In this tutorial, we’ll describe Python’s Multiple Inheritance idea and clarify the right way to use it in your packages. We’ll additionally cowl multilevel inheritance, the tremendous() operate, and deal with the tactic decision order.
In the earlier tutorial, we’ve got gone via Python Class and Python (Single) Inheritance. There, you will have seen {that a} little one class inherits from a base class. However, Multiple Inheritance is a characteristic of the place a class can derive attributes and strategies from a couple of base lessons. Hence, it creates an excessive degree of complexity and ambiguity and is recognized as the diamond downside in the technical world. We’ll be taking on this downside later in this tutorial.
A basic notion of Multiple Inheritance is that it is both “dangerous” and “bad.” Also, Java doesn’t permit Multiple Inheritance, while C++ does have it. However, Python lays down a mature and nicely-designed method to deal with a number of Inheritance.
Python Multiple Inheritance Explained
Let’s now try to know the subject in element.
Please do undergo every part fastidiously to know these OOPs ideas inside out.
What is Multiple Inheritance?
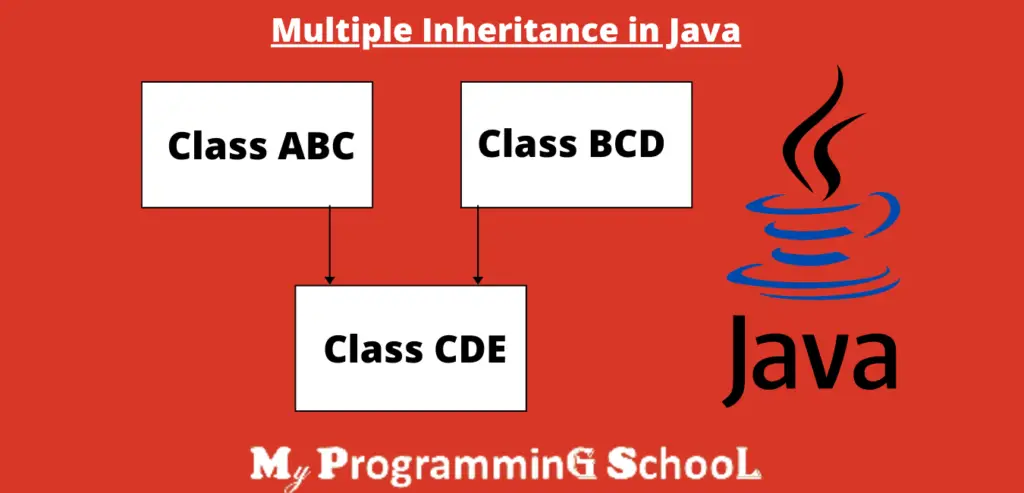
When you inherit a baby class from a couple of base lessons, that scenario is recognized as Multiple Inheritance. It, nonetheless, reveals identical habits as does the only inheritance.
The syntax for Multiple Inheritance is additionally much like the only inheritance. In the best way, in Multiple Inheritance, the kid class claims the properties and strategies of all of the mother or father lessons.
In Python, the tasks and packages comply with a precept referred to as DRY, i.e., don’t-repeat-your self. And Class inheritance is a wonderful method to design a class reusing the options of one other one and staying DRY.
Basic Python Multiple Inheritance Example
""" Desc: Python program to exhibit the diamond downside (a.okay.a. Multiple Inheritance) """ # Parent class 1 class TeamMember(object): def __init__(self, identify, uid): self.identify = identify self.uid = uid # Parent class 2 class Worker(object): def __init__(self, pay, jobtitle): self.pay = pay self.jobtitle = jobtitle # Deriving a baby class from the 2 mother or father lessons class TeamChief(TeamMember, Worker): def __init__(self, identify, uid, pay, jobtitle, exp): self.exp = exp TeamMember.__init__(self, identify, uid) Worker.__init__(self, pay, jobtitle) print("Name: , Pay: , Exp: ".format(self.identify, self.pay, self.exp)) TL = TeamChief('Jake', 10001, 250000, 'Scrum Master', 5)
The output is:
Name: Jake, Pay: 250000, Exp: 5
Overriding Methods Example
When you outline a mother or father class methodology in the kid, then this course of is referred to as Overriding.
