Java program that reads a file and displays the file on the screen with a line number before each line
Aim:
To write a Java program that reads a file and displays the file on the screen with a line number before each line.
Algorithm:
- import java package
- Create class Linenum
- Create main function
- public static void main( String[] args)throws IOException
- FileInputStream fil;
- LineNumberInputStream line;
- int i;
- Create try block
- fil = new FileInputStream(args[0]);
- line = new LineNumberInputStream( file );
- Catch( FileNotFoundException e )
- Print No such file found
- Inside do
- read line i = line.read();
- Use if condition
- if( i == ‘n’)
- System.out.print(line.getLineNumber()+” “);
- Else: System.out.print((char)i);
- if( i == ‘n’)
- Use while loop:
- while(i!=-1);
- fil.close();
- line.close();
- while(i!=-1);
Source Code:
import java.io.*; class linenum { public static void main(String[] args)throws IOException { FileInputStream fil; LineNumberInputStream line; int i; try { fil=new FileInputStream(args[0]); line=new LineNumberInputStream(fil); } catch(FileNotFoundException e) { System.out.println("No such file found"); return; } do { i=line.read(); if(i=='n') { System.out.println(); System.out.print(line.getLineNumber()+" "); } else System.out.print((char)i); }while(i!=-1); fil.close(); line.close(); } }
Input:
This is file as input:Demo.java class Demo { public static void main(java Demo beta gamma delta) { int n = 1 ; System.out.println(“The word is ” + args[ n ] ); } }
Output:
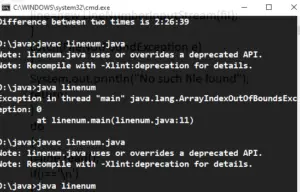
Recommended Post:
- Python Hello World Program
- Python Comment | Creating a Comment | multiline comment | example
- Python Dictionary Introduction | Why to use dictionary | with example
- How to do Sum or Addition in python
- Python Reverse number
- find the common number in python
- addition of number using for loop and providing user input data in python
- Python Count char in String
- Python Last Character from String
- Python Odd and Even | if the condition
- Python Greater or equal number
- Python PALINDROME NUMBER
- Python FIBONACCI SERIES
- Python Dictionary | Items method | Definition and usage
- Python Dictionary | Add data, POP, pop item, update method
- Python Dictionary | update() method
- Delete statement, Looping in the list In Python
- Odd and Even using Append in python
- Python | Simple Odd and Even number
Get Salesforce Answers
5 thoughts on “Write a Java program that reads a file and displays the file on the screen with a line number before each line”