What is an exception in java? handle exception in java
Java – exception. An exception (or extraordinary event) is a problem that occurs during the execution of a program. When an exception occurs, the normal flow of the program is interrupted and the program / application terminates abnormally, which is not recommended, therefore, these exceptions have to be controlled.
Types of exception to handle exception in java
There are Two type of exception
- Checked Exception
Checked exception occur at compile time or generated by compiler.
- Unchecked Exception
Unchecked exception occured at run time
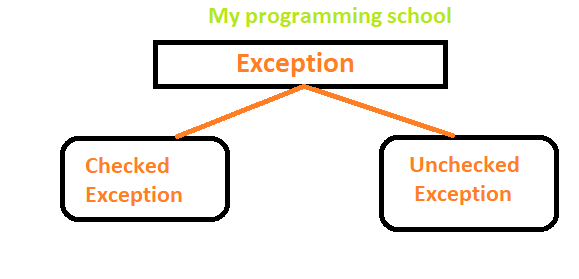
Checked and unchecked exception type
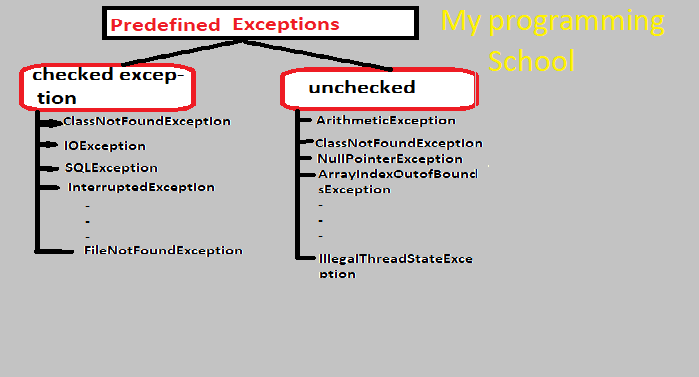
- ArithmeticException
It is thrown when an exceptional condition has occurred in an arithmetic operation.
Class: Java.lang.ArithmeticException
This is the built-in-class present in the java.lang package. This exception occurs when the integer is divided by zero.
Example:
Class example 1
{
public static void main (String [] args[])
{
try{
int num1 = 30, num2 = 0;
int output = num1 / num2;
System.out.println (“Result:” + output);
}
Catch (ArithmeticException) {
System.out.println (“You should not divide a number by zero”);
}
}
}
Output
You should not divide a number by zero
Explanation: In the above example I divided an integer by a zero and because of this an arithmetic exception was thrown.
- ArrayIndexOutOfBoundsException
It is thrown to indicate that an array has been accessed with an invalid index. The index is either negative or equal to or greater than the size of the array.
Class: Java.lang.ArrayIndexOutOfBoundsException
This exception occurs when you try to access an array index that does not exist. For example, if the array has only 5 elements and we are trying to display the 7th element it will throw an exception.
Example:
Class exception demo 2
{
public static void main (String [] args[])
{
try{
int a [] = new int [10];
Array contains only 10 elements
[11] = 4;
}
Catch (ArrayIndexOutOfBoundsException e) {
System.out.println (“ArrayIndexOutofBounds”);
}
}
}
Result
ArrayIndexOutofBounds
- ClassNotFoundException
This exception is raised when we try to access a class whose definition is not found
Example
Package com.journaldev.exception;
Public class DataTest {
public static void main (String [] args) {
try {
Class.forName (“com.journaldev.MyInvisibleClass”);
ClassLoader.getSystemClassLoader () loadClass (“com.journaldev.MyInvisibleClass”).;
ClassLoader.getPlatformClassLoader () loadClass (“com.journaldev.MyInvisibleClass”).;
} Catch (ClassNotFoundException e) {
e.printStackTrace ();
}
}
}
Result
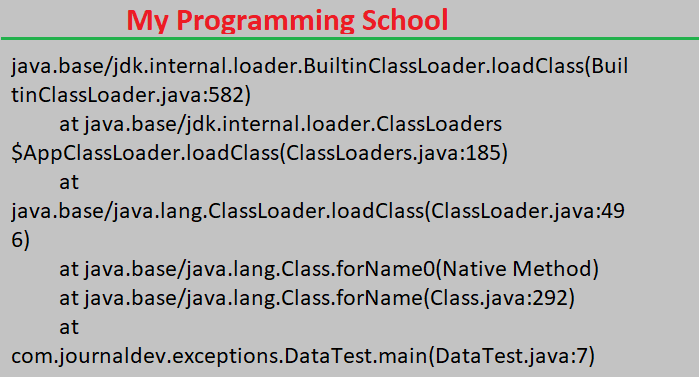
- FileNotFoundException
This exception is raised when a file is unreachable or does not open.
