What Is Type Casting In Python With Example?
In Python,Ā Type castingĀ is a method used to change the variables/ values declared in a particular dataĀ typeĀ into another dataĀ typeĀ to match the operation required to be performed by the code snippet. InĀ python, this feature can be accomplished by using constructor functions like int(), string(), float(), char(), bool(), etc.
Type Casting Functions are:
- int()
- float()
- complex()
- bool()
- str()
int() type casting in python
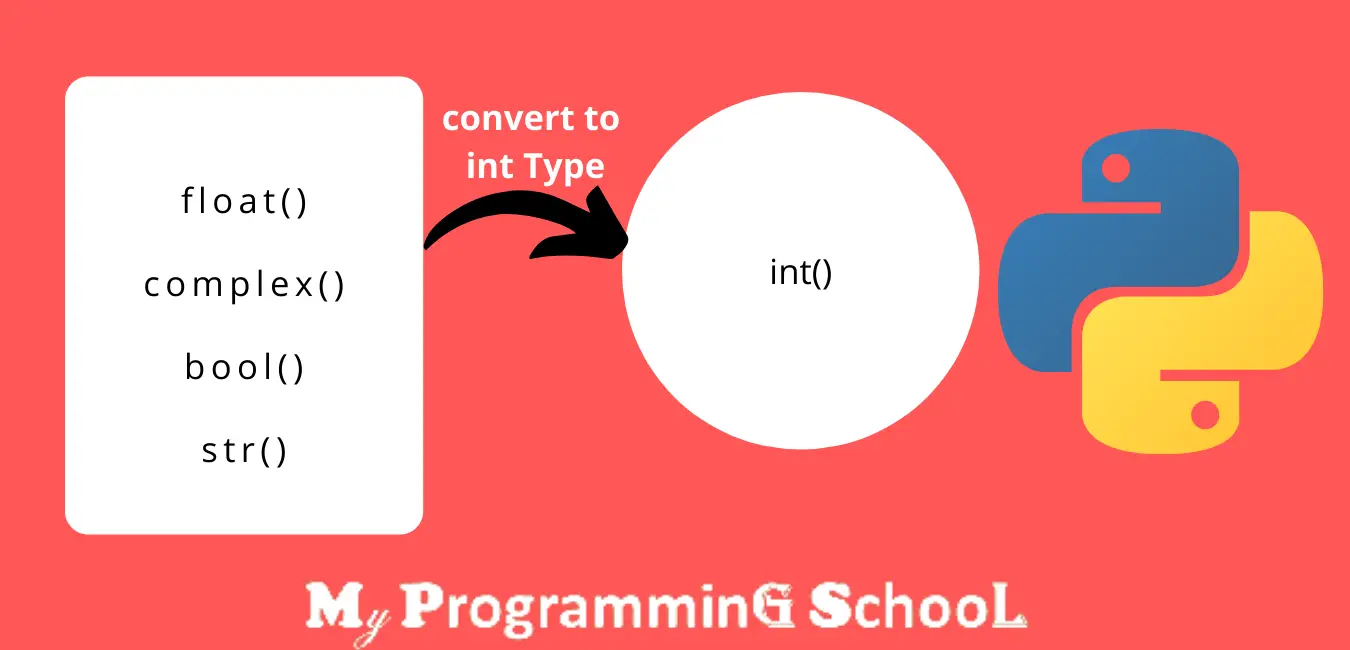
Here we will try to convert other data types into int data types for example.
- float() to int() conversion in python
Here we will learn to convert a float value into an integer value.
Syntax:
int(float_value)
Example -1:
int(10.989)Ā #output: 10
Example ā 2:
aĀ = 10.989 b = int(a) print('Type of a is: ', type(a)) print('Type of a is: ',type(b))
Output:
Type of a is:Ā <class 'float'> Type of a is:Ā <class 'int'>
- Complex number to int()/ complex ā int()
In Python, we canāt convert complex numbers into integer numbers.
Example:
int( 10 + 20j ) # Error
Output:
Traceback (most recent call last): File "<string>", line 1, in <module> TypeError: can't convert complex to int
- Bool to int Python
Convert the boolean value into an integer value. Before seeing the example we must have to know that True ā 1 and False ā 0 in Python.
Syntax:
int( boolean_value ) int( boolean_value )
Example:
print(int( True )) #Output ā 1 print(int( False )) #Output ā 0
- Convert String to int in Python
Here we will see, how we can convert string to int type.
