Develop a flowchart for swapping two values using functions
In this program, we will learn to swap two numbers using functions.
Algorithm:
- Step1: Start
- Step2: Declare the variables a, b, and read the inputs.
- Step3: Call the function swap(a,b)
- Step4: The function performs the following steps.
- t=a
- a=b
- b=t
- Step5: print Swapped numbers are: a b.
- Step6: End.
Flowchart to swap two numbers
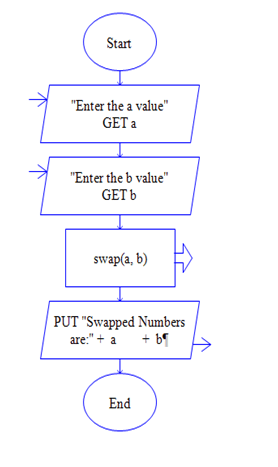
flow chart for swapping values
Python program to swap two numbers in a list
// Take two integer input value a = int ( input ( " enter your number a : ")) b = int ( input ( " enter your number b : ")) # n2 = eval ( input ( " enter your second number : ")) # in this you can take any types of user input n1 = a a = b b = n1 print (" After swap the number b is : ",b) print (" After swap the number a is : ",a)
Input and Output
enter your number a : 100
enter your number b : 200
After swap the number b is : 100
After swap the number a is : 200
How do you swap two numbers without using a temporary variable in Python?
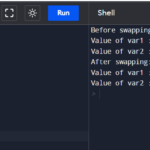
To switch two variables first we have to declare two variables.
Example
var1 = 5
var2 = 7
print (“Before swapping: “)
print(“Value of var1 : “, var1, “\nValue of var2 : “, var2)
code to swap ‘var1’ and ‘var2’
var1, var2 = var2, var1
print (“After swapping: “)
print(“Value of var1 : “, var1, “\nValue of var2 : “, var2)
Output:
Before swapping:
Value of var1 : 5
Value of var2 : 7
After swapping:
Value of var1 : 7
Value of var2 : 5
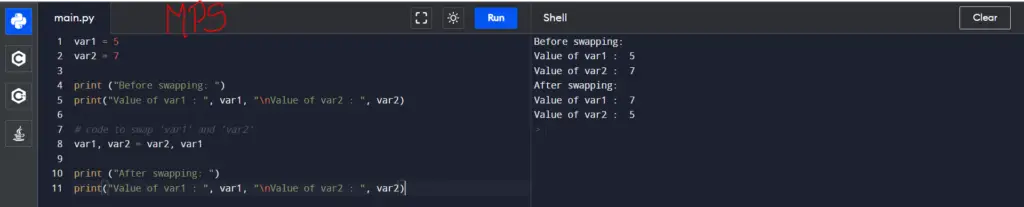
How do you switch two variables in Python?
To swap values in python, we have to declare two variables and one temporary variable. Example
temp = a
a = b
b = temp
Recommended Posts:
- How to Become a Machine Learning Engineers – easy Step by Step Guide
- Python Difference Between Two Lists using Python Set and Without Set
- How to Open file in python|Read File Line by Line with Examples
- Python String Find() Method Explained with Examples
- How to Generate Random Numbers in Python with Examples
Get Salesforce Answers