Each key is separated by a colon (:) from its value, items are separated by commas, and the whole thing is enclosed in a curved brace. An empty dictionary with no items is written with only two curly braces, such as: {}.
Keys are unique within a dictionary while values cannot be. The values of a dictionary can be of any type, but the key must be of an immutable data type such as strings, numbers, or tuples.
Access value in a dictionary
To access dictionary elements, you can use square brackets familiar with the key to get its value.
Python creates a dictionary using for loop
for i in user1: # To check key in dictionary
print(i) To check all value presents in dictionary for i in user1.values(): print(i) OutPut = pramod Output = 22
value method in Python iterate dict
Python dictionary value () method The python values () method is used to gather all values from a dictionary. It takes no parameters and gives a dictionary of values. If the dictionary has no value then it returns an empty dictionary. The syntax and examples of this method are given below. signature Values () Parameter No parameters The return It gives a dictionary of values. See some examples of the values () method to understand values. working capacity.
Python dictionary value() method example -1
This is a simple example that returns all values from the dictionary. An example is given below.
# Python dictionary value () method # Creating a Dictionary Inventory = {'fan': 200, 'bulb': 150, 'LED': 1000} # Calling function Share = Inventory.values () # Displaying Results Print ("stock available", share)
Output:
Stock available {'Fan': 200, 'Bulb': 150, 'LED': 1000}
Python dictionary value () method example 2
If the dictionary is already empty, this method also returns an empty dictionary except for any errors or exceptions. See the example below.
# Python dictionary value () method # Creating a Dictionary Inventory = {} # Calling function Share = Inventory.values () # Displaying Results Print ("stock available", share)
Output:
stock available {}
Python iterate dict value() method example 3
This example uses various methods such as length, keys, etc. to get information about the dictionary.
# Python dictionary value () method # Creating a Dictionary Inventory = {'fan': 200, 'bulb': 150, 'LED': 1000} # to work length = len(inventory) Print ("Total number of values:", length) Keys = Inventory.keys () Print ("All keys:", key) Item = Inventory.items () Print ("item:", Inventory) p = Inventory.popitem () print ("Deleted Items:", p) Share = Inventory.values () Print ("stock available", share)
Output: Total number of values: 3 All Keys: Tana_Key (['Fan', 'Bulb', 'Led']) Item: {'Fan': 200, {Bulb ': 150,: Led': 1000} Deleted Items: ('LED', 1000) Stock available {'Fan': 200, 'Bulb': 150}
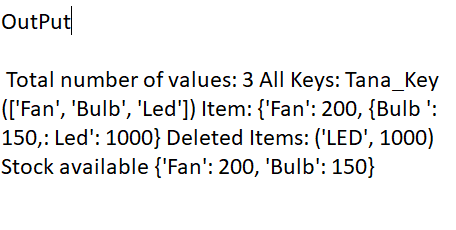
Example -2
user_info_value = user_info.values()
user_info_value = user_info.values() print(user_info_value
# OutPut = dict_values(['pramod', 22,'17691a05b9', 'B-Tech'])
example python loop through a dictionary
for i in user_info.keys(): print(user_info[i]) OutPut pramod 2217691a05b9 B-Tech OR user_info_value2 = user_info.keys() print(user_info_value2)
# OutPut = dict_keys(['name', 'age', 'rollno','class'])
Recommended Post:
- Python Hello World Program
- Python Comment | Creating a Comment | multiline comment
- Python Dictionary Introduction | Why to use dictionary
- How to do Sum or Addition in python
- Python Reverse number
- find the common number in python
- addition of number using for loop and providing user input data in python
- Python Count char in String
- Python Last Character from String
- Python Odd and Even | if the condition
- Python Greater or equal number
- Python PALINDROME NUMBER
- Python FIBONACCI SERIES
- Python Dictionary | Items method | Definition and usage
- Python Dictionary | Add data, POP, pop item, update method
- Python Dictionary | update() method