- String internally contains the only integer value that should be specified base 10
Example:
print(int('15'))Ā #Output ā 15 print(int(0B111)) # 0B111 is binary value, 0B represented as binary & 111 is binary value. #Output ā 7 print(int('10.5')) # ValueError: invalid literal for int() with base 10: '10.5'
- float to int in python
While converting float to int type it will remove the decimal value and it will print output only integer value.
Example:
print(int(12.134)) #Output ā 12 print(int(11023.234)) #Output ā 11023
Float() Type Casting In Python
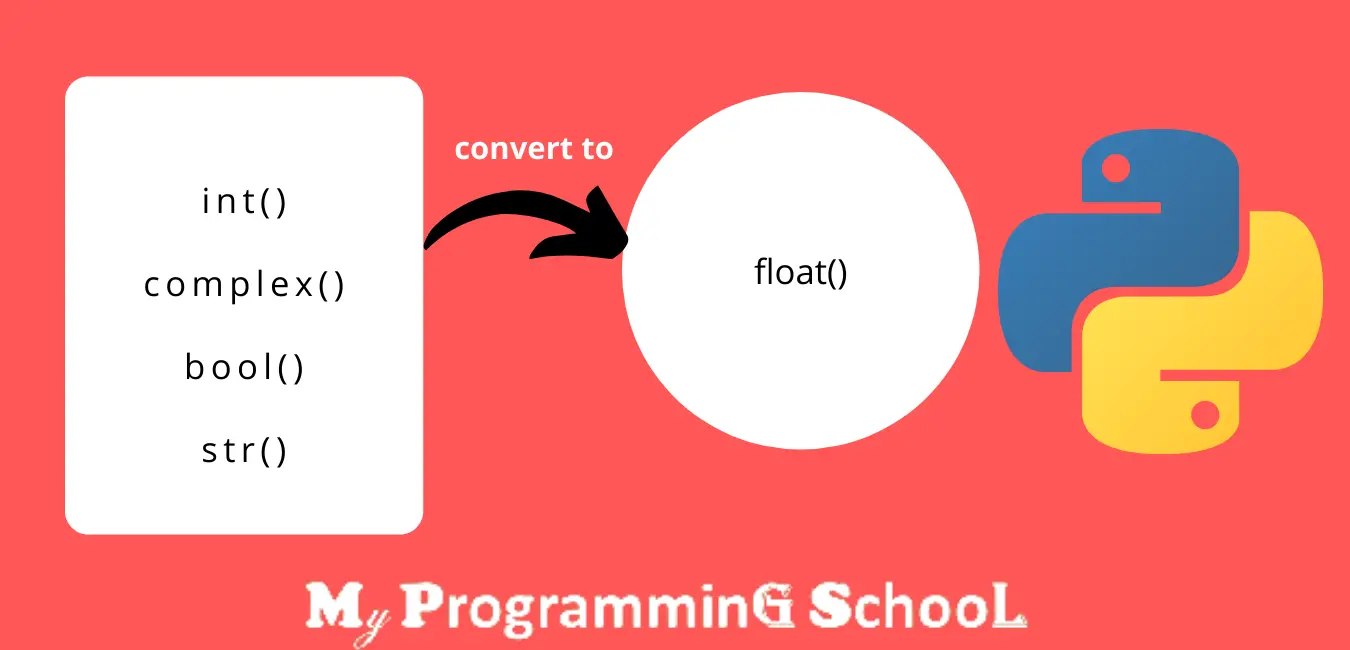
Now we will convert other data types to float types.
Syntax:
float(value)
- Int to Float in python
Here we will convert an integer value into a float value. Letās check with an example.
Syntax:
float(int_value)
Example:
print(float(15)) #Binary value to float conversion print(float(0B111)) #Hexadecimail to float conversion print(float(0XFace))
Output:
15.0 7.0 64206.0
- Complex to Float in Python
- Complex to-float conversion is not possible.
Example:
float(10 + 20j )Ā # Output ā TypeError: can't convert complex to float
- Boolean to float in Python
True and False consider as a boolean value, where True ā 1 and False ā 0
Example:
float( True ) #Output ā 1.0 float( False ) #Output ā 0.0
- convert string to float in python
To convert a string into a float, the String should contain an int value or float value with a base of 10.
