String methods in python:
Strings modules include many useful constants and classes, therefore some deprecated inheritance functions that are also available as strings methods. In addition, Python’s built-in string classes support sequence type methods described in sequence types – str, bytes, by the array, list, tuple, category section, and string-specific methods are also described in the string-specific methods section. To output formatted strings, see the String Formatting section. Also, see the re-module for string functions based on regular expressions.
Algorithm of String Methods in Python
- step1: Start
- step2: initialise upper case letters capital = str.upper()
- step3: Initialise upper case letter small = capital.lower()
- step4: Replace str1 to str2 str2 = str1.replace(“students’,”engineer”)
- step-5: print left and right strips left = str1.strip() right = str1.strip()
- step-6: print prefixes prefix1 = str1.start with(“welcome”)
- step-7: end
String Methods in Python with Examples
str1 = "welcome to all student"
capital = str1.upper()
print(capital)
str2 = "WELCOME TO MITS"
lower = str2.lower()
print(str2)
str3 = str2.replace("MITS","madanapalle")
print(str3)
str4 = "This is an example of strip"
left = str4.strip()
print(left)
prefix1 = str1.startswith('hellow')
print(prefix1)
prefix2 = str1.startswith('wel')
print(prefix2)
prefix3 = str1.endswith('student')
print(prefix3)
Output:
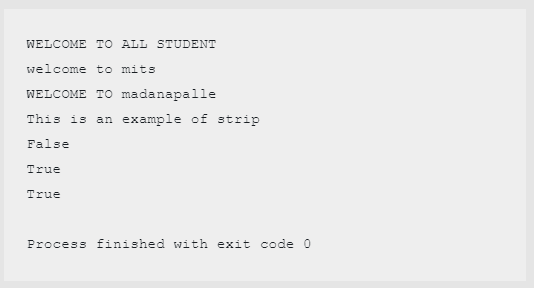
Recommended Post:
- Python Comment | Creating a Comment | multiline comment | example
- Python Dictionary Introduction | Why to use dictionary | with example
- How to do Sum or Addition in python
- Python Reverse number
- find the common number in python
- addition of number using for loop and providing user input data in python
- Python Count char in String
- Python Last Character from String
- Python Odd and Even | if the condition
- Python Greater or equal number
- Python PALINDROME NUMBER
- Python FIBONACCI SERIES
- Python Dictionary | Items method | Definition and usage
- Python Dictionary | Add data, POP, pop item, update method
- Python Dictionary | update() method
order desloratadine – cheap dapoxetine buy priligy 90mg sale