__main__": primary()
After execution, the output is:
Tesla Ram GMC Chrysler Chevrolet
Reading files utilizing Python context supervisor
Python offers an idea of context managers. It includes utilizing the “with” clause with the File I/O capabilities. It retains the monitor of the open file and closes it robotically after the file operation ends.
Hence, we will verify that you simply by no means miss closing a file handle utilizing the context supervisor. It performs cleanup duties like closing a file accordingly.
In the beneath instance, you possibly can see that we’re utilizing the context supervisor (with) alongside the for loop to first write and then study strains.
Example
""" Example: Using context supervisor and for loop open file in python to learn it. """ ListOfLines = ["NumPy", "Theano", "Keras", "PyTorch", "SciPy"] # Function to create take a look at log utilizing context supervisor def createFile(): with open ("testLog.txt", "w") as wr: for line in ListOfLines: # write all strains wr.write(line) wr.write("n") # Function to learn take a look at log utilizing context supervisor def learnFile(): rd = open ("testLog.txt", "r") # Read record of strains out = [] # record to save strains with open ("testLog.txt", "r") as rd: # Read strains in loop for line in rd: # All strains (moreover the last) will embody newline, so strip it out.append(line.strip()) return out # Main take a look at def primary(): # create testLog.txt createFile() # learn strains from testLog.txt outList = learnFile() # Iterate over the strains for line in outList: print(line.strip()) # Run Test if __name__ == "__main__": primary()
After operating the code snippet, the output is:
NumPy Theano Keras PyTorch SciPy
To be taught more about File I/O, learn our Read/ Write File In Python With Examples