Example:
float( ā10ā ) #Output ā 10.0 float ( ā10.5ā ) #Output ā 10.5 float('oXFace' ) Ā #ValueError: could not convert string to float: 'oXFace' float('A')Ā #ValueError: could not convert string to float: 'A' float('a') #ValueError: could not convert string to float: 'a'
Complex() Type Casting
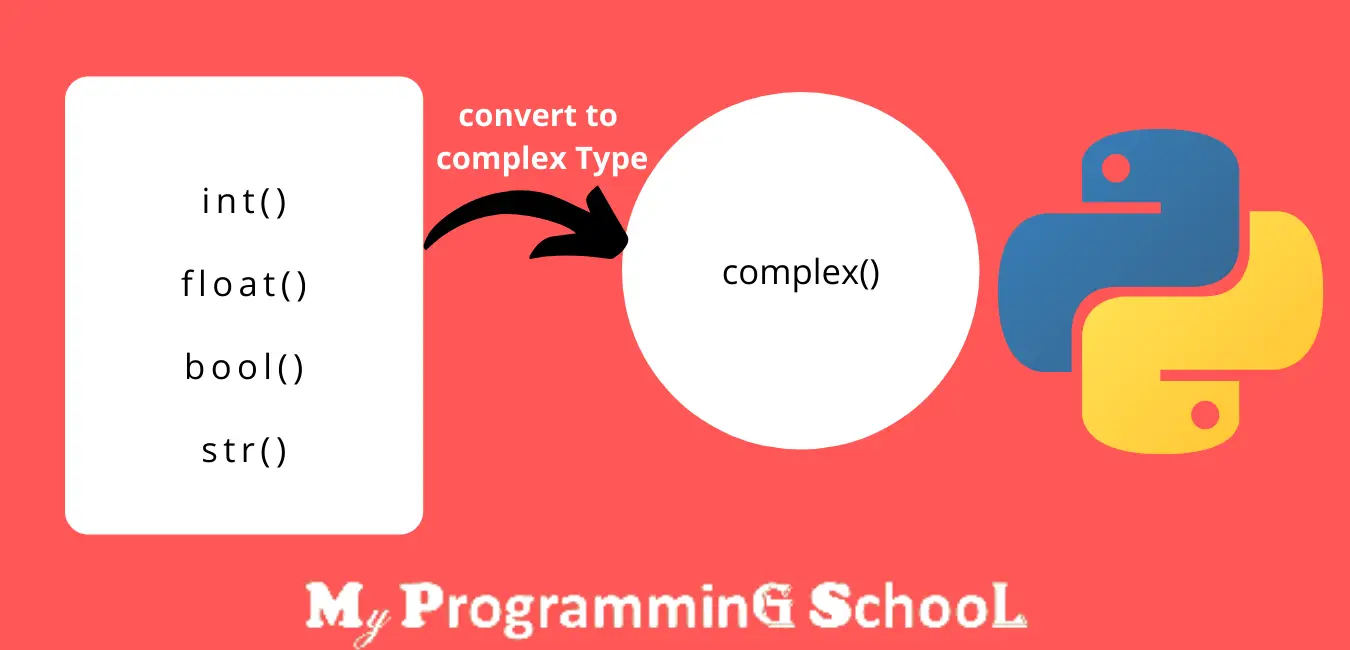
Here we will learn to convert complex number types into other data types like int to complex, float to complex, a string to complex, etc.
Syntax:
- complex(x)
- complex(x, y)
WhereĀ xĀ is aĀ real numberĀ andĀ yĀ is anĀ imaginary number
- int to complex() Python
Letās here we will learn to convert an integer number to a complex number.
Example1:
In this example, we will convert an integer number into a complex number using only a real number.
complex(10) #Outputā (10 + 0j) complex(0B1111) #Output ā (15 + 0j)
Example2:
In this example, we will convert an integer number into a complex number using both a real number & an imaginary number.
complex(10,20) #Output ā (10 + 20j) complex(0B1111, 0B1010) #Output ā (15 + 10j)
- float to complex() Python
Letās here we will learn to convert a float number to a complex number.
Example1:
In this example, we will convert a float number into a complex number using only a real number.
complex(10.5) #Outputā (10.5 + 0j)
Example2:
In this example, we will convert a float number into a complex number using both a real number & an imaginary number.
complex( 10.5, 20.6 ) #Output ā (10.5 + 20j.6)
- Boolean to complex() Python
Letās here we will learn to convert a boolean number to a complex number.
