In Python programming, iteration occurs everywhere. Mostly it involves lists, tuples, and strings because they’re iterable. In case you’re lost on the word Python Iterator, don’t be alarmed. In three minutes read from now, you’ll have a vivid understanding of the following subjects:
- What Is Python Iterator?
- Python Iterator And Iterables
- How To Create An Iterator In Python?
- Custom Iterator
- Python For Loop For Iterator
- Infinite Iterators
In the summary, you’ll be all set to carry out some iterating exercise
What are Python Iterators
In simple terms, Python Iterator is simply an object with a countable value. It’s the collection of objects that your can count on, meaning that you can count through the whole values in the object repeatedly. An iterator uses the iterator protocol. The iterator protocol consists of two methods which are __iter__() and __next__().
- __iter__(): this method is used for the initialization of an iterator. It returns an iterator object
- __next__(): this method gives out the values for the iterable. It also raises the stop iteration in order to signal the stop iteration.
Progressively, let’s clear some clogged about iterator and miserable. An iterator returns one element at a time.
Python Iterators and Iterables
Straight up, Python Iterables are the data types such as list, dictionaries, strings, tuples, and set that carries the iterator. You can get an iterator from each object by using the iter() function. An iterator, on the other hand, is the content in the iterables.
Let’s see some examples of tuples and strings returning an iterator
Example 1: a tuple returning an iterator
Python Input
mytuple = ("sugar", "strawberry ", "milkshake") myit = iter(mytuple) print(next(myit)) print(next(myit)) print(next(myit))
Output: sugar strawberry milkshake
Example 2: a string returning an iterative
Python Input
mystr = "Simonmonday " myit = iter(mystr) print(next(myit)) print(next(myit)) print(next(myit)) print(next(myit)) print(next(myit)) print(next(myit))
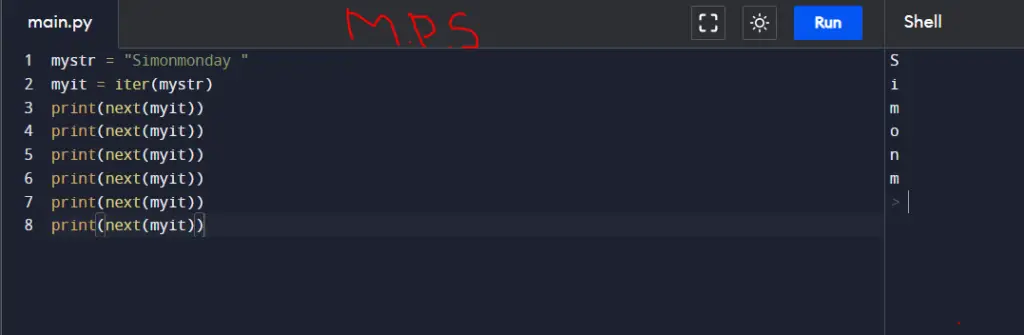
How to Create an Iterator in Python?
Though we’ve seen some examples of iterators, however, let’s go deep on the steps to create iterators in python. To create the python iterator, we must consider the two necessary methods which are the _iter_ and _next_(). Let’s begin
- You create a sequence of elements that you wish to iterate on. Let’s use: nums = [2, 3, 7, 5, 9, 1]
- Use the __iter__ to call out the miserable object from the code nums_iter = iter( nums )
print( nums_iter ). When done properly on the list object, you should have something similar to: <list_iterator object at 0x00000258BF9CE5C0>
- To access the elements from the iterator, use the __next__ functions. That is print(next(nums_iter)). The method will give the iterator one after the other, when the above code is well implemented, you’ll get this:
Python Input
nums = [2, 3, 7, 5, 9, 1] nums_iter = iter( nums ) print( nums_iter ) print(next(nums_iter))
Output: <list_iterator object> 2
Note: when you keep repeating the print(next(nums_iter)), it keeps printing the next elements after the previous. Like this:
Python input
nums = [2, 3, 7, 5, 9, 1] nums_iter = iter( nums ) print( nums_iter ) print(next(nums_iter)) print(next(nums_iter)) print(next(nums_iter)) print(next(nums_iter)) print(next(nums_iter)) print(next(nums_iter))
Output: <list_iterator object> 2 3 7 5 9 1
However, it’ll raise an error report when there are no more elements to read.
