inheritance types in java with example programs (OOPs)
This tutorial will guide you on various java inheritance types available. You will know about each of them using sample Java programs.
Java Inheritance Types
You can go through the following sections to learn about Java Inheritance Types.
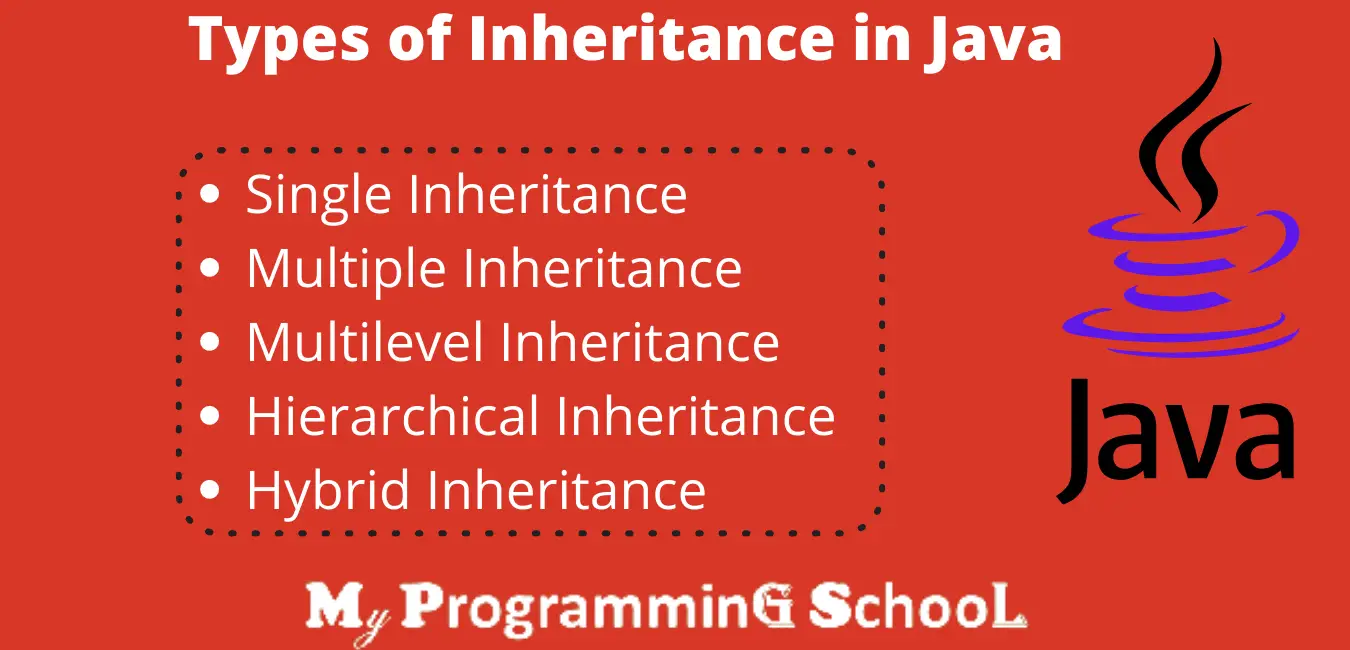
As discussed before_inheritance is one of the important concepts in Object-Oriented Programming Language. It is the process through which an already existing class extends its features to a new class. Java supports various kinds of inheritance.
Single Inheritance in Java
As the name would suggest, single inheritance_is simply one subclass extending one superclass. It is the simplest of all. The general syntax to implement single inheritance_is given below. Here, class Parent is the superclass and Child is the subclass.
class Parent
{
// methods
// fields
// ……
}
class Child extends Parent
{
// already supports the methods and fields in Parent //class
// additional features
}
Diagram Representation of Single Inheritance
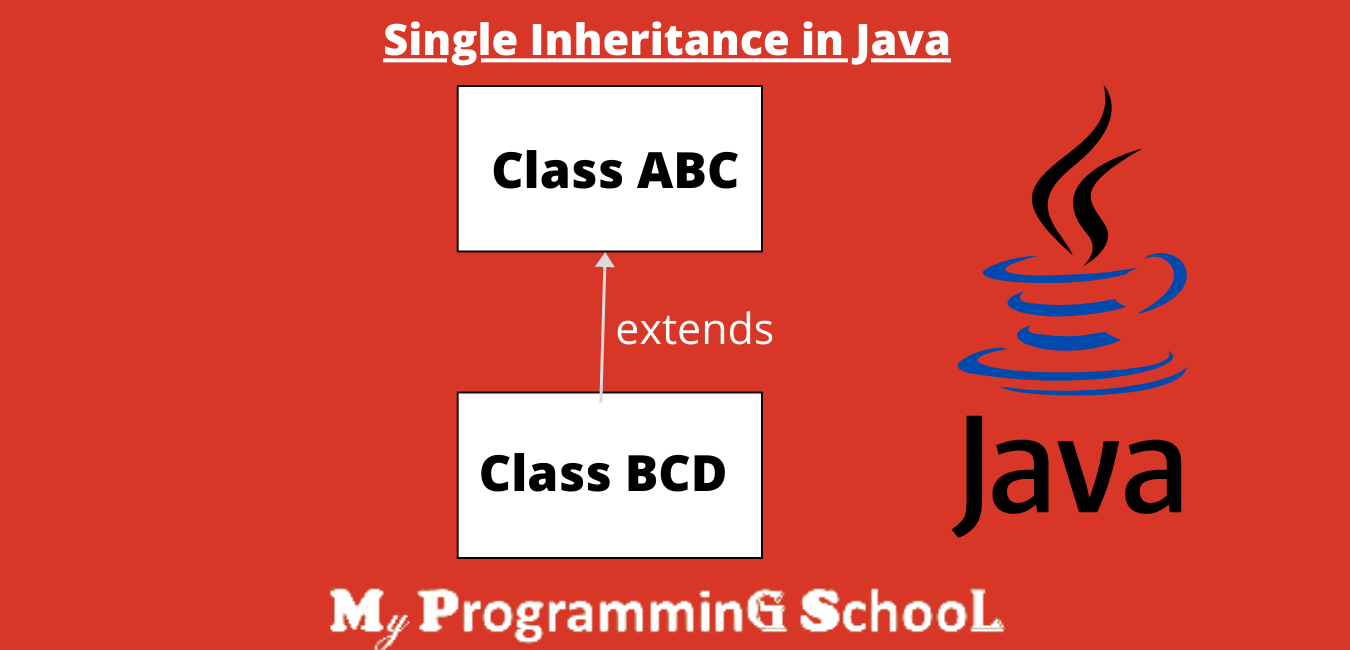
The example below will help you visualize the implementation of single inheritance.
