In this article, we will help you to know about Inheritance in Java. You will realize how to utilize Inheritance for a class and reuse its all properties.
Basics of Inheritance in Java
We will go step by step to learn about Java Inheritance.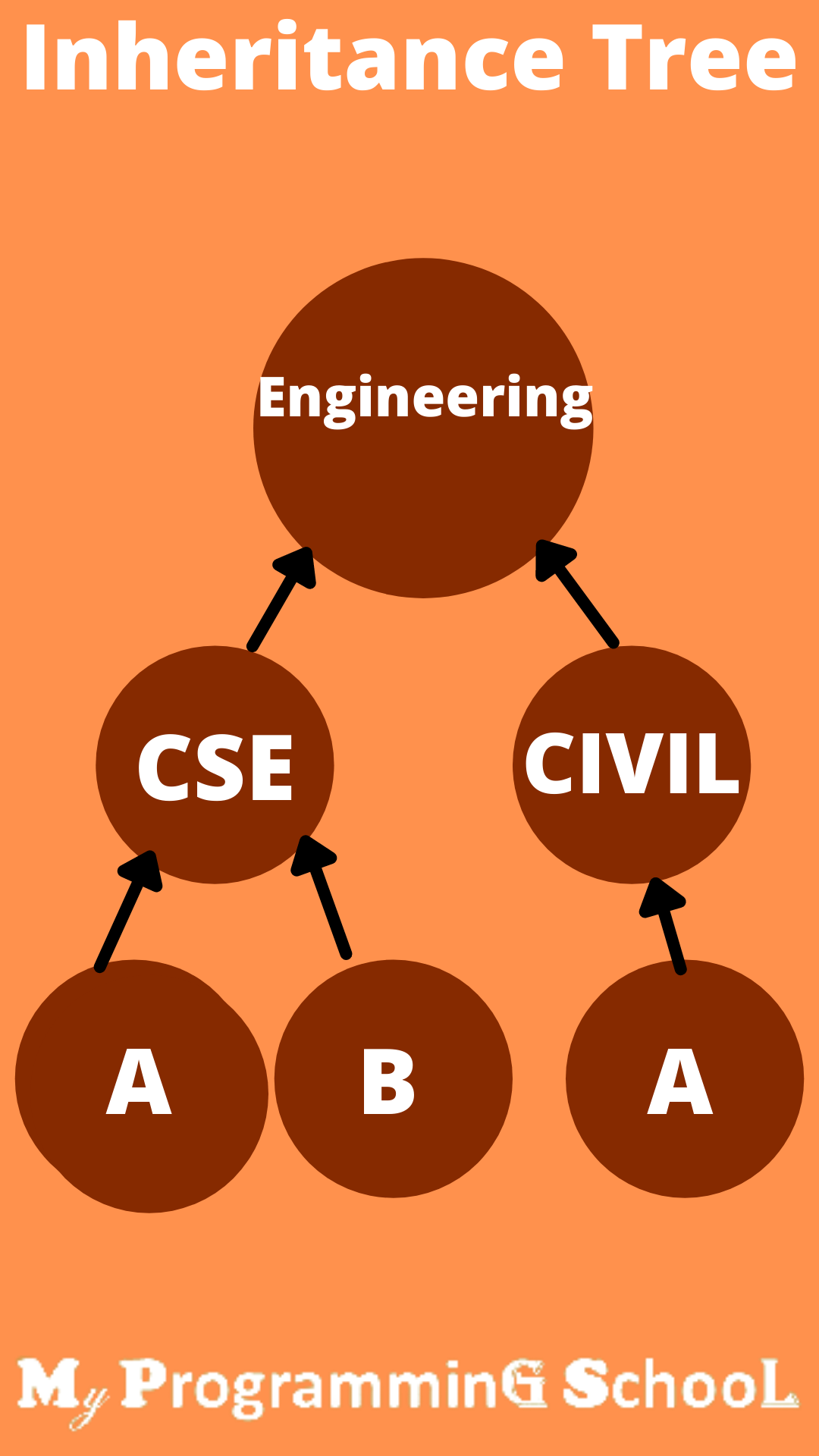
Java Inheritance Concept
Description:
In Java Inheritance is perhaps the most utilized highlights of OOP (Object Oriented Programming). Java Inheritance indicates a methodology where a recently made class extricates highlights (methods and factors/fields) from a generally existing class. With the execution of legacy, information gets accessible in a progressive request.As indicated by the progressive framework, the class which acquires the highlights of the beforehand existing class is known as a subclass or a determined or kid class.The class down the progression, from that the highlights are acquired is called parent class or superclass.In Java, you can utilize the stretches out watchword to execute the idea of legacy. The utilization of legacy is acknowledged when the programmer doesn’t wish to characterize a similar arrangement of methods or fields for the new class in the event that they are as of now characterized once.Syntax:
The syntax is given below to know and implement inheritance. You can not extend a class as long as it is not defined:class Parent_Class { // methods// // fields// } class Child_Class extends Parent_Class { // Statements // statements }
Inheritance example:
The example which is given below can help you to understand the implementation of Inheritance.class Calculation { int add(int x , int y) { return x + y; } int sub(int x , int y) { return x - y; } } public class AdvCalculation extends Calculation { int mult(int x , int y) { return x * y; } int div(int x , int y) { return x / y; } } public static void main(String args[]) { AdvancedCalculator obj = new AdvancedCalculator(); System.out.println( obj.add(1, 2) ); System.out.println( obj.sub(1, 2) ); System.out.println( obj.mult(1, 2) ); System.out.println( obj.div(1, 2) ); }The super-class Advanced-Calculator utilizes effectively characterized add and sub-methods and adds greater usefulness (augmentation and division).At the point when the article obj is made, a duplicate of substance from the Calculator class is made. It is imperative to note constructors are not acquired by sub-class as they are neither methods nor fields. Notwithstanding, the constructor of the parent class can be conjured by the youngster class.Output:
