List Interface in Java with Examples
Aim:
To write a java program for creating a list.
The Java.util. The list is a child interface of collection. It is an ordered collection of objects in which duplicate values can be stored. Since List preserves the insertion order, it allows positional access and insertion of elements.
Algorithm for Java List:
- List Interface is the subinterface of Collection.
- It contains index-based methods to insert and delete elements.
- It is a factory of ListIterator interface.
- List<String> al = new ArrayList<String>();
- al.add(“Amit”);
- al.add(“Vijay”);
- al.add(“Kumar”);
- al.add(1,”Sachin”);
- ListIterator<String> itr=al.listIterator();
Source Code:
// Java program to demonstrate positional access
// operations on List interface
import java.util.*;
public class ListDemo
{
public static void main (String[] args)
{
// Creating a list
List<Integer> l1 = new ArrayList<Integer>();
l1.add(0, 1); // adds 1 at 0 index
l1.add(1, 2); // adds 2 at 1 index
System.out.println(l1); // [1, 2]
// Creating another list
List<Integer> l2 = new ArrayList<Integer>();
l2.add(1);
l2.add(2);
l2.add(3);
// Will add list l2 from 1 index
l1.addAll(1, l2);
System.out.println(l1);
// Removes element from index 1
l1.remove(1);
System.out.println(l1); // [1, 2, 3, 2]
// Prints element at index 3
System.out.println(l1.get(3));
// Replace 0th element with 5
l1.set(0, 5);
System.out.println(l1);
}
}
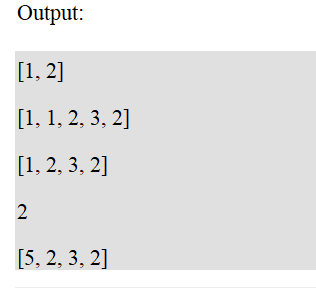
FAQ:
How do you declare a list in Java?
In java, we can declare a list by following way:
List<Integer> l1 = new ArrayList<Integer>();
What are the list methods in Java?
The list methods in java are:
int size()
boolean isEmpty()
boolean contains(Object o)
iterator iterator()
object[] toArray()
boolean add(E e)
boolean remove(Object o)
boolean retainAll(Collection c)
void clear()
E get(int index)
How do I search a list in Java?
To search object from the list in java using the method contains(). It will return True when the object is available in a list if not available then it will return False.
Is ArrayList same as list Java?
ArrayList is a Class. ArrayList is present in java.util package. It provides us with a dynamic array. The size of ArrayList is increased automatically if the size of the list increase or the list removes.
The list is as Interface. List interface extends the size of the frame. The list can not be instantiated. List interface creates a group of elements that store the sequence of the element.
Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Write a java program to Checks whether a given string is a palindrome or not
- Write a java program to perform multiplication of two matrices
- write a java program that prints the Fibonacci series for a given number.
- Write a Java program that finds the factorial of a number
- Write a Java program that finds prime numbers between 1 to n
- Write a Java program that prints all real and imaginary solutions to the quadratic equation
- Odd and Even number in java | Algorithm
- Even number in java | algorithm with source code
- Java greater number using a loop
- Java Area of Rectangle
- What is a polygon? | Area of Triangle in java
- To calculate the Area of Circle in the java program
- Java Addition through user input
- Addition program in java with source code
Find the solution to the salesforce Question and many more