In this tutorial, we will discuss the Python Random Number. We will see everything step by step. we will also syntax and example related to it.
Python has a constructed-in random module for this objective. It exposes a number of strategies reminiscent of randrange(), randint(), random(), seed(), uniform(), and many others. You can name any of those features to generate a Python random quantity.
Usually, a random quantity is an integer, however, you possibly can generate float random additionally. However, you first want to grasp the context as a programmer after which choose the best operation to make use of.
A Python Random Number Tutorial for Beginners
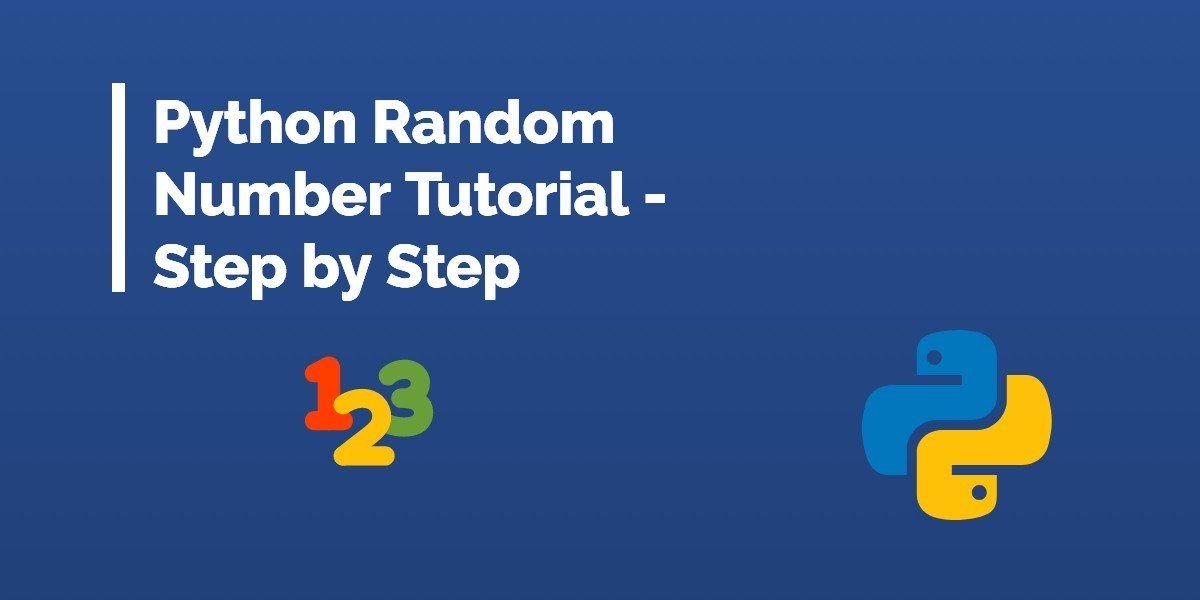
Python random quantity generator
Python random quantity generator is a deterministic system that produces pseudo-random numbers. It makes use of the Mersenne Twister algorithm that may generate an inventory of random numbers.
A deterministic algorithm at all times returns the identical outcome for the identical enter.
It is by far essentially the most profitable PRNG method applied in numerous programming languages.
A pseudo-random quantity is statistically random, however has an outlined place to begin and should repeat itself.
Let’s now take a look at some actual examples to generate random integer and float numbers.
Randrange() to generate a random quantity
The randrange() operation is obtainable with the Python random module. It makes you produce a random quantity from a given variety of values.
This operation has the next three variations:
Syntax:
# randrange() with all three arguments random.randrange(start, finish, step counter)
While utilizing this type of randrange(), you must move the beginning, cease, and step values.
# randrange() with first two arguments random.randrange(start, finish)
With this kind, you present the beginning and cease, and the default (=1) is used because of the step counter.
# randrange() with a single argument random.randrange(finish)
You solely must move the endpoint of the vary. The worth (=0) is taken into account for the place to begin and the default (=1) for the step counter.
Also, please be aware the top/cease worth is excluded when producing a random quantity. And this operation produces the ValueError exception within the following circumstances:
- If you’ve used a float vary for the beginning/cease/step.
- Or if the beginning worth is greater than cease worth.
Let’s now take a look at a couple of examples.
