This Python programming model discloses how to replace events (single or the entirety) of a character in a string. It tells the total rationale exhaustively.
In this short instructional exercise, we will utilize Python’s str.replace() capacity to finish our errand of supplanting a single in the objective string. It’s anything but a typical prerequisite for developers when they need to adjust the first string by subbing it with some worth.
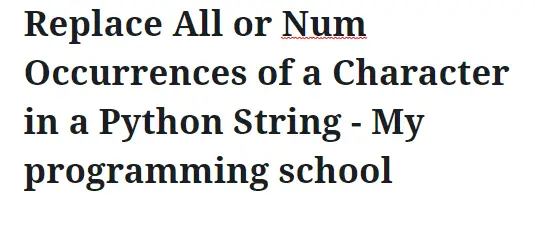
Python String Replace Function
Syntax:
#Python's String Replace Function python_string.replace(old_str, new_str, count) Parameters: old_str => Old string to be replaced new_str => New string used for substitution count => Replacement counter Returns: Returns a copy of the old string with the new after replacment.
The above work makes another string and returns something very similar. It’s anything but a duplicate of the first worth replaced with the upgraded one.
You should take note of the accompanying focuses while utilizing the string.replace() strategy:
In the event that the check boundary isn’t indicated, all events of the old string will get replaced with the enhanced one.
In the event that there is some worth in the check boundary, the old string will get replaced indicated no. of time by the enhanced one.
Presently we will see with models.
Replace all occurrences of a char/string in a string
Let’s assume this is the following original string:
original_str = "Python Programming language is very easy to learn and easy to code."
Now out the task is to replace all appearances of the string “easy” with the following string:
replace_str = "simple"
Source Program:
""" #Example: #Replace all occurrences of the specifed string in the original string """ final_str = original_str.replace("easy" , replace_str) print(final_str)
Output:
Python Programming language is very simple to learn and simple to code.
Presently we will check one more model that replaces a character with another in the first string.
original_char = '.' replace_char = '!'
Below code does the needful.
""" #Example: #Replace all occurrences of a given char in the original string """ final_str = original_str.replace(original_char , replace_char) print(final_str)
Output:
Python Programming language is very easy to learn and easy to code!
Replace num occurrences of a char/string in a string
How about we do our test with the accompanying unique string:
original_str = "Python Programming language, Python Programmer, Python Unit Testing."
Your errand is to replace the initial two events of the string “Python” with the accompanying:
replace_str = "CSharp"
""" Example: Replace first two occurrences of the specifed string in the original string """ final_str = original_str.replace("Python" , replace_str, 2) print(final_str)
Output:
CSharp Programming language, CSharp Programmer, Python Unit Testing.
Since we have provided 2 as the tally contention esteem, so it would just replace the initial two events of the “Python” string.
How to replace multiple chars/substrings in a string?
The capacity string.replace() just backings one string to get replaced. Nonetheless, you should replace different scorches or substrings in your objective string.
For instance, we may have the accompanying unique string:
orig_string = "JavaScript Programming language, CSharp Programmer, Python Unit Testing."
Presently, you need to replace “JavaScript” with “Python” and “CSharp” with “Python”. To achieve this, we have made a custom capacity.
""" #Function: #Replace a list of many sub strings with a new one in original string. """ def replace_Many(orig_str, substring_list, new_str): # Traverse the substring list to replace for string in substring_list : # Test if string exists in the original string if string in orig_str : # Replace the string orig_str = orig_str.replace(string, new_str) return orig_str
Presently, we should summon the visit custom capacity by passing the ideal boundaries.
final_str = replace_Many(orig_string, ["JavaScript", "CSharp"], "Python") print(final_str)
Output:
Python Programming language, Python Programmer, Python Unit Testing.
Recommended Posts:
- MySQL Create User with Password Explained with Examples – MPS
- SQL Exercises with Sample Tables and Demo Data – MPS
- MySQL LOWERcase/LCASE() Functions with Simple Examples – MPS
- MySQL Date and Date Functions Explained with Examples – MPS
- MySQL CURRENT_TIMESTAMP() Function Explained with Examples – MPS
- Grant Privileges on a Database in MySQL with Examples – MPs
- MySQL vs PostgreSQL Comparison – Know The Key Differences – MPS
- MySQL UPSERT | Three Techniques to Perform an UPSERT – MPs
- MySQL FROM_UNIXTIME() Function Explained with Examples – MPS