Palindrome Program in Python
In the palindrome program, we learn whether the given string is Palindrome or not.
Palindrome number is such number which if you write opposite then it will be the same as previous number. Let see one simple example of a palindrome number i.e. 121 if you write from the last digit to the first digit then still it will be the same. There will not be any changes in the number. This can be also performed in String.
There are different example of plindrome i.e. ‘dad’, ‘mom’, ‘aibohphobia’, etc.
Palindrome Number Source Code
def palindrome(name):
rev=name[::-1]
if name==rev:
return "palindrome"
return "not palindrome"
name=input("enter any string: ")
print(palindrome(name))
Output:
Input as: 12121212121
C:\Users\Pramod\PycharmProjects\pythonProject\venv\Scripts\python.exe C:/Users/Pramod/PycharmProjects/pythonProject/main.py
enter any string: 12121212121
palindrome
Process finished with exit code 0
Input as: dad
C:\Users\Pramod\PycharmProjects\pythonProject\venv\Scripts\python.exe C:/Users/Pramod/PycharmProjects/pythonProject/main.py
enter any string: dad
palindrome
Process finished with exit code 0
Input as: aibohphobia
C:\Users\Pramod\PycharmProjects\pythonProject\venv\Scripts\python.exe C:/Users/Pramod/PycharmProjects/pythonProject/main.py
enter any string: aibohphobia
palindrome
Process finished with exit code 0
Input as: Book
C:\Users\Pramod\PycharmProjects\pythonProject\venv\Scripts\python.exe C:/Users/Pramod/PycharmProjects/pythonProject/main.py
enter any string: Book
not palindrome
Process finished with exit code 0
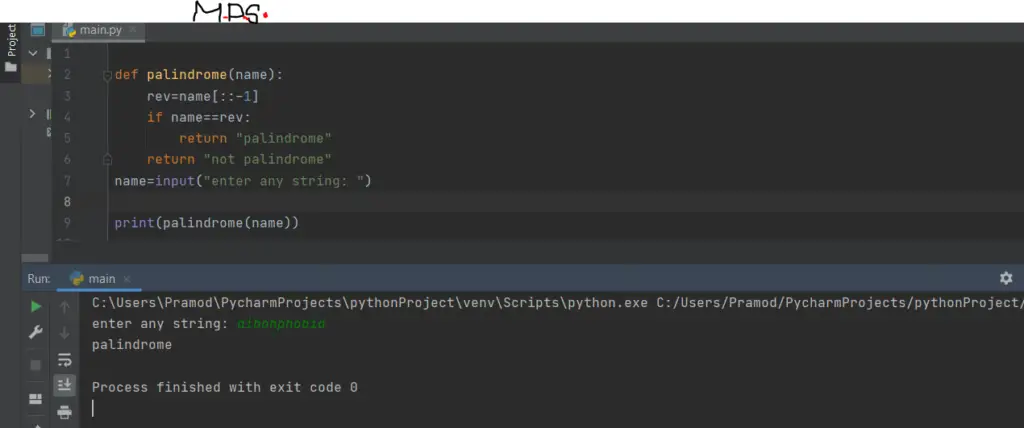
Recommended Post:
- Python Hello World Program
- Python Comment | Creating a Comment | multiline comment | example
- Python Dictionary Introduction | Why to use dictionary | with example
- How to do Sum or Addition in python
- Python Reverse number
- find the common number in python
- addition of number using for loop and providing user input data in python
- Python Count char in String
- Python Last Character from String
- Python Odd and Even | if the condition
- Python Greater or equal number
- Python PALINDROME NUMBER
- Python FIBONACCI SERIES
- Python Dictionary | Items method | Definition and usage
- Python Dictionary | Add data, POP, pop item, update method
- Python Dictionary | update() method
- Delete statement, Looping in the list In Python
- Odd and Even using Append in python
- Python | Simple Odd and Even number
Get Salesforce Answers
1 thought on “Python Palindrome Number with Example”