C Variables with Flowcharts and Examples
In this C programming class, we’ll explain the thoughts of C variables using flowcharts and models. It is one of many center building blocks in C. Subsequently, kindly learn and see with full core interest.
1. What are Variables in C Programming?
In arithmetic, a variable suggests that its value can change and fixed infers that its value can not change.
In C, variables are particular names identified with values. They fill in as a holder and factor to a specific area in this framework memory.
We can enter a C variable in a split second by its title and moreover using the memory bargain relegated to it.
2. C Variable Naming Rules
C variables are case-sensitive names. Here are a number of easy naming conventions for them.
- A-C variable title can embody one or extra of the next:
- Letters (In each capital and small case)
- Digits from 0 – 9
- Underscore (No different particular characters)
- It should both have an underscore or an alphabet as the primary character.
- No restriction on the title size however 31 is the max appropriate for a lot of the C compilers.
Check under a number of examples of legitimate and invalid C variable names.
int _count = 5; // legitimate integer variable int fifth = 5; // error => variable title cannot start with a digit. char selection = '0'; // legitimate variable title int selection = 0; // error => cannot have one other variable with the identical title.
3. C Variables Types
In C programming, variables are of two sorts:
- Local,
- Global
3.1. Local Variables in C
Nearby C variables have a confined degree inside its code block portrayed with the wavy supports. Their position should happen initially.
The C compiler doesn’t appoint a default worth to the local variables. Thus, we should introduce a cost sooner than using them.
The lifetime of a C local variable starts as the execution goes into the code block which is its degree of beginning and finishes in the wake of leaving from the indistinguishable.
In any case, sooner than concentrating extra on variables, we should cowl a few basics of data sorts.
3.2. Global Variables in C
A variable that has a placement in the header part of a C file classifies as global.
The compiler initializes all Global C variables by default as per the next guidelines.
- An integer variable with 0 as the default worth
- The char sort with ‘.’
- A float sort once more with 0 as the default
- A pointer with NULL
It stays lively and accessible all through the execution till it ends.
4. C Variables Data Types
Data sorts allow programmers to outline variables that may maintain the worth required by this system or the enterprise logic.
Let’s assume that we’ll solely want the next three commonplace C information sorts for this class.
4.1. int
The int represents the integer information sort. It can comprise optimistic, unfavorable numbers, and zero. Any different information included in this will give an error.
4.2. float
The float key phrase is to outlines variables that may maintain decimal numbers.
4.3. double
The double is one other information sort just like the float. The variables of this sort may also maintain decimal numbers however present higher accuracy and precision.
That is all we have to study at this second. We will see information sorts from the depth in the upcoming lessons.
Now, we’ll create a sample right here for all applications hereafter. We will see one instance in three alternative ways.
5. Workflow of A C Program
We can perceive the workflow of any C program utilizing the flowcharts. Let’s know what are they and how can they assist.
5.1. What is the aim of Flowcharts?
It is only a visible illustration of the functioning of a program created utilizing a pre-outlined set of shapes or symbols.
5.2. Elements in a Flowcharts
The following desk ought to enable you to in understanding them.
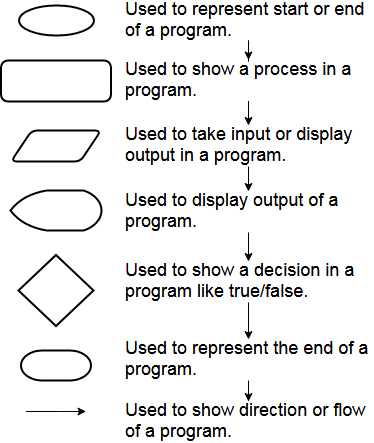
These are just a few fundamental shapes however sufficient to get us began.
Let’s study a number of important phrases.
- Algorithm: An algorithm is a quick systematic clarification of a program. It simply explains the working of a program roughly, not the precise code.
- Pseudo Code: It is the code written in a human-readable language such as English.
6. Variables Demo in C
Let us begin with an easy program to multiply two numbers.
6.1. Example – C Program to Multiply Two Numbers
6.1.1. Flowcharts & Pseudo Code
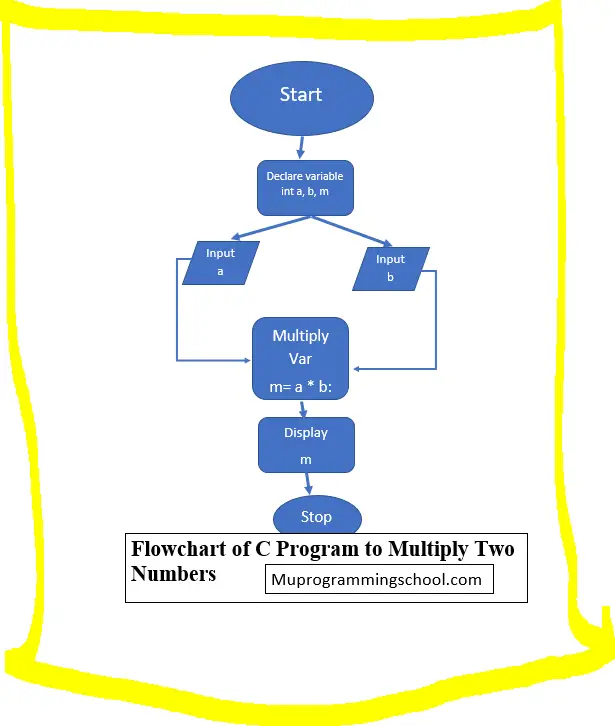
Algorithm:
- Step 1: Start.
