How to get a value from Dictionary in Python?
The value method returns a view object that displays a list of all the values in the dictionary. The python values() method is used to gather all values from a dictionary. It takes no parameters and gives a dictionary of values. If the dictionary has no value then it returns an empty dictionary. The syntax and examples of this method are given below.
Syntax:
dictionary.values()
Example: 1
user_info = {'name': 'pramod', 'age': 'unknown', 'height': 'unknown'}
user_info_value = user_info.values()
user_info_value=user_info.values()
print(user_info_value)
Output:
C:\Users\Pramod\PycharmProjects\pythonProject\venv\Scripts\python.exe C:/Users/Pramod/PycharmProjects/pythonProject/main.py
dict_values(['pramod', 'unknown', 'unknown'])
Process finished with exit code 0
Python dictionary value method Example 2
# Python dictionary value () method
# Creating a Dictionary
Inventory = {'fan': 200, 'bulb': 150, 'LED': 1000}
# Calling function
Share = Inventory.values()
# Displaying Results
print ("stock available:", Share)
Output:
C:\Users\Pramod\PycharmProjects\pythonProject\venv\Scripts\python.exe C:/Users/Pramod/PycharmProjects/pythonProject/main.py
stock available: dict_values([200, 150, 1000])
Process finished with exit code 0
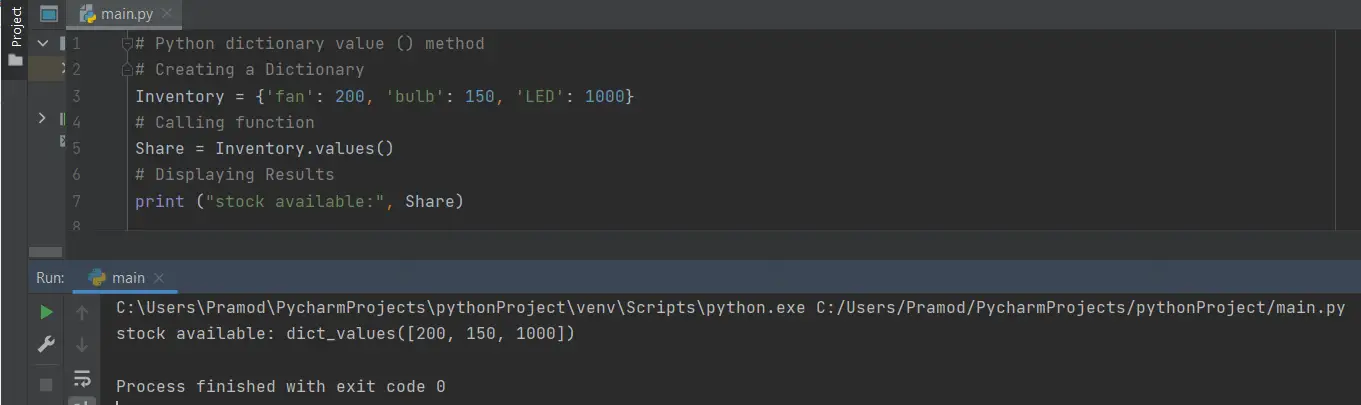
Python empty dictionary value() method
Example:
# Python empty dictionary value() method
# Creating an empty Dictionary
Inventory = {}
# Calling function
Share = Inventory.values()
# It Displaying Empty dictionary
print ("Dictionary Values:", Share)
Output:
C:\Users\Pramod\PycharmProjects\pythonProject\venv\Scripts\python.exe C:/Users/Pramod/PycharmProjects/pythonProject/main.py
Dictionary Values: dict_values([])
Process finished with exit code 0
Dictionary Operations in Python
This example uses various methods such as length, keys, etc. to get information about the dictionary.
# Python dictionary value() method
# Creating a Dictionary
Inventory = {'fan': 200, 'bulb': 150, 'LED': 1000}
# Find the length of dictionary
length = len (Inventory)
print ("Total number of values:", length)
# Display the key of dictionary
key = Inventory.keys()
print ("All keys:", key)
#Display items of dictionary
item = Inventory.items()
print ("item:", item)
#Remove or Delete the item of dictionary
p = Inventory.popitem()
print ("Deleted Items:", p)
#Display the value
share = Inventory.values()
print ("stock available", share)
Output:
C:\Users\Pramod\PycharmProjects\pythonProject\venv\Scripts\python.exe C:/Users/Pramod/PycharmProjects/pythonProject/main.py
Total number of values: 3
All keys: dict_keys(['fan', 'bulb', 'LED'])
item: dict_items([('fan', 200), ('bulb', 150), ('LED', 1000)])
Deleted Items: ('LED', 1000)
stock available dict_values([200, 150])
Process finished with exit code 0
How to Iterate Key value from Dictionary using for loop in Python
The keys() method returns a view object that displays a list of all the keys in the dictionary.
Example
user_info = {'name': 'Pramod', 'Rollno': '17691a05b9', 'Branch': 'CSE'}
for i in user_info.keys():
print(user_info[i])
user_info_value2 = user_info.keys()
print('\n',user_info_value2)
Output:
C:\Users\Pramod\PycharmProjects\pythonProject\venv\Scripts\python.exe C:/Users/Pramod/PycharmProjects/pythonProject/main.py
Pramod
17691a05b9
CSE
dict_keys(['name', 'Rollno', 'Branch'])
Process finished with exit code 0
Recommended:
- Python Hello World Program
- Python Comment | Creating a Comment | multiline comment | example
- Python Dictionary Introduction | Why to use dictionary | with example
- How to do Sum or Addition in python
- Python Reverse number
- find the common number in python
- addition of number using for loop and providing user input data in python
- Python Count char in String
- Python Last Character from String
- Python Odd and Even | if the condition
- Python Greater or equal number
- Python PALINDROME NUMBER
- Python FIBONACCI SERIES
- Python Dictionary | Items method | Definition and usage
- Python Dictionary | Add data, POP, pop item, update method
- Python Dictionary | update() method
- Delete statement, Looping in the list In Python
- Odd and Even using Append in python
- Python | Simple Odd and Even number
Get Salesforce Answers