Example
Import java.io.BufferedReader;
Import java.io.File;
Import java.io.FileReader;
Import java.io.IOException;
Public class FileNotFoundExceptionExample {
Private static final string filename = “input.txt”;
public static void main (String [] args) {
Bufferreader rd = null;
try {
// Open the file for reading.
rd = new BufferedReader (new FileReader (new File (filename)));
// Read all the contents of the file.
String inputline = null;
While ((inputline = rd.readLine ())! = Null)
Println (inputLine);
}
Catch (before IOException) {
System.err.println (“An IOException was caught!”);
ex.printStackTrace ();
}
After all
// Close the file.
try {
rd.close ();
}
Catch (before IOException) {
System.err.println (“An IOException was caught!”);
ex.printStackTrace ();
}
}
}
}
OutPut
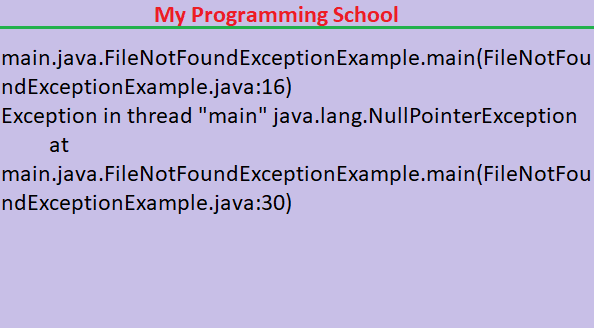
- IOException
It is thrown when the input-output operation fails or is interrupted
Example
Import java.util. *;
Public class ScannerIOExceptionExample1 {
public static void main (String [] args) {
// create a new scanner with the specified string object
ScannerScan = new Scanner (“Hello World! Hello My Programming School”);
// print the line
System.out.println (“” + scan.nextLine ());
// check if there is an IO exception
System.out.println (“Exception output:” + scan.ioException ());
scan.close ();
} }
OutPut:
Hello World! Hello My Programming School Exception output: null
- InterruptedException
It is thrown when a thread is waiting, sleeping, or processing something, and it is interrupted.
Example
Package com.javaguides.corejava;
Class childhread’s extension thread {
Public void run () {
try {
Thread.Sleep (1000);
} Catch (interrupted exception) {
System.err.println (“InterruptedException caught!”);
e.printStackTrace ();
}
}
}
Public class InterruptedExceptionExample {
Public static void main (String [] args) throws InterruptedException {
ChildThread childThread = new ChildThread ();
childThread.start ();
childThread.interrupt ();
}
}
Out Put:
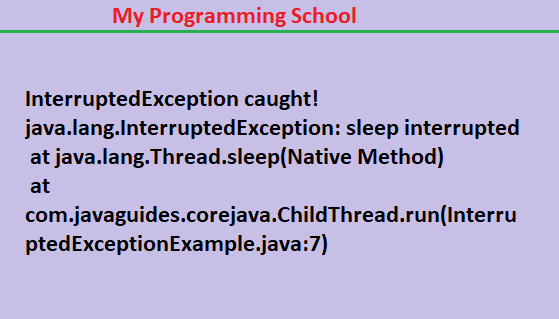
- NoSuchFieldException
It is thrown when a class does not contain a specified field (or variable)
- NoSuchMethodException
It is thrown when using a method that is not found.