Example1:
In this example, we will convert a boolean number into a complex number using only a real number.
complex(True) #Outputā (1 + 0j) complex(False)Ā #Output ā (0 + 0j)
Example2:
In this example, we will convert an integer number into a complex number using both a real number & an imaginary number.
complex(True, 20) #Output ā (1 + 20j) complex(False, 10) #Output ā (10j) complex(10, True) #Output ā (10 + 1j) complex(10, False) #Output ā (10 + 0j)
- String to Complex()
Letās here we will learn to convert a string number to a complex number. Int and float should be based on 10.
Example1:
In this example, we will convert a string number into a complex number using only a real number.
complex(ā10ā) #Outputā (10 + 0j) complex(ā10.5ā) #Output ā (10.5 + 0j)
Example2:
In this example, we will convert a string number into a complex number using both a real number & an imaginary number.
#Both should not be a string value
complex(ā10ā, ā20ā)
#SyntaxError:Ā invalid character in an identifier
complex(ā10ā,20 )
#complex() canāt take the second arg if the first is a string
Bool() Type:
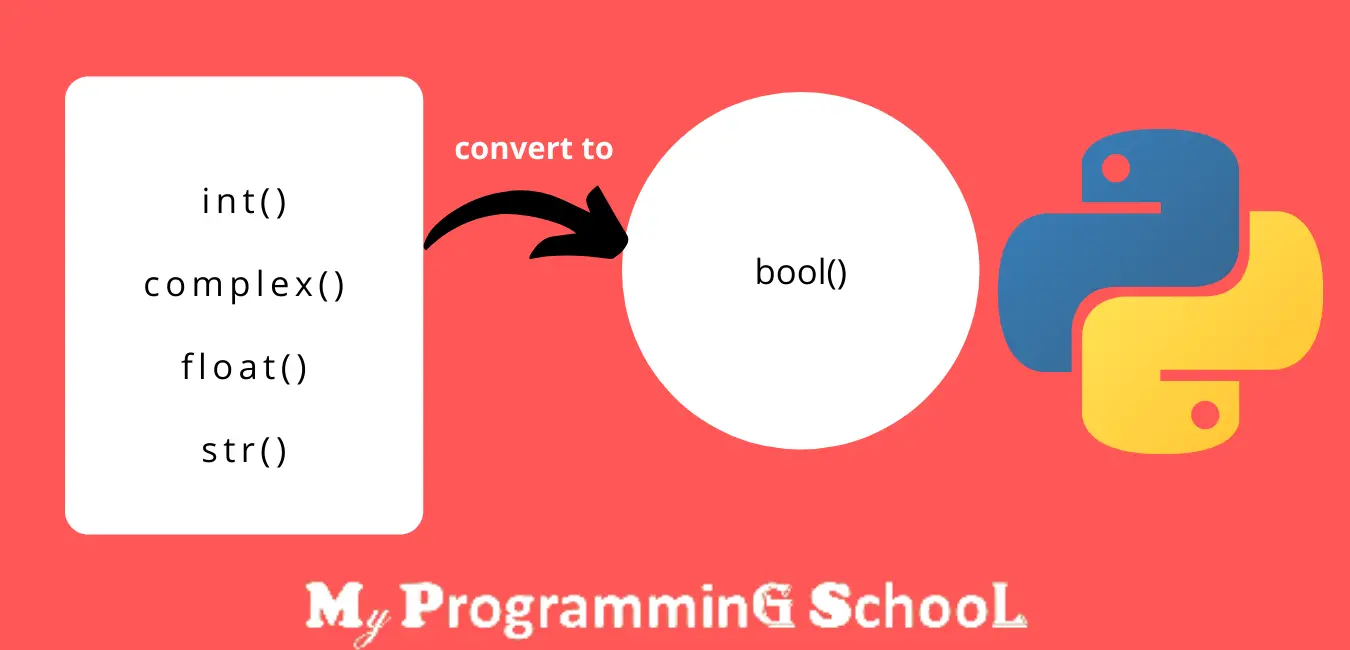
Here we will learn to convert bool number types into other data types like int to bool, float to bool, etc.
Syntax:
bool(value)
- int to bool
bool(10) #Output ā True bool(0) #Output ā False bool(-10) #Output ā True
- float to bool
bool(0.1) #Output ā True bool(0.0) #Output ā False bool(1.0) #Output ā True bool(-0.0001) #Output ā True
- Complex to bool
If both real and imaginary parts are zero ā0ā then only False else True.
Example:
bool( 0 + 0j )Ā #Output ā False bool( 1 + 0j )Ā #Output ā True
- Str to bool
If any string is available then the output is True and if a string is empty then the output is False.
