Flowchart to sort list of numbers python
In this program, we will learn to draw a flowchart for sorting array elements.
Algorithm:
- Step1: Start
- Step 2: Enter the array size and read as n.
- Step 3: Enter the array elements and read as [i].
- Step 4: Print the array elements before sorting.
- Step5: After sorting, print the statement array elements.
- Step6: Take 2 nested loops, take i variable in 1 loop, and j variable in 1 loop.
- Step7: Check condition i> n in the I loop.
- Step8: If the condition is false, go to the j loop. Go to the other end.
- Step9: In j loop, check condition [j]> [a + j + 1].
- Step10: If the condition is true, swap one [j] and one [j + 1]. Otherwise, go to the end.
- Step11: Print the array elements [i].
- Step 12: End
Flow Chart Diagram
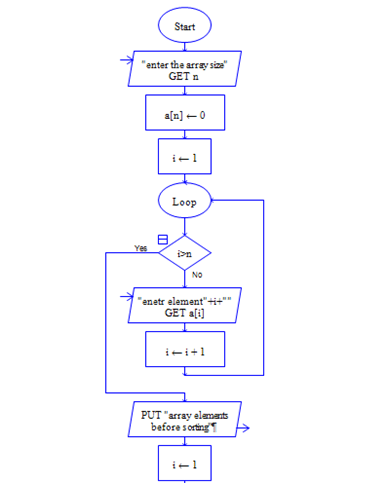
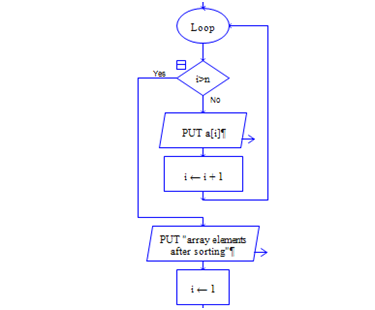


Source Code:
Sort the list of number in Ascending Order
# Sort the list of integer number number = [11,23,32,43,21,45,63,76,4] # sort the list in assending order number.sort() print('list assending:',number)
Sort the list of number in Descending Order
number = [11,23,32,43,21,45,63,76,4] # sort the list of number in decending order number.sort(reverse = True) print('List decending:',number)
Sort the list in single line of code
number = [11,23,32,43,21,45,63,76,4] # sort the list of number in single line print('Sorted list:',sorted(number))
Sort the list using key
def sorting(numbers): return numbers[1] list_tupl = [(1,3), (4,2), (7,1), (9,7)] # sort the list of tuple in assending order list_tupl.sort(key = sorting) # sort the list of tuple in decending order list_tupl.sort(reverse = True) print('Set of tuple decending',list_tupl)
Related Posts:
- Understand C Variables with Flowcharts and Examples
- Flowchart to find largest element in an array in python
- Flowchart to sort the list of numbers in python
- Flowchart for swapping two values using functions in Python
- Flowchart for finding biggest number in “python”
- Flowchart to display the reverse of a number in python
Get Salesforce Answers