Write a Java program that implements a simple client-server application
In this program, we will learn to implements a client and server application
Aim:
To Write a Java program that implements a simple client server application. The client sends data to a server. The server receives the data, uses it to produce a result, and then sends the result back to the client. The client displays the result on the console. For ex: The data sent from the client is the radius of a circle, and the result produced by the server is the area of the circle. (Use java.net)
Source Code for client server application:
/*Program for the implementation of the client-server application*/
import java.io.*;
import java.net.*;
public class Server
{
public static void main(String rr[])throws IOException
{
ServerSocket ss=new ServerSocket(8000);
Socket s=ss.accept();
DataInputStream dis=new DataInputStream(s.getInputStream());
PrintStream ps=new PrintStream(s.getOutputStream());
String str=dis.readLine();
double r=new Double(str).doubleValue();
System.out.println("Radius Given by client:"+r);
double area=3.14*r*r;
ps.println(area);
ss.close();
}
}
/*Program for the implementation of the client server application*/
import java.io.*;
import java.net.*;
public class Client
{
public static void main(String rr[])throws Exception
{
DataInputStream dis1,dis2;
String host;
PrintStream ps;
if(rr.length==0)
host="LocalHost";
else
host=rr[0];
Socket s=new Socket(host,8000);
System.out.println("Enter the radius:");
dis1=new DataInputStream(System.in);
dis2=new DataInputStream(s.getInputStream());
double r=new Double(dis1.readLine());
ps=new PrintStream(s.getOutputStream());
ps.println(r);
String a=dis2.readLine();
System.out.println("Area Calculated by server:"+a);
s.close();
}
}
Expected Output of client server application:
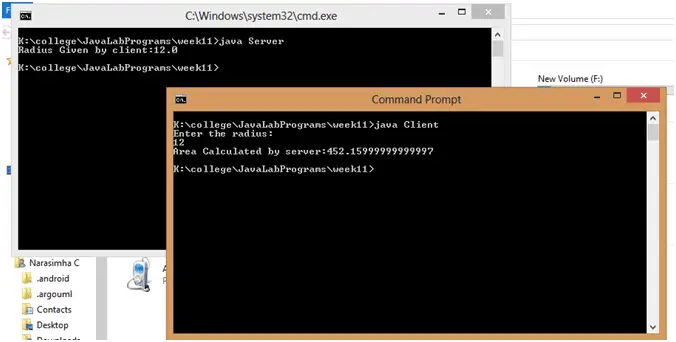
FAQ:
How do I run a client-server program in Java?
First, we have to connect with the host using the port number. Connection in server-side: ServerSocket ss=new ServerSocket(8000);
Socket s=ss.accept();
Connection in client side: Socket s=new Socket(host,8000);
What is client and server in Java?
Client means the System which is requesting to the server for data and Server means backend or system which serves the data.
What is port number in Java?
Port number is a unique identification number that is used to connect the two different systems to share data.
What is TCP and UDP?
TCP is a connection-oriented protocol and UDP is a connectionless protocol. TCP establishes a connection between client and server before to share data. UDP does not have to establish a connection before sending data.
Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Write a java program to Checks whether a given string is a palindrome or not
- Write a java program to perform multiplication of two matrices
- write a java program that prints the Fibonacci series for a given number.
- Write a Java program that finds the factorial of a number
- Write a Java program that finds prime numbers between 1 to n
- Write a Java program that prints all real and imaginary solutions to the quadratic equation
- Odd and Even number in java | Algorithm
- Even number in java | algorithm with source code
- Java greater number using a loop
- Java Area of Rectangle
- What is a polygon? | Area of Triangle in java
- To calculate the Area of Circle in the java program
- Java Addition through user input
- Addition program in java with source code
Find the solution to the salesforce Question and many more