Example
bool( āTrueā )Ā #Output ā True bool( āFalseā )Ā #Output ā True bool( āyesā )Ā #Output ā True bool( ānoā )Ā #Output ā True bool( ā ā )Ā #Output ā False
String Type/ Str() Type In Python
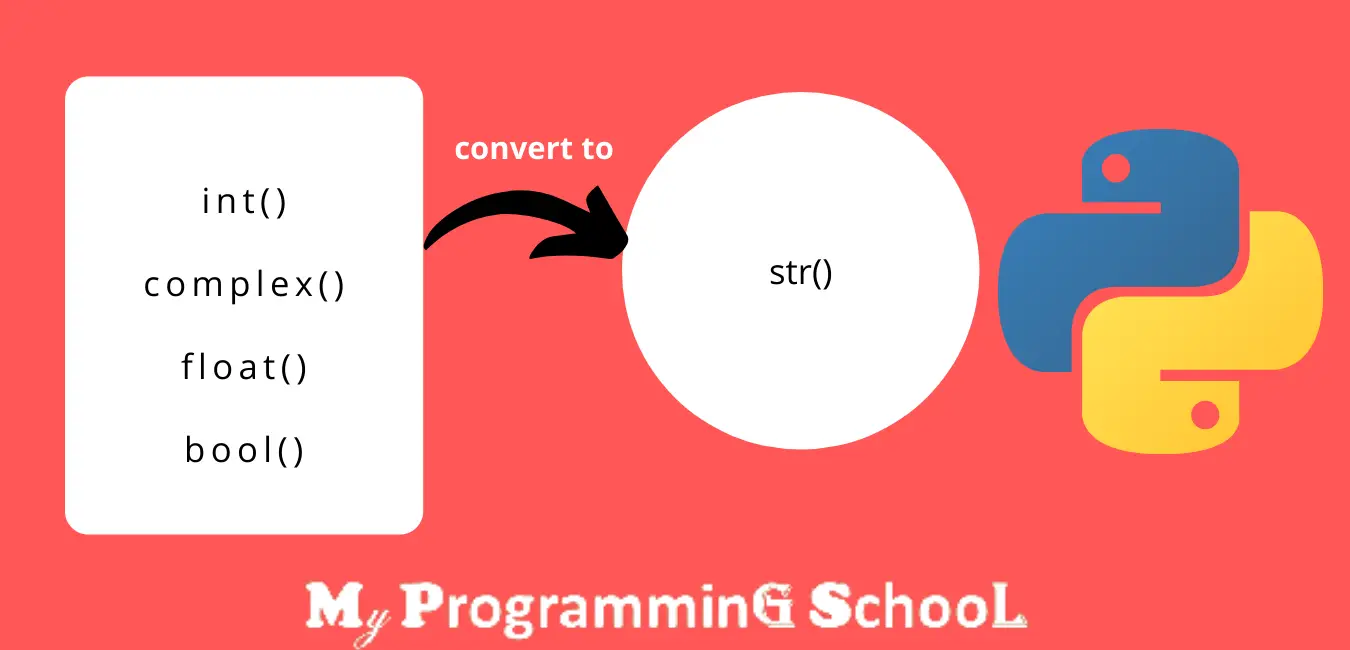
Any value can be easily converted into a string type.
Syntax:
str(value)
- int to string Type
str(10) #Output ā ā10ā #binary value to string type str(0B1111) #Output ā ā15ā
- Float to string type conversion
str(10.5) #Output ā ā10.5ā str(0.111) #Output ā ā0.111ā
- Complex to string type conversion
str(10 + 20j) #Output ā (10 + 20j) str(True + 0j) #Output ā (1+0j) str(False + 0j) #Output ā 0j str(False + 10j) #Output ā 10j str(True + 10j) #Output ā (1 + 10j)
- Bool-to-string type conversion
str(True) #Output ā āTrueā str(False) #Output ā āFalseā
Type Casting Summary
Typecasting Evaluation\Ā Arg type | Int arg | Float arg | Complex arg | Bool arg | Str arg |
int() | yes | yes | no | yes | Contain only int value with base 10 |
float() | Yes | yesĀ | no | yes | Contain int or float value with base 10 |
complex() | yes | yes | yes | yes | 1st arg. should any value but 2nd arg must be int. |
bool() | yes | yes | yes | yes | yes |
str() | yes | yes | yes | yes | yes |