A) Python Program To Find the Largest Number
In this program, we will learn to find the greatest number between three numbers. We will also see the algorithm and flowchart exercises using python.
1) Algorithm and Flowchart Exercises
First, we will see the algorithm to find the largest number and then we will see exercise
A Flowchart:
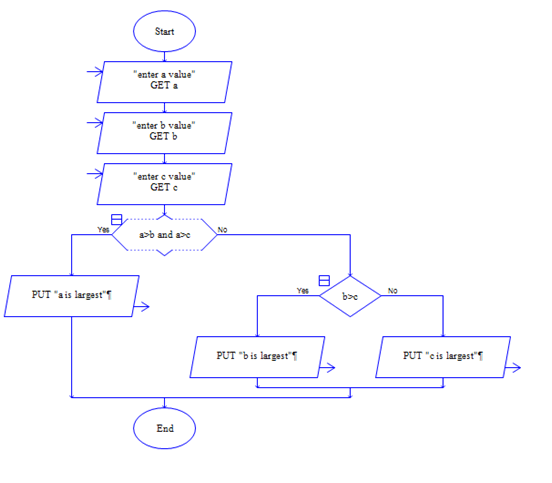
Algorithm in Python
- Step1: start
- Step2: Declare the variables a, b,c and read the inputs.
- Step3: If the condition a>b and a>c is true Print that a is largest.
- Step4: Otherwise go to the next condition b > c.
- Step5: If it is true Print that b is largest, Otherwise
- Step6: Print that c is largest,
- Step7: End
Flowchart Examples
# we can find greater numbers in different ways in python, there are different short-cut methods to find the greater and smaller number among given numbers otherwise you can take user input and then also you can. The sample code is given below.
def greater_smaller(num1, num2): if num1 > num2: return "num_1 is greater" elif num2 > num1: return "num_2 is greater" return "both are equal" num1, num2 = input("enter your num1 and num2 values in single line seperated by comma ,:").split(",") print(greater_smaller(num1, num2)) # .................................................................
Input & Output
enter your num1 and num2 values in single line seperated by comma , :23,43
num_2 is greater

B) Implement a Python script to find the biggest number between two numbers
In this program, we will see to find the biggest number between two numbers in a different way than the previous example.
2) Finding Largest Number Algorithm and Flowchart Exercises
First, we will also see the algorithm to find the largest number and then we will see example.
Algorithms for beginners
- Step1: Start
- Step2; Declare the variables a & b and read a,b.
- Step3: check condition a>b
- Step4: If the condition is true then
- Step5: Print that a is largest
- Step6: Else
- Step7: Print that b is the largest
- Step: End
Source Code:
a=int(input("enter a:"))
b=int(input("enter b:"))
if (a>b):
print("a is largest")
elif (a<b):
print("b is largest")
else:
print("a and b are equal")
Output:
>>>
enter a:45
enter b:54
b is largest
>>>

Recommended Posts:
- How to Become a Machine Learning Engineers – easy Step by Step Guide
- Python Difference Between Two Lists using Python Set and Without Set
- How to Open file in python|Read File Line by Line with Examples
- Python String Find() Method Explained with Examples
- How to Generate Random Numbers in Python with Examples
Get Salesforce Answers