In this Lecture, we will assist you with what is a constructor in Java. You will realize how to compose a constructor method for a class and launch its object.
Basics of Constructor in Java
You can go through the following areas to find out about Java constructors.
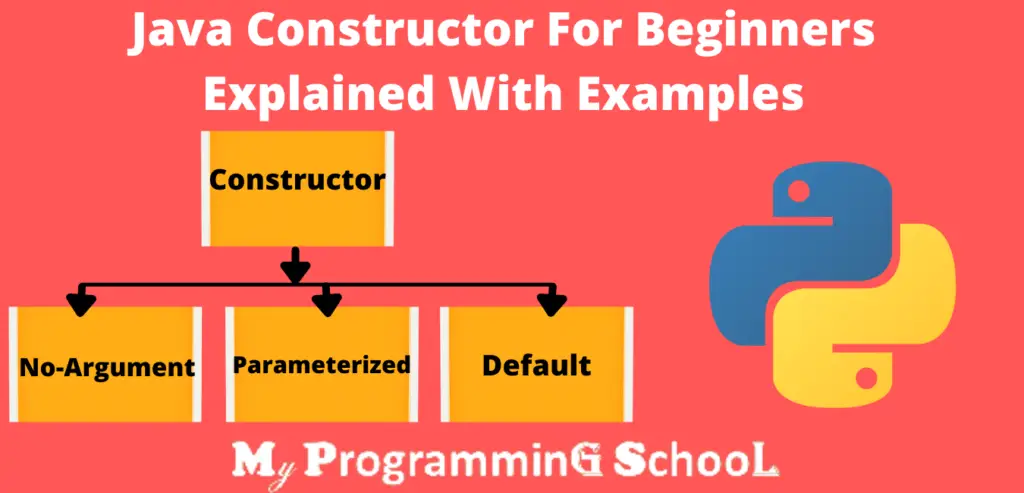
What is a constructor in Java?
In java, a constructor is a specific schedule that goes about as callback work. It implies that you don’t have to call it unequivocally. Java summons the constructor consequently while making the object of a class.
The constructor’s motivation is to introduce an object during its creation. Aside from having a similar name as its class, constructors are actually similar to some other part method.
Note: Constructors don’t have a characterized bring type back. Typically, you will use a constructor to give beginning qualities to the neighborhood class variables described inside the class or to play out some other beginning up measure needed to make another object.
What is a default constructor?
Every single class has constructors, whether or not you make one or not. Java gives a default constructor to each class. The default constructor sets every part variable to nothing.
Regardless, when you make a constructor expressly, the default constructor doesn’t come into the image.
Constructor Syntax
class className { // Constructor className() { //code } // Other methods… void method_1() { // Code } void method_2() { // code } }
When is a constructor called in Java?
At whatever point your program makes an object utilizing the new() administrator, Java runtime checks if you have an express constructor.
Assuming it discovers one, it conjures the client characterized constructor else to execute the default one that Java gives.
Check this example:
// Create a TestConstructor class public class TestConstructor { int attribute; //Create a class member //Create an eattributeplicit constructor for the TestConstructor class public TestConstructor() { attribute= 1; //Set the initial value for the class attribute attribute } public static void main(String[] args) { TestConstructor object=new TestConstructor(); //Create an object of class TestConstructor(It'll trigger the constructor) System.out.println(object.attribute); //Display the result } }
Output:
1
Types of Constructors
In Java Constructors are mainly of two types:
- Default/No-Argument Constructors
- Parameterized Constructors
No Argument Constructors:
No contention constructor is gainful when the developer would not like to give any boundaries for the object variables or individuals. With the assistance of no-contention constructors.
- The local variables of the class get initialized
- The objects or case variables will have predefined and fixed values.
No Argument Constructor Example:
let’s check the no-argument constructor with the sample code.
public class NoArgConst { int data; public NoArgConst( /*No any Arguments will pass here*/) { num= 10; } public static void main(String[] args) { NoArgConst oj=new NoArgConst(); System.out.println("data: " + oj.num); } }
Output:
data :10
Parameterized Constructors:
In the program, on the off chance that we need to decide new objects with client characterized qualities or qualities that change with time, the developer needs a constructor that acknowledges these qualities as at least one boundaries from the client as information or characterizes them himself.
- We can pass boundaries or qualities to a constructor likewise concerning some other way.
- Parameters are characterized inside the enclosures in the wake of referencing the constructor’s name.
Parameterized Constructor Example:
Constructors can accept parameters that help to set the attribute values.
let see the example:
public class Computer { int modelYears; String modelMake; String modelName; public Laptop(int year,String make,String name) { modelYears =year; modelMake =make; modelName =name; } public static void main(String[] args) { Laptop myLaptop=new Laptop(2020, "MSI", "GF63 Thin"); System.out.println(myLaptop.modelYears + " " + myLaptop.modelMake + " " + myLaptop.modelName); } }
Output:
2020 MSI GF63 Thin
What does a constructor return in Java?
Like other OOP dialects, constructors in Java don’t have a return proclamation to return any client characterized esteem.
Nonetheless, they do return the current class case. Likewise, it is totally okay to add a “return;” explanation towards the finish of a constructor method.
On the off chance that you compose it before any executable assertion, an inaccessible assertion error would happen.
Example
public class TestConstr { int data; public TestConstr( ) { data= -1; return; } public static void main(String[] args) { TestConstr obj=new TestConstr(); System.out.println("data : "+obj.data); } }
Output:
-1
Constructor Overloading in Java
Java program allows the overloading of constructors. Its JVM can identify constructors depending on the number of parameters, their types, and the sequence.
We try to understand more clearly way with example
public class ConstrOverloading { int x; double y; public ConstrOverloading( ) { System.out.println("No arg contructor called"); x= 0; y= 0.0; } public ConstrOverloading( int x, double y) { System.out.format("Order (%d, %f) based contructor called \n", x, y); this.x= a; this.y= 0.0; } public ConstrOverloading( double y, int x) { System.out.format("Order (%f, %d) based contructor called \n", y, x); this.x= 0; this.y= b; } public ConstrOverloading( int value) { System.out.format("Type(int: %d) based contructor called \n", value); this.x=value; this.y= 0.0; } public ConstrOverloading( double value) { System.out.format("Type (double:%f) based contructor called \n", value); this.y=value; this.x= 0; } public static void main(String[] args) { ConstrOverloading obj1=new ConstrOverloading(); System.out.format("x : %d, y : %f \n", obj1.x, obj1.y); ConstrOverloading obj2=new ConstrOverloading(1, 1.1); System.out.format("x : %d, y : %f \n", obj2.x, obj2.y); ConstrOverloading obj3=new ConstrOverloading(1.1, 1); System.out.format("x : %d, y : %f \n", obj3.x, obj3.y); ConstrOverloading obj4=new ConstrOverloading(1); System.out.format("x : %d, y : %f \n", obj4.x, obj4.y); ConstrOverloading obj5=new ConstrOverloading(1.1); System.out.format("x : %d, y : %f \n", obj5.x, obj5.y); } }
Output:
No argument contructor called. x :0, y :0.000000 Order (1, 1.100000) based contructor called. x : 1, y :0.000000 Order (1.100000, 1) based contructor called. x : 0, y :1.100000 Type (int: 1) based contructor called. x : 1, y :0.000000 Type (double: 1.100000) based contructor called. x : 0, y :1.100000
Related Posts
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Source link