- Step 2: Take enter from the person for multiplying two numbers.
- Step 3: Multiply two numbers (m=a*b)
- Step 4: Display output (m)
- Step 5: Stop
6.1.2. C Coding Snippet
Note: Now there are some key phrases, which we’ll see after this code.
#include<stdio.h>
#include<conio.h>
void main()
{
int a, b, m;
printf("Enter two numbers: ");
scanf("%d,%d",&a,&b);
m= a*b;
printf("The multiplication of %d and %d is %d",a,b,m);
getch();
}
6.1.3. Step by Step Program Summary
- #include<conio.h>: This header record advises the pc to consolidate console enter/output abilities. Consequently, conio represents console enter/yield.
- Void main(): It is the primary presentation in a C program as referenced in the sooner exercises.
- Note: You have seen us using the int sort with the essential. The essential differentiation here is that void makes no difference to return while the number sort requires the entertainer to return a numeric worth.
- scanf(): Used to take enter from an individual. The %d signal is for showing that the data sort is a number. We will concentrate extra about this in extra sections. The ampersand (&) signal advises the pc that it should retailer the value of the taken enter in that specific arrangement with. E.g., &a means that the value of the essential enter will get saved in the circumstance of a.
- getchar(): It advises this framework to take care of the reaction of the individual. It means “Get Character.” The PC holds the yield shown for the individual to comprehend the yield.
Output:
Now we’ll see a thrilling instance of how variables work.
6.2. Example – C Program to Swap Two Integers
6.2.1. Flowcharts & Pseudo Code
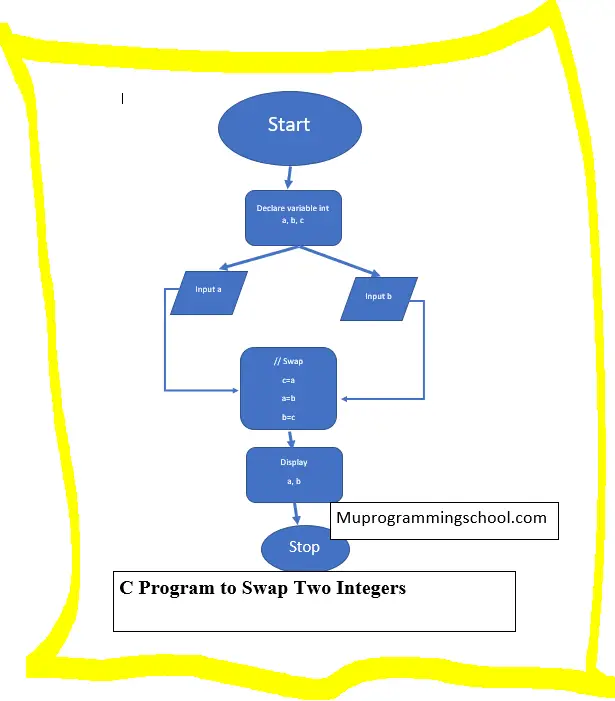
Algorithm:
- Step 1: Start
- Step 2: Initialize two enter variables (a, b) and one for swapping(c).
- Step 3: Accept enter variables from the person (a & b)
- Step 4: Swap the 2 variables
- # Swap variables c=a, a=b, b=c
- Step 5: Display the values earlier than and after swapping.
- Step 6: Stop.
6.2.2. C Coding Snippet
#include<stdio.h>
#include<conio.h>
void main()
{
int a,b,c;
printf("Enter two numbers to swap : ");
scanf("%d %d",&a,&b);
printf("Values before swapping: %d %d",a,b);
c=a;
a=b;
b=c;
printf("\nValues after swapping: %d %d",a,b);
getch();
}
The output ought to come one thing like this-
You might have realized that we used the 3rd variable for swapping the values of the 2 inputs. Can you write a program that may do this without utilizing the third variable? If you’ll be able to do this, you’ll actually get the thought of how variables work. If not, do not fear, one other instance is accessible subsequent.
We would advocate that you just try very laborious on this. It is simple.
7. Programming Exercise on C Variables
Write a program to swap values of two variables without utilizing a 3rd variable.
Solution:
#include<stdio.h>
#include<conio.h>
void main()
{
int a,b;
printf("Enter two numbers to swap : ");
scanf("%d %d",&a,&b);
printf("Values before swapping: %d %d",a,b);
a = a+b;
b = a-b;
a = a-b;
printf("\nValues after swapping: %d %d",a,b);
getch();
}
The output would be identical to the sooner program.
NOTE: We have simply lined the fundamentals of variables right here. As variables are unlimited, we’ll see them in bits and items included in different chapters.
Related Posts
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Source link