How to find unique elements in array java
Write a java program that inputs 5 numbers, each between 10 and 100 inclusive. As each number is read display it only if it‘s not a duplicate of any number already read display the complete set of unique values input after the user enters each new value.
Description :
The main two conditions of these programs are
- A number once read should not be read again.
- The number we read should be in the range of 10 and 100.
The logic is as follows
- If the number lies between 10 and 100,
Compare the recently read number with all the previously read numbers, if any number matches then declare that it a duplicate and ask the user to enter another number. Else declare that the number is not in the range of 10 and 100 and read another number.
Algorithm:
- import java package
- import java.io.*;
- import java.util.*;
- Create class Array
- create main function
- public static void main(String ar[])
- Declare variable
- int temp, i, flag = 0;
- int a[] = new int[10];
- Scanner s = new Scanner(System.in);
- Pass message to enter values
- System.out.println(” Enter values: “);
- Use for loop and write a statement and also perform the comparison.
- for( i = 0; i<5;)
- term = s.nestInt();
- flag = 0;
- if (temp > 10 && temp < 100)
- for int j= 0; j++)
- if (a[j] == temp)
- flag = 1;
- if (a[j] == temp)
- if( flag == 0);
- a [i] = temp;
- i++;
- else Print Number already exist, enter another value:
- else print number not in range
- for int j= 0; j++)
- Print Number in the array are:
- for( int j = 0; j < 5; j++)
- print a[j];
Program to find Unique Array Element Source code:
import java.io.*; import java.util.*; public class Arrayy { public static void main(String ar[]) { int temp,i,flag=0; int a[]=new int[10]; Scanner s=new Scanner(System.in); System.out.println("Enter values : "); for( i=0;i<5;) { temp=s.nextInt(); flag=0; if(temp>10 && temp<100) { for(int j=0;j<i;j++) if(a[j]==temp) flag=1; if(flag==0) { a[i]=temp; i++; } else System.out.println("Number already entered. Enter another value : "); } else System.out.println("Number not in range. Enter another values : "); } System.out.println("Numbers in the array are : "); for(int j=0;j<5;j++) System.out.println(a[j]); } }
Sample output :
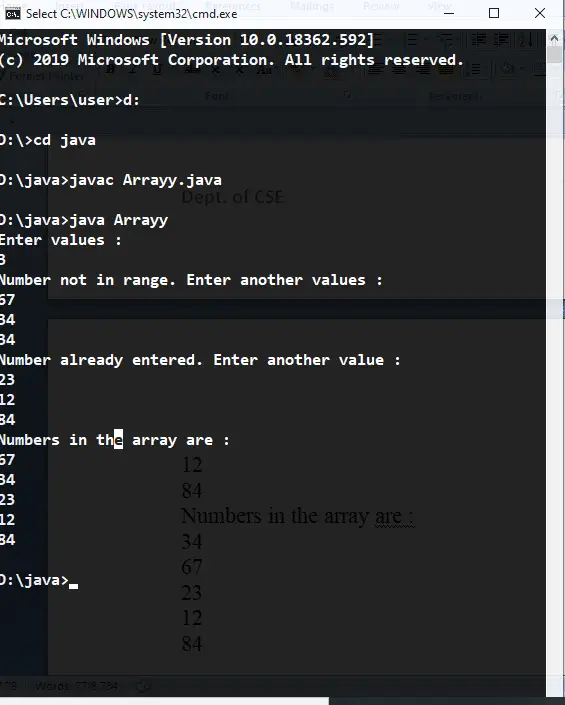
Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Write a java program to Checks whether a given string is a palindrome or not
- Write a java program to perform multiplication of two matrices
- write a java program that prints the Fibonacci series for a given number.
- Write a Java program that finds the factorial of a number
- Write a Java program that finds prime numbers between 1 to n
- Write a Java program that prints all real and imaginary solutions to the quadratic equation
- Odd and Even number in java | Algorithm
- Even number in java | algorithm with source code
- Java greater number using a loop
- Java Area of Rectangle
- What is a polygon? | Area of Triangle in java
- To calculate the Area of Circle in the java program
- Java Addition through user input
- Addition program in java with source code
Find the solution to the salesforce Question and many more