In different phrases, a baby class can override the strategies of its mother or father, or superclass by defining an operation with an identical identity.
However, there are some guidelines for overriding:
- The identity of the tactic must be identical and its parameters as nice.
- If the superclass methodology is personal (prefixed with double underscores), then you may override it.
In Python, you should use the tremendous() methodology for overriding. It has the next syntax:
# Override utilizing the tremendous() methodology tremendous(class_name, self).override_method_name()
Check the beneath instance.
""" Desc: Python program to exhibit overriding utilizing the tremendous() methodology """ class base(object): def base_func(self): print('Method of base class') class little one(base): def base_func(self): print('Method of kid class') tremendous(little one, self).base_func() class next_child(little one): def base_func(self): print('Method of next_child class') tremendous(next_child, self).base_func() obj = next_child() obj.base_func()
The outcome is:
Method of next_child class Method of kid class Method of base class
What is Multi-level Inheritance?
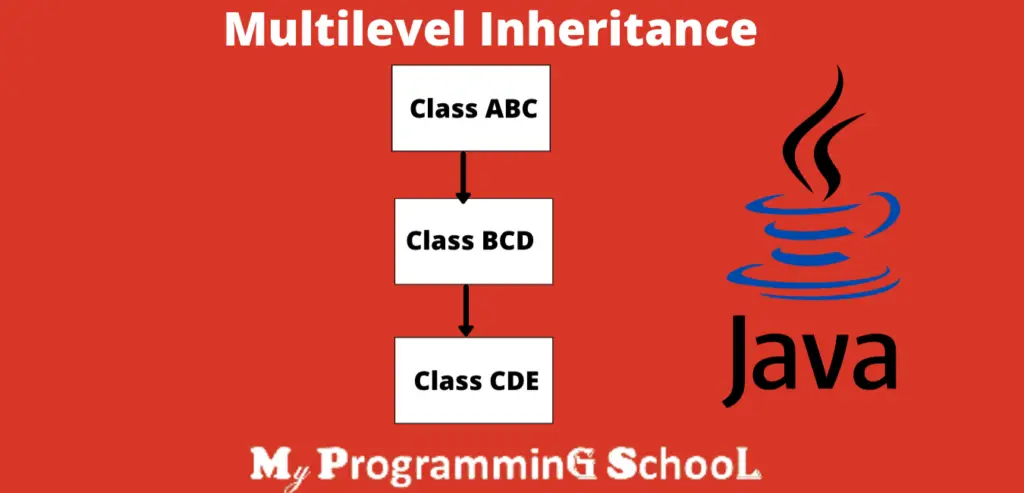
When you inherit a class from a derived class, then it’s referred to as multilevel inheritance. And, it might go up any range in Python.
In multilevel inheritance, the properties of the mother or father and the kid’s lessons can be found in the brand-new class.
Multilevel inheritance is akin to the connection between grandpa, father, and the kid. You can sense it in the beneath examples.
- An automobile derives from the automobile, which itself belongs to the car class.
- An inverter AC is a subclass of the AC class, which pertains to the Appliance superclass.
Below is an easy illustration depicting the multilevel inheritance.
class mother or father: pass class little one(mother or father): pass class next_child(little one): pass
You can observe the next by trying on the above code:
- The little one class is a by-product of the mother or father.
- The next_child class is a by-product of the kid.
Python Multi-level Inheritance Example
""" Desc: Python program to exhibit multilevel inheritance """ class Team: def show_Team(self): print("This is our Team:") # Testing class inherited from Team class Testing(Team): TestingName = "" def show_Testing(self): print(self.TestingName) # Dev class inherited from Team class Dev(Team): DevName = "" def show_Dev(self): print(self.DevName) # Sprint class inherited from Testing and Dev lessons class Sprint(Testing, Dev): def show_parent(self): print("Testing :", self.TestingName) print("Dev :", self.DevName) s1 = Sprint() # Object of Sprint class s1.TestingName = "James" s1.DevName = "Barter" s1.show_Team() s1.show_parent()
The output is:
This is our Team: Testing : James Dev : Barter
Python Multiple Inheritance vs. Multi-level Inheritance
The main variations between Multiple and Multilevel Inheritance are as follows:
- Multiple Inheritance denotes a state of affairs when a class derives from a couple of base lessons.
