How to Create Dictionary in Python with Example
In this program, we will learn to create a dictionary, delete the dictionary, insert a value into the dictionary, and display value from a dictionary.
In Python a dictionary can be created by placing a sequence of elements within curly {} braces, separated by a ‘comma’, The dictionary consists of a pair of values, a key, and a corresponding pair element with its key: value
python dictionary operation is like insert, delete, display, etc
learn more about Python Dictionary:
- Merge Python Dictionary using Multiple Methods
- Python Iterate Dict and Python Loop Through Dictionary Example
- fromkeys method, copy method, clear method, get method in dictionary
- Python Dictionary Update method
- Python dictionary add data, pop, popitem, update method
- Python Dictionary Items method Definition and usage
Algorithm to create dictionary in Python
- Step1 Start
- Step2 Create dictionary and assign to stud
- Step3 insert 1,2,3, as name,age,sub
- Step4 Display stud dictionary
- Step5 Insert
- Step6 Insert 4 as add and again display dictionary
- Step7 Delete 1,3 from dictionary and display
Source Code:
#Create dictionary stud = {} print(stud) # insert operation in dictionary stud['1']='name' stud['2']='age' stud['3']='sub' # Display operation in dictionary print(stud) stud['4']='add' print(stud) # Delete operation in a dictionary using del del stud['1'] print(stud) del stud['3'] print(stud)
Output:
{}
{'1': 'name', '2': 'age', '3': 'sub'}
{'1': 'name', '2': 'age', '3': 'sub', '4': 'add'}
{'2': 'age', '3': 'sub', '4': 'add'}
{'2': 'age', '4': 'add'}
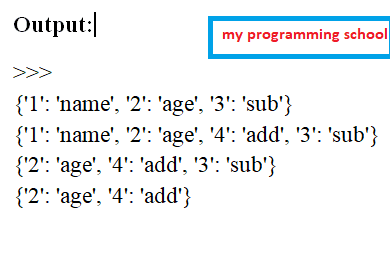
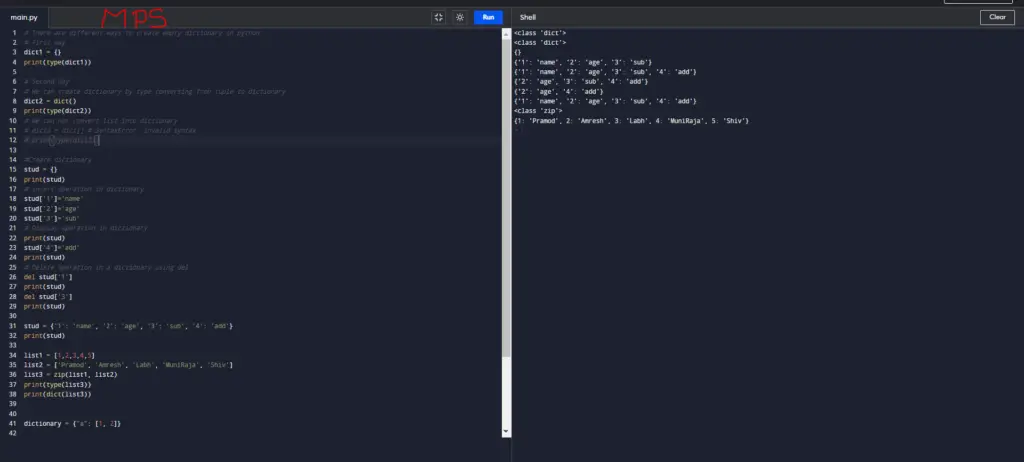
FAQ:
How do you create an empty dictionary?
# There are different ways to create empty dictionary in python
# First way
dict1 = {}
print(type(dict1)) # <class ‘dict’>
# Second way
# We can create dictionary by type conversing fron tuple to dictionary
dict2 = dict()
print(type(dict2)) # <class ‘dict’>
# We can not convert list into dictionary
# dict3 = dict[] # SyntaxError: invalid syntax
# print(type(dict3))
How do I create a static dictionary in Python?
In the python dictionary program, a pair of key and value are closed by Curly brackets {}. key:value represents a single element of dictionary in python. Example:
stud = {‘1’: ‘name’, ‘2’: ‘age’, ‘3’: ‘sub’, ‘4’: ‘add’}
print(stud)
How do you declare a dictionary in Python 3?
To declare a dictionary in python 3, just we have to use the curly brackets {}. Example:
variable = {} or we can declare by converting tuple in dictionary
variable = dict()
How do you make a list into a dictionary?
To make a list into a dictionary, we have to need two lists and we have to zip function to combine both list,s and then we have to do type conversion.
Example:
list1 = [1,2,3,4,5]
list2 = [‘Pramod’, ‘Amresh’, ‘Labh’, ‘MuniRaja’, ‘Shiv’]
list3 = zip(list1, list2)
print(type(list3)) # <class ‘zip’>
print(dict(list3)) #{1: ‘Pramod’, 2: ‘Amresh’, 3: ‘Labh’, 4: ‘MuniRaja’, 5: ‘Shiv’}
How do you create a dictionary of a list?
To create a dictionary of a list, use dict.update() to add elements with key and value in dictionary.
dictionary = {“a”: [1, 2]}
dictionary.update({“b”: [3, 4]}) #Merge two dictionaries
print(dictionary)
Output: {‘a’: [1, 2], ‘b’: [3, 4]}
Recommended Posts:
- How to Create Forgot System Password with PHP & MySQL
- Datatables Editable Add Delete with Ajax, PHP & MySQL
- Download Login and Registration form in PHP & MySQL
- Export Data to Excel in Php Code
- Hospital Database Management System with PHP MySQL
Get Salesforce Answers