3
-1
2
0
Super keyword
Description:
The super watchword is utilized when we need to summon the constructor of the super-class from inside the sub-class. On the off chance that individuals and fields of both, the classes have indistinguishable names, the super catchphrase is utilized to separate between the super-class individuals from the sub-class individuals.the super watchword is actually similar to this catchphrase yet just utilized for the super-class, inside the sub-class.On the off chance that the super-class has a pre-characterized parametrized constructor, the sub-class needs to summon the super-class constructor utilizing the super catchphrase.Super keyword example:
The example below shows the use of super keyword:class Parent { int num = 1; int a; Parent(int a) { this.a = a; } void disp( ) { System.out.println("Number from Parent class " + num); } void getA( ) { System.out.println("Value of a " + a); } } public class Child extends Parent { int num = 2; Child(int a) { super( a ); } void disp( ) { System.out.println("Number from Child class " + num); } void methodToCallSuperKeyword( ) { System.out.println(super.num); super.disp(); } } public static void main(String args[]) { Child child = new Child(100); child.methodToCallSuperKeyword(); System.out.println(child.num); child.disp(); child.getA(); }Output:
1
Number from Parent class 1
2
Number from Child class 2
Value of a 100
The instanceof administrator can be utilized to check if a specific case has a place with a class. For an acquired class, the example of the sub-class is an instanceof both sub-class and super-class.Thus in the example above:System.out.println(child instanceof Child);
System.out.println(child instanceof Parent);
Outputs:true
true
In light of the model over, the Parent is the super-class of the Child-class. As far as the IS-A relationship, we can legitimize Child IS-A Parent. With the expands watchword, every one of the properties of a sub-class is acquired in the super-class, aside from the private individuals.HAS-A connection is utilized to decide whether a class has something specific. For instance in the above model the Child-class HAS-A methodToCallSuperKeyword().We need to remember that Multiple Inheritance in java. It implies that one class can’t expand to different classes. The idea of different Inheritance is executed by means of interfaces as talked about already.Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order