This tutorial covers the next matter – Python Add Two checklist Elements. It describes 4 distinctive methods to add the checklist objects in Python. For instance – utilizing a for loop to iterate the lists, add corresponding parts, and retail their sum on a similar index in a brand new checklist. Some of the opposite strategies you should use are utilizing map() and zip() strategies.
All of those procedures use constructed-in features in Python. However, whereas utilizing the map(), you’ll require the add() methodology, and zip() will be used with the sum() operation. Both these routines are outlined within the operator module, so you would need to import them into your program. After ending up this publication, you possibly can assess which of those methods is extra appropriate to your state of affairs.
By the way, which, will probably be helpful in case you have some elementary data concerning the Python list. If not, please undergo the linked tutorial.
Python Add Two List Elements – 4 Unique Ways
For loop so as to add parts of two lists
It is the only strategy in Python to add two checklist parts. In this methodology, a for loop is used to iterate the smaller of the 2 lists. In each iteration, add the corresponding values on the working index from the 2 lists, and insert the sum in a brand-new checklist.
# Python add two checklist parts utilizing for loop # Setting up lists in_list1 = [11, 21, 34, 12, 31, 26] in_list2 = [23, 25, 54, 24, 20] # Display enter lists print ("nTest Input: **********n Input List (1) : " + str(in_list1)) print (" Input List (2) : " + str(in_list2)) # Using for loop # Add corresponding parts of two lists final_list = [] # Choose the smaller checklist to iterate list_to_iterate = len(in_list1) < len(in_list2) and in_list1 or in_list2 for i in vary(0, len(list_to_iterate)): final_list.append(in_list1[i] + in_list2[i]) # printing resultant checklist print ("nTest Result: **********n Smaller list is : " + str(list_to_iterate)) print (" Resultant list is : " + str(final_list))
The above program will yield the next output:
(*4*)
List comprehension so as to add parts of two lists
Checklist comprehension is a singular shorthand method in Python to create lists at runtime. It lets the programmer carry out any operation on the entered checklist parts.
This methodology can be a bit sooner for manipulating the checklist information construction. Check out the under-pattern Python program.
# Python add two checklist parts utilizing checklist comprehension # Setting up lists in_list1 = [11, 21, 34, 12, 31] in_list2 = [23, 25, 54, 24, 20, 27] # Display enter lists print ("nTest Input: **********n Input List (1) : " + str(in_list1)) print (" Input List (2) : " + str(in_list2)) # Using checklist comprehension # Add corresponding parts of two lists final_list = [] # Choose the smaller checklist to iterate list_to_iterate = len(in_list1) < len(in_list2) and in_list1 or in_list2 final_list = [in_list1[i] + in_list2[i] for i in vary(len(list_to_iterate))] # printing resultant checklist print ("nTest Result: **********n Smaller list is : " + str(list_to_iterate)) print (" Resultant list is : " + str(final_list))
This program will produce the next outcome:
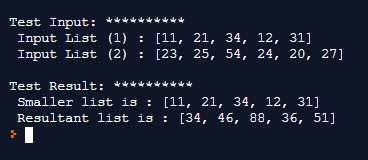
map() and add() features so as to add given lists
map() is likely one of the greater-order features in Python. It takes one other methodology as its first argument, alongside the 2 lists.
Both the entry lists are handed to the enter operate (add() in our case) as parameters. This operation provides the weather of two lists and returns an iterable as output.
We convert the iterable into an inventory utilizing the checklist constructor methodology. Check out the whole instance under:
# Python add two checklist parts utilizing map() and add() from operator import add # Setting up lists in_list1 = [11, 21, 34, 12, 31] in_list2 = [23, 25, 54, 24, 20, 27] # Display enter lists print ("nTest Input: **********n Input List (1) : " + str(in_list1)) print (" Input List (2) : " + str(in_list2)) # Using map() and add() # Add corresponding parts of two lists final_list = checklist(map(add, in_list1, in_list2)) # printing resultant checklist print ("nTest Result: **********n Resultant list is : " + str(final_list))
This instance will give the next final result:
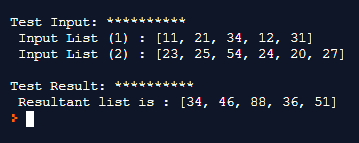
zip() and sum() features so as to add given lists
The sum() operation provides checklist parts one after the other utilizing the index. And the zip() operate teams the 2 checklist objects collectively.
This strategy can be a sublime strategy to obtain – Python Add two checklist parts. Check out the complete instance with the supply code under:
# Python add two checklist parts utilizing zip() and sum() # Setting up lists in_list1 = [11, 21, 34, 12, 31, 77] in_list2 = [23, 25, 54, 24, 20] # Display enter lists print ("nTest Input: **********n Input List (1) : " + str(in_list1)) print (" Input List (2) : " + str(in_list2)) # Using zip() and sum() # Add corresponding parts of two lists final_list = [sum(i) for i in zip(in_list1, in_list2)] # printing resultant checklist print ("nTest Result: **********n Resultant list is : " + str(final_list))
The above code will present the next output:
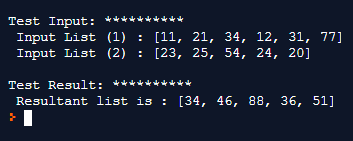
You’ve seen numerous strategies right here for Python Add two checklist parts. Now, you possibly can select any of them based mostly on your corporation case. By the way in which, to be taught Python from scratch to depth, do learn our step-by-step Python tutorial.
FAQ: Python List Addition of two elements
Python Add Two List Elements
It is the only strategy in Python to add two checklist parts. In this methodology, a for loop is used to iterate the smaller of the 2 lists. In each iteration, add the corresponding values on the working index from the 2 lists, and insert the sum in a brand-new checklist.
# Python add two checklist parts utilizing for loop ………
List comprehension so as to add parts of two lists
Checklist comprehension is a singular shorthand method in Python to create lists at runtime. It lets the programmer carry out any operation on the entered checklist parts.
This methodology can be a bit sooner for manipulating the checklist information construction. Check out the under-pattern Python program.
map() and add() features so as to add given lists
map() is likely one of the greater-order features in Python. It takes one other methodology as its first argument, alongside the 2 lists.
Both the entry lists are handed to the enter operate (add() in our case) as parameters. This operation provides the weather of two lists and returns an iterable as output.
We convert the iterable into an inventory utilizing the checklist constructor methodology. Check out the whole instance under:
zip() and sum() features so as to add given lists
Here sum() operation provides checklist parts one after the other utilizing the index. And the zip() operate teams the 2 checklist objects collectively.
This strategy can be a sublime strategy to obtain – Python Add two checklist parts.