In This tutorial, we will provide several techniques to compute the frequency of each character in a Python string, followed by simple examples.
Here, we have to write a program that will take an input string and count the occurrence of each character in it. We can solve this problem with different programming logic. Let’s check out each solution one by one.
Compute the Frequency of Characters in a String
It is always interesting to address a problem by taking different approaches. A real programmer keeps trying and continues thinking of doing things in a better way.
Novice approach – Use A dictionary for the frequency of chars
This may be the simplest way to count the character frequency in a Python string. You can use a dictionary, use its keys to keep the char, and the corresponding values for the no. of occurrences. It just requires to keep incrementing each value field by 1.
Source Code:
""" Using a dictionary to store the char frequency in string """ InputString = "Data Science" frequencies = for char in InputString: if char in frequencies: frequencies[char] += 1 else: frequencies[char] = 1 # Show Output print ("Per char frequency in '' is :n ".format(InputString, str(frequencies)))
Output:

Using collections.Counter() to print character frequency
Finally, you can use Python’s collection module to expose the counter() function. It computes the character frequency and returns a dictionary-like object.
Source Code:
""" Using collections.Counter() to print the char frequency in string """ from collections import Counter InputString = "Data Science" frequency_per_char = Counter(InputString) # Show Output print ("Per char frequency in '' is :n ".format(InputString, str(frequency_per_char))) print ("Type of frequency_per_char is: ", type(frequency_per_char))
Output:
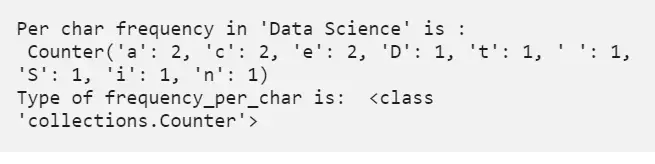
Dictionary’s get() method to compute char frequency
We have seen two approaches so far in this tutorial. However, we can further fine-tune our previous logic of using a Python dictionary and use its get() function instead. This method allows us to set the key value to zero for a new char or increment by one otherwise.
Source Code:
""" Using dict.get() to print the char frequency in string """ InputString = "Data Science" frequency_table = for char in InputString: frequency_table[char] = frequency_table.get(char, 0) + 1 # Show Output print ("Character frequency table for '' is :n ".format(InputString, str(frequency_table)))
Output:

Python set() method to compute character frequency
By using Python’s set() method, we can accomplish this assignment. Besides, we’ll need to make use of the count() function to keep track of the occurrence of each character in the string.
Source Code:
""" Using dict.get() to print the char frequency in string """ InputString = "Data Science" frequency_table = char : InputString.count(char) for char in set(InputString) # Show Output print ("Character frequency table for '' is :n ".format(InputString, str(frequency_table))) print ("Type of 'frequency_table' is: ", type(frequency_table))
Output:

Note that the output of the above program is also a dictionary object. To learn more, read our flagship Python tutorial for beginners and advanced learners.
Recommended Posts:
- MySQL Create User with Password Explained with Examples – MPS
- SQL Exercises with Sample Tables and Demo Data – MPS
- MySQL LOWERcase/LCASE() Functions with Simple Examples – MPS
- MySQL Date and Date Functions Explained with Examples – MPS
- MySQL CURRENT_TIMESTAMP() Function Explained with Examples – MPS
- Grant Privileges on a Database in MySQL with Examples – MPs
- MySQL UPSERT | Three Techniques to Perform an UPSERT – MPs
- MySQL FROM_UNIXTIME() Function Explained with Examples – MPS
- MySQL vs PostgreSQL Comparison – Know The Key Differences – MPS