Write a program that creates a user interface to perform integer divisions
An interface in Java is a template of a class. It has static constants and abstract methods.
Java has a mechanism to achieve interface abstraction. The Java interface can only contain abstract methods, not method entities. It is used to achieve abstraction and multiple inheritance in Java.
In other words, you can say that interfaces can contain abstract methods and variables. It cannot be a method body.
Aim to write a program that creates user interface
To write a program that creates a user interface to perform integer divisions. The user enters two numbers in the text fields, Num1 and Num2. The division of Num1 and Num2 is displayed in the Result field when the Divide button is clicked. If Num1 or Num2 were not an integer, the program would throw a NumberFormatException. If Num2 were Zero, the program would throw an ArithmeticException Display the exception in a message dialog box.
Description:
- NumberFormatException arises when a non-integer is divided with any non-integer value.
- ArithmeticException arises when a number is divided by zero.
Read input of two values, click on compute. If there is any NumberFormatException, a dialog is raised. If there is any ArithmeticException, another dialog is raised.
Source code to write a program that create user interface
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.applet.*;
public class Week10 extends Frame implements ActionListener
{
Dialog d;
TextField t1,t2,t3;
Button comp;
public Week10()
{
setLayout(new FlowLayout());
setSize(500,500);
t1=new TextField(10);
t2=new TextField(10);
t3=new TextField(10);
comp=new Button("Compute ");
add(new Label("Enter a: "));
add(t1);
add(new Label("Enter b: "));
add(t2);
add(new Label("Result : "));
add(t3);
add(comp);
comp.addActionListener(this);
setVisible(true);
}
pubic void actionPerformed(ActionEvent ae)
{
if(ae.getSource()==comp)
{
try
{
t3.setText(Integer.toString((Integer.parseInt(t1.getText().trim()))/(Integer.parseInt(t2.getText().trim()))));
}
catch(ArithmeticException aex)
{
Dia d1=new Dia("Arithmetic Exception");
d1.setVisible(true);
}
catch(NumberFormatException nfe)
{
Dia d2=new Dia("Number Format Exception ");
d2.setVisible(true);
}
}
}
public static void main(String ar[])
{
new Week10();
}
}
class Dia extends Dialog implements ActionListener
{
Button cancel;
Dia(String str)
{
super(new Frame(),str,true);
cancel=new Button("Cancel");
setLayout(new FlowLayout());
setSize(300,200);
add(new Label("Press the Button"));
add(cancel);
cancel.addActionListener(this);
}
public void actionPerformed(ActionEvent ae)
{
setVisible(true);
}
public void paint(Graphics g)
{
g.drawString("Exception Occured ",10,70);
}
}
a
OUTPUT
without any exceptions


Number Format Exception
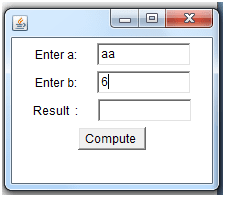
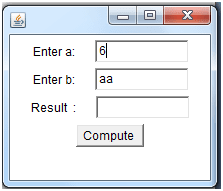
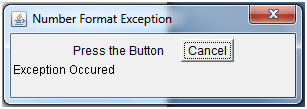
Arithmetic Exception
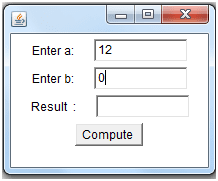
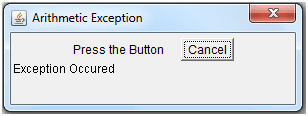
Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Write a java program to Checks whether a given string is a palindrome or not
- Write a java program to perform multiplication of two matrices
- write a java program that prints the Fibonacci series for a given number.
- Write a Java program that finds the factorial of a number
- Write a Java program that finds prime numbers between 1 to n
- Write a Java program that prints all real and imaginary solutions to the quadratic equation
- Odd and Even number in java | Algorithm
- Even number in java | algorithm with source code
- Java greater number using a loop
- Java Area of Rectangle
- What is a polygon? | Area of Triangle in java
- To calculate the Area of Circle in the java program
- Java Addition through user input
- Addition program in java with source code
Find the solution to the salesforce Question and many more