- NullPointerException
This exception is raised when referring to members of a null object. Null represents nothing
Class: Java.lang.NullPointer Exception
Whenever a member is called with an “null” object, an object of this class is created.
Example
Class exception 2
{
public static void main (String [] args[])
{
try{
String str = null;
System.out.println (str.length ());
}
Catch (NullPointerException e) {
Println (“NullPointerException ..”);
}
}
}
Result
NullPointerException ..
Here, there is a length () function, which should be used on an object. However the string object str in the above example is null so it is not the object that caused the NullPointerException.
- NumberFormatException
This exception is raised when a method cannot convert a string to a numeric format.
Class: Java.lang.NumberFormatException
This exception occurs when a string is parsed on any numeric variable.
For example, the statement int num = Integer.parseInt (“XYZ”); The numbers will throw a FormatException because the string “XYZ” cannot be parsed to int.
Example
Class ExceptionDemo3
{
public static void main (String [] args[])
{
try{
int num = Integer.parseInt (“XYZ”);
Println (number);
} Catch (numberFormatException e) {
System.out.println (“Number format exception occurred”);
}
}
}
Result
Number format exception occurred
- Runtime Exception
This represents any exception that occurs during runtime.
Example
You can see above example of FileNotFoundException
- StringIndexOutOfBoundsException
It is thrown by string class methods to indicate that the index is either negative by the size of the string
Class: Java.lang.StringIndexOutOfBoundsException
Whenever an index is not implemented in a string, an object of this class is created, which is not in the range.
Each character of a string object is stored in a special index starting at 0.
To get a character present in a particular index of a string we can use charAt (int), a method of java.lang.tring. Where the difference is the logic index.
Example
Class ExceptionDemo4
{
public static void main (String [] args[])
{
try{
String str = “beginbook”;
Println (str.length ()) ;;
char c = str.charAt (0);
c = str.charAt (40);
Println (c);
} Catch (StringIndexOutOfBoundsException e) {
Println (“StringIndexOutOfBoundsException !!”);
}
}
}
Result
StringIndexOutOfBoundsException !!
For more Click HERE
Recommended Posts:
- Find the area of triangle and rectangle in java
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Write a java program to Checks whether a given string is a palindrome or not
- Write a java program to perform multiplication of two matrices
- write a java program that print the fibonacci series for a give number.
- Write a Java Program that find the factorial of a number
- Write a Java program that find prime numbers between 1 to n
- Write a Java program that prints all real and imaginary solutions to the quadratic equation
- Odd and Even number in java | Algorithm
- Even number in java | algorithm with source code
- find unique elements in array java | algorithm and source code
- Write a Java program for sorting a given list of names in ascending order
- write a java program that print the fibonacci series for a give number.
- Write a Java program that prints all real and imaginary solutions to the quadratic equation
- Missing Number in java with example
- Java greater number using loop
- Java Print function with example | Difference between print () and println () in java
- What is the Fibonacci series? in java | Displaying the Fibonacci sequence using a loop
- Odd and Even number in java | Algorithm
- Even number in java | algorithm with source code
- Java Area of Rectangle
- What is a polygon? | Area of Triangle in java
- To calculate Area of Circle in java program
- Java Addition through user input
- Producer consumer problem using threads in java
- Types of exception in java with examples
- Java applet program that displays a simple message
The exception occurred because the referenced index was not present in the string.
What is an exception in java? handle exception in java
What is an exception in java? handle exception in java|What is an exception in java? handle exception in java|What is an exception in java? handle exception in java|What is an exception in java? handle exception in java|What is an exception in java? handle exception in java|What is an exception in java? handle exception in java|What is an exception in java? handle exception in java|What is an exception in java? handle exception in java|What is an exception in java? handle exception in java|What is an exception in java? handle exception in java