Set Operation in java
In this program, we will write a program for set operations in java.
The set is an interface that extends Collection. It is an unordered collection of objects in which duplicate values cannot be stored. Basically, Set is implemented by Hash Set, LinkedHashSet, or TreeSet (sorted representation). Set has various methods to add, remove clear, size, etc to enhance the usage of this interface
Procedure of Set Operations in Java:
- Set is an interface that extends Collection. It is an unordered collection of objects in which duplicate values cannot be stored.
- Basically, Set is implemented by HashSet, LinkedHashSet, or TreeSet (sorted representation).
- Set has various methods to add, remove clear, size, etc to enhance the usage of this interface.
Set Operations Examples
// Java code for adding elements in Set importjava.util.*; publicclassSet_example { publicstaticvoidmain(String[] args) { // Set deonstration using HashSet Set<String>hash_Set = newHashSet<String>(); hash_Set.add("Hello"); hash_Set.add("For"); hash_Set.add("Hello"); hash_Set.add("Example"); hash_Set.add("Set"); System.out.print("Set output without the duplicates"); System.out.println(hash_Set); // Set deonstration using TreeSet System.out.print("Sorted Set after passing into TreeSet"); Set<String>tree_Set = newTreeSet<String>(hash_Set); System.out.println(tree_Set); } }
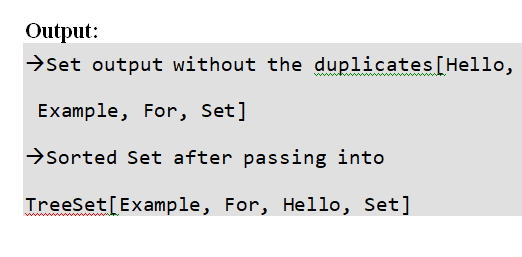
Recommended Posts:
- How to Create Forgot System Password with PHP & MySQL
- Datatables Editable Add Delete with Ajax, PHP & MySQL
- Download Login and Registration form in PHP & MySQL
- Export Data to Excel in Php Code
- Hospital Database Management System with PHP MySQL
Get Salesforce Answers