Define a function that check whether the given number is Armstrong
In this program, we will create a function to checks whether the given number is Armstrong/
Armstrong Number Algorithm
- Step1. Start
- Step2. import math
- Step3. Declare the variable n and read n
- Step4. define Armstrong function
- Step5. In Armstrong function
- Step6. Assign n to a
- Step7. Initialize sum to 0
- Step8. In while Loop, perform
- d=n%10
- sum+=d*d*d
- n=math.floor(n/10)
- Step9. check the condition sum==a
- Step10 If the condition is a true print that given number is Armstrong number
- Step11. otherwise, print that given number is not Armstrong number
- Step12. Call Armstrong (n) function
- Step13. End
Armstrong Number Example
import math n=int(input("enter the number:")) def armstrong(n): a=n # 0 1 153 370 371 407 sum=0 while(n!=0): d = n%10 sum += d**3 n=math.floor(n/10) if(sum==a): print("given number is Armstrong number") else: print("given number is not Armstrong number") armstrong(n)
Output:
>>> enter the number:155 given number is not Armstrong number >>> >>> enter the number:153 given number is Armstrong number >>>
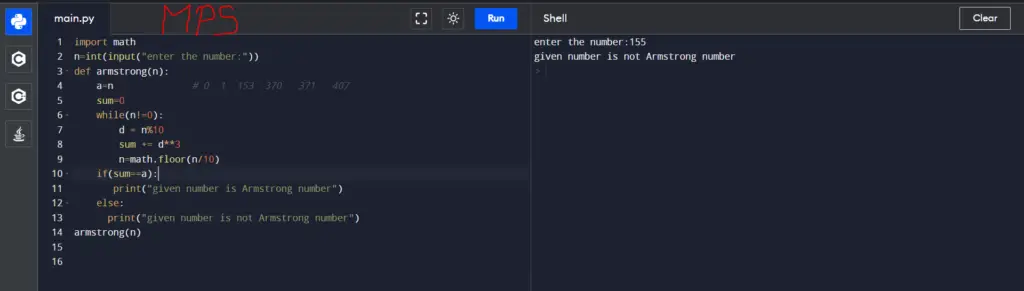
Recommended Posts:
- How to Create Forgot System Password with PHP & MySQL
- Datatables Editable Add Delete with Ajax, PHP & MySQL
- Download Login and Registration form in PHP & MySQL
- Export Data to Excel in Php Code
- Hospital Database Management System with PHP MySQL
Get Salesforce Answers