- Multilevel Inheritance means a class derives from a subclass making that subclass a mother or father for the brand new class.
- Multiple Inheritance is extra advanced and therefore not used broadly.
- Multilevel Inheritance is an extra typical case and is therefore used incessantly.
- Multiple Inheritance has two lessons in the hierarchy, i.e., a base class and its subclass.
- Multilevel Inheritance requires three ranges of lessons, i.e., a base class, an intermediate class, and the subclass.
Method Resolution Order (MRO)
Method Resolution Order (MRO) is a method {that a} programming language takes to resolve the variables or strategies of a class.
Python has a built-in base class named the object. So, another in-built or consumer-outlined class that you outline will finally inherit from it.
Now, let’s discuss how the tactic decision order (MRO) takes place in Python.
- In the number of inheritance use cases, the attribute first appeared in the present class. If it fails, then the following place to go looking is in the mother or father class, and so on.
- If there are a number of mother or father lessons, then the choice order is depth-first adopted by a left-proper path, i.e., DLR.
- MRO ensures {that a} class all the time precedes its dad and mom and for a number of dad and mom, retains the order as the tuple of base lessons.
Basic MRO Example
""" Desc: Python program to exhibit how MRO resolves a way or an attribute """ class Heap: def create(self): print(" Creating Heap") class Node(Heap): def create(self): print(" Creating Node") node = Node() node.create()
Here is the outcome:
Creating Node
MRO Example with Multiple Inheritance
""" Desc: Python program to exhibit how MRO works in a number of inheritance """ class Agile: def create(self): print(" Forming class Agile") class Dev(Agile): def create(self): print(" Forming class Dev") class QA(Agile): def create(self): print(" Forming class QA") # Ordering of lessons class Sprint(Dev, QA): pass dash = Sprint() dash.create()
Here is the output:
Forming class Dev
In this instance, we showcased the Multiple Inheritance, recognized as Diamond inheritance or Deadly Diamond of Death.
Methods for Method Resolution Order(MRO)
You can examine the Method Resolution Order of a class. Python supplies a __mro__ attribute and the mro() methodology. With these, you will get the decision order.
See the beneath instance:
""" Desc: Python program to exhibit the right way to get MRO utilizing __mro__ and mro() """ class Appliance: def create(self): print(" Creating class Appliance") class AC: def create(self): print(" Creating class AC") # Oder of lessons class InverterAC(Appliance, AC): def __init__(self): print("Constructing InverterAC") appl = InverterAC() # Display the lookup order print(InverterAC.__mro__) print(InverterAC.mro())
The outcome is:
Constructing InverterAC (<class '__main__.InverterAC'>, <class '__main__.Appliance'>, <class '__main__.AC'>, <class 'object'>) [<class '__main__.InverterAC'>, <class '__main__.Appliance'>, <class '__main__.AC'>, <class 'object'>
Built-in Inheritance Methods in Python
Python gives us two built-in methods to check inheritance. Here, they are:
a. isinstance()
The isinstance() function tests an object type. It returns True or False accordingly.
# Syntax isinstance(object, class)
Check the below example:
# Python issubclass() example num = 1.0 print("Is the number of float class type? ".format(num, isinstance(num, float)))
Here is the result:
Is the number 1.0 of float class type? True
b. issubclass()
The issubclass() function tests if a particular class inherits another class or not. It has the following syntax:
# Syntax issubclass(child_class, parent_class)
It results in True if the given class is actually derived from the parent or returns False otherwise.
""" Desc: Python program to showcase issubclass() """ class parent(): def __init__(self, x): self.x = x class child(parent): def __init__(self, x, y): self.y = y parent.__init__(self, x) print("Is the child derived from the parent class? ".format(issubclass(child, parent)))
The result is as follows:
Is the child derived from the parent class? True
After wrapping up this tutorial, you should feel comfortable in using Python Multiple Inheritance. However, we’ve provided you with enough examples to practice more and gain confidence.
Also, to learn Python from scratch to depth, do read our step-by-step Python tutorial.