Write a java program establish a Java Database Connectivity (JDBC)
Aim:
To write a java program establish a JDBC connection, create a table student with properties name, register number, mark1,mark2, mark3. Insert the values into the table by using java.
What is JDBC explain?
Java Database Connectivity (JDBC) is an application program interface (API) specification for connecting programs written in Java to the data in popular databases. The application program interface lets you encode access request statements in Structured Query Language (SQL) that are then passed to the program that manages the database.
It returns the results through a similar interface. JDBC is very similar to the SQL Access Group’s Open Database Connectivity (ODBC) and, with a small “bridge” program, you can use the JDBC interface to access databases through the ODBC interface. For example, you could write a program designed to access many popular database products on a number of operating system platforms.
When accessing a database on a PC running Microsoft’s Windows 2000 and, for example, a Microsoft Access database, your program with JDBC statements would be able to access the Microsoft Access database.JDBC actually has two levels of interface. In addition to the main interface, there is also an API from a JDBC “manager” that in turn communicates with individual database product “drivers,” the JDBC-ODBC bridge if necessary, and a JDBC network driver when the Java program is running in a network environment (that is, accessing a remote database).
When accessing a remote database, JDBC takes advantage of the Internet’s file addressing scheme and a file name looks much like a Web page address (or Uniform Resource Locator). For example, a Java SQL statement might identify the database as:
JDBC Example Program in Java
import java.sql.*;
public class JDBCExample {
// JDBC driver name and database URL
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/STUDENTS";
// Database credentials
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
//STEP 2: Register JDBC driver
Class.forName("com.mysql.jdbc.Driver");
//STEP 3: Open a connection
System.out.println("Connecting to a selected database...");
conn = DriverManager.getConnection(DB_URL, USER, PASS);
System.out.println("Connected database successfully...");
//STEP 4: Execute a query
System.out.println("Creating table in given database...");
stmt = conn.createStatement();
String sql = "CREATE TABLE STUDENT " +
"(RollNo INTEGER, " +
" Name VARCHAR(255), " +
" Marks1 INTEGER " +
" Marks2 INTEGER, " +
" Marks3 INTEGER, ")";
stmt.executeUpdate(sql);
System.out.println("Created table in given database...");
String sql1="INSERT INTO STUDENTS (RollNo,Name,Marks1,Marks2,Marks3) values (1,"a",21,23,34)";
stmt.executeUpdate(sql1);
System.out.println("data inserted into table");
}catch(SQLException se){
//Handle errors for JDBC
se.printStackTrace();
}catch(Exception e){
//Handle errors for Class.forName
e.printStackTrace();
}finally{
//finally block used to close resources
try{
if(stmt!=null)
conn.close();
}catch(SQLException se){
}// do nothing
try{
if(conn!=null)
conn.close();
}catch(SQLException se){
se.printStackTrace();
}//end finally try
}//end try
System.out.println("Goodbye!");
}//end main
}//end JDBCExample
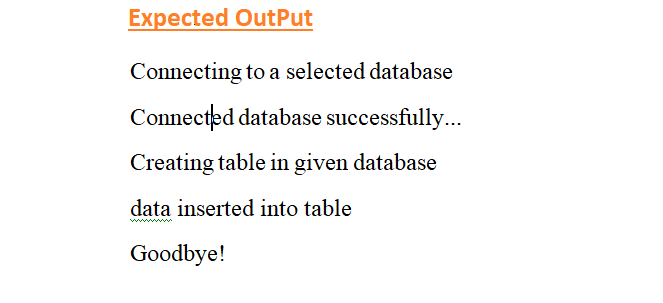
FAQ:
What are the 4 types of JDBC drivers?
#1: JDBC-ODBE
#2: Native API
#3: Net Protocol Java Driver
#4: Native Protocol Java Driver
What are the steps in JDBC connection?
In the following way the JDBC Connections occurs:
//STEP 1: Register JDBC driver
Class.forName(“com.mysql.jdbc.Driver”);
//STEP 2: Open a connection
conn = DriverManager.getConnection(DB_URL, USER, PASS);
What is JDBC in Java with example?
JDBC is an application program interface (API) specification for connecting programs written in Java to the data in popular databases. The application program interface lets you encode access request statements in SQL that are then passed to the program that manages the database.
Example: You can check the above mention.
What is ODBC and JDBC?
JDBC (Java Database Connectivity) is an application program interface (API) specification for connecting programs written in Java to the data in popular databases. Open Database Connectivity (ODBC) is an SQL-based Application Programming Interface (API) created by Microsoft that is used by Windows software applications to access databases via SQL.
What is JDBC Connection class?
The Java Database Connectivity (JDBC) Connection class, java.sql.Connection, represents a database connection to a relational database. Before reading or write data from and to a database via JDBC, you just need to open a connection to the database.
Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Write a java program to Checks whether a given string is a palindrome or not
- Write a java program to perform multiplication of two matrices
- write a java program that prints the Fibonacci series for a given number.
- Write a Java program that finds the factorial of a number
- Write a Java program that finds prime numbers between 1 to n
- Write a Java program that prints all real and imaginary solutions to the quadratic equation
- Odd and Even number in java | Algorithm
- Even number in java | algorithm with source code
- Java greater number using a loop
- Java Area of Rectangle
- What is a polygon? | Area of Triangle in java
- To calculate the Area of Circle in the java program
- Java Addition through user input
- Addition program in java with source code
Find the solution to the salesforce Question and many more