Example:
Python Input
nums = [2, 3, 7, 5, 9, 1] nums_iter = iter( nums ) print( nums_iter ) print(next(nums_iter)) print(next(nums_iter)) print(next(nums_iter)) print(next(nums_iter)) print(next(nums_iter)) print(next(nums_iter)) print(next(nums_iter))
Output: <list_iterator object> 2 3 7 5 9 1
Traceback (most recent call last):
File https://extendsclass.com/lib/Brython-3.8.9/www/src/Lib/site-packages/editor.py, line 116, in run
exec(src, ns)
File <string>, line 10, in <module>
StopIteration: StopIteration
One more __next__ was added which wasn’t in the list, and it raises the error feedback
Now it’s time to customize your own iterator
Custom Iterator
You can create your own iterator from scratch. It’s easy. What you should never forget is to use the __iter__ and the __next_() method. Following the steps shown above, let’s code some iterators.
Example:
Python Input
class EvenNumbers: def __iter__(self): self.num = 0 return self def __next__(self): next_num = self.num self.num += 2 return self.num evens = EvenNumbers() even_iter = iter(evens) print(next(even_iter)) print(next(even_iter)) print(next(even_iter)) print(next(even_iter)) print(next(even_iter)) print(next(even_iter)) print(next(even_iter))
Output: 2 4 6 8 10 12 14
This is an infinite iterator because you keep getting a value the more you repeat the print command. To bring the reoccurrence to a stop, you’ll use the for loop to iterate over the elements in order to raise the stop iterator function.
Python for loop for Iterators
In python, we can use the for loop to iterate over the elements. A python for loop is used to repeat count over python sequences. Refer to python for a loop. Progressively, the for loop automatically iterate over the elements in a sequence until the task is done.
Example:
Python Input
for city in ("Dome", "Platu", "Chinto", "Pami"): print(city)
Output: Dome Platu Chinto Pami
Infinite Iterators in Python
For short, Infinite Iterators in Python means iterator with no end. However, we must be careful when dealing with infinite iterators. The protocol uninvolved in the infinite iterator is that the __iter_ function will be called with two arguments. Where you must be able to call the first function and the second should be used as Sentinel(to terminate the loop)
Example:
Python Input
class EvenNumbers: def __iter__(self): self.num = 0 return self def __next__(self): if(self.num < 10): next_num = self.num self.num += 2 return self.num else: raise StopIteration evens = EvenNumbers() even_iter = iter(evens) for n in even_iter: print(n)
Output: 2 4 6 8 10
A point to remember is always to remember to raise the stop iteration order so that you can conclude a project.
Summary
Remember that:
In simple terms, Python Iterator is simply an object with a countable value. It’s the collection of objects that your can count on, meaning that you can count through the whole values in the object repeatedly.
An iterator uses the iterator protocol. The iterator protocol consists of two methods which are __iter__() and __next__().
Python Iterables are the data types such as list, dictionaries, strings, tuples, and set that carries the iterator. You can get an iterator from each object by using the iter() function. An iterator, on the other hand, is the content in the iterable
You can create your own iterator from scratch. It’s easy. What you should never forget is to use the __iter__ and the __next_() method. Following the steps shown above, let’s code some iterators.
At the end of this page you’ve learned:
- What Is Python Iterator?
- Python Iterator And Iterables
- How To Create An Iterator In Python?
- Custom Iterator
- Python For Loop For Iterator
- Infinite Iterators
Give it all your efforts and be rewarded with excellence. For a helping hand reader to the Python Tutorials. Good Lick Coding!