Python random quantity between 0 and 100
import random as rand # Generate random quantity between 0 to 100 # Pass all three arguments in randrange() print("First Random Number: ", rand.randrange(0, 100, 1)) # Pass first two arguments in randrange() print("Second Random Number: ", rand.randrange(0, 100)) # Default step = 1 # Or, you possibly can solely move the beginning argument print("Third Random Number: ", rand.randrange(100)) # Default begin = 0, step = 1
The output is:
First Random Number: 3 Second Random Number: 36 Third Random Number: 60
Python random quantity between 1 and 99
import random as rand # Generate random quantity between 1 to 99 # Pass all three arguments in randrange() print("First Random Number: ", rand.randrange(1, 99, 1)) # Pass first two arguments in randrange() print("Second Random Number: ", rand.randrange(1, 99)) # Default step = 1
The results:
First Random Number: 18 Second Random Number: 77
Randint() for the inclusive vary
The randint() operate is considerably much like the randrange(). It, too, generates a random integer quantity from vary. However, it differs a bit:
- Randint() has two obligatory arguments: begin and cease
- It has an inclusive vary, i.e., can return each endpoint because of the random output.
Syntax:
# random module's randint() operate random.randint(begin, cease)
This operation considers each of the starts and finishes values whereas producing the random output.
Randint() random quantity generator instance
import random as rand # Generate random quantity utilizing randint() # Simple randint() instance print("Generate First Random Number: ", rand.randint(10, 100)) # randint() together with seed() rand.seed(10) print("Generate Second Random Number: ", rand.randint(10, 100)) rand.seed(10) print("Repeat Second Random Number: ", rand.randint(10, 100))
The following is the outcome:
Generate First Random Number: 14 Generate Second Random Number: 83 Repeat Second Random Number: 83
Random() to generate a random float quantity
It is among the most simple features of the Python random module. It computes a random float quantity between 0 and 1.
This operation has the next syntax:
Syntax:
# random() operate to generate a float quantity random.random()
Generate a random float quantity between 0 and 1
import random as rand # Generate random quantity between 0 and 1 # Generate first random quantity print("First Random Number: ", rand.random()) # Generate second random quantity print("Second Random Number: ", rand.random())
The following is the output after execution:
First Random Number: 0.6448613829842063 Second Random Number: 0.9482605596764027
Seed() to repeat a random quantity
The seed() methodology permits preserving the state of random() operation. It implies that when you set a seed worth and calls random(). Python then maps the given seed to the output of this operation.
So, every time you name seed() with an identical worth, the next random() returns the identical random quantity.
Syntax:
# random seed() operate random.seed([seed value])
The seed is a non-compulsory discipline. Its default worth is None, which suggests making use of the system time for random operation.
Seed() operate with random() instance
import random as rand # Generate random quantity utilizing seed() # Using seed() w/o any argument rand.seed() print("Generate First Random Number: ", rand.random()) rand.seed() print("Generate Second Random Number: ", rand.random()) # Using seed() with an argument rand.seed(5) print("Generate Third Random Number: ", rand.random()) rand.seed(5) print("Repeat Third Random Number: ", rand.random())
Here is the execution outcome:
Generate First Random Number: 0.6534144429163206 Generate Second Random Number: 0.4590722400270483 Generate Third Random Number: 0.6229016948897019 Repeat Third Random Number: 0.6229016948897019
Please be aware that you could even pair up seed() with different Python random features reminiscent of randint() or randrange().
Uniform() to generate random float numbers
The uniform() operation computes a random float quantity from the given vary. It takes two arguments to specify the variation.
The first parameter is inclusive of random processing.
Syntax:
# random uniform() operate random.uniform(begin, cease)
This operation supplies a random outcome (r) satisfying the beginning <= r < cease. Also, please be aware that each of the beginning and ceasing parameters is obligatory.
Uniform() operates to generate a random quantity
import random as rand # Generate random float quantity utilizing uniform() # Simple randint() instance print("Generate First Random Float Number: ", rand.uniform(10, 100)) print("Generate Second Random Float Number: ", rand.uniform(10, 100))
The outcome after execution is:
Generate First Random Float Number: 20.316562022174068 Generate Second Random Float Number: 27.244409254588806
Now, we’ve got come to the top of this Python random quantity generator tutorial. And we hope that it could enable you to main on the best path. You might also wish to learn the under-publish.