Example of Single Inheritance
class Calculator
{
int add(int a , int b)
{
return a+b;
}
int sub(int a , int b)
{
return a-b;
}
}
public class AdvancedCalculator extends Calculator
{
int mult(int a , int b)
{
return a*b;
}
int div(int a , int b)
{
return a/b;
}
public static void main(String args[])
{
AdvancedCalculator cal= new AdvancedCalculator();
System.out.println(cal.add(2,2));
System.out.println(cal.sub(2,2));
System.out.println(cal.mult(3,2));
System.out.println(cal.div(4,2));
}
}
The superclass AdvancedCalculator uses already defined add and sub-methods and adds more functionality (product and division). Therefore, the output is:
Multiple Inheritance in Java
Multiple Inheritance is one class extending multiple classes. As discussed before, Java does not support Multiple Inheritance. However, with the help of interfaces, we can visualize multiple_Inheritance at work.
Conceptually, a visualization for multiple Inheritance would something like:
Diagram Representation of Multiple Inheritance
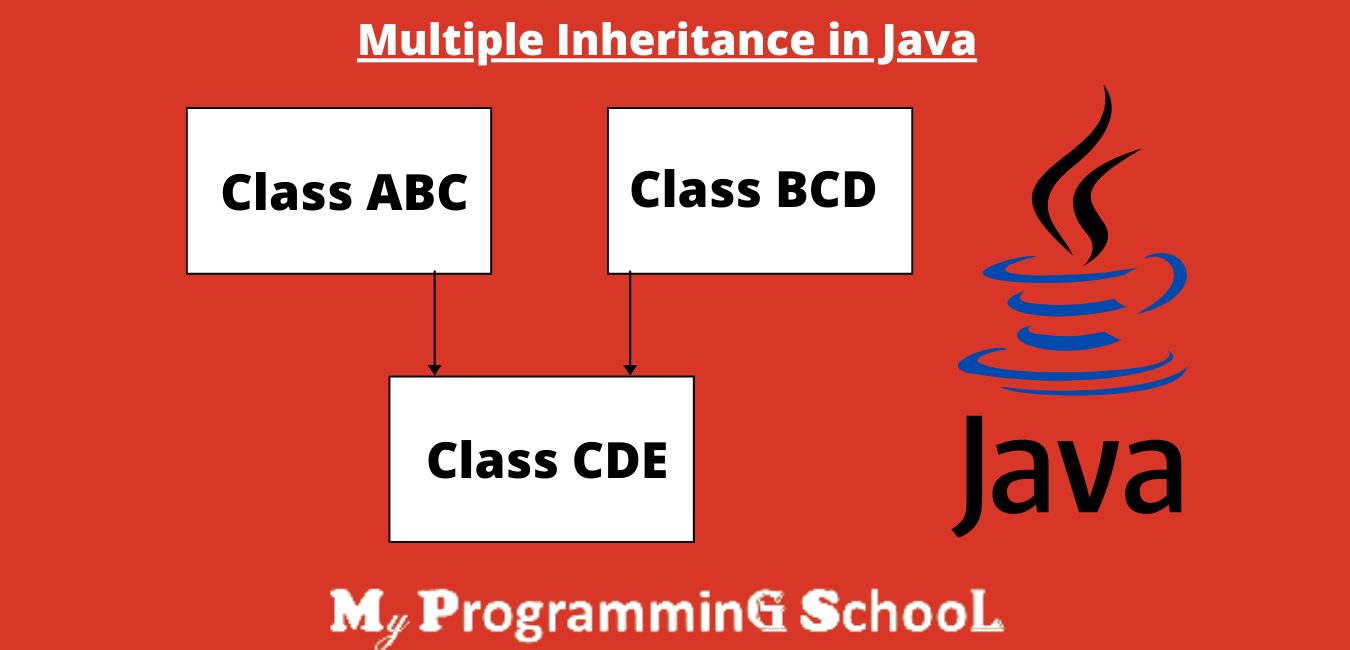
Example of Multiple Inheritance
The example below shows multiple Inheritance through interfaces:
interface ABC
{
public void dis(int num);
}
interface BCD
{
public void disp(int num);
}
class Mult implements ABC, BCD
{
public void dis(int num)
{
System.out.println("From Interface ABC number is " + num);
}
public void disp(int num)
{
System.out.println("From Interface BCD number is " + num);
}
}
public class Test extends Mult
{
public static void main(String args[])
{
Mult ml = new Mult();
ml.dis(10);
ml.disp(20);
}
}
The output is:
Multilevel Inheritance in Java
Multilevel inheritance is about a superclass extending its features to a subclass, which in turn acts as another superclass to a new subclass. Multilevel inheritance is implemented in a hierarchy. This means, once a subclass, will be a future superclass.
Diagram Representation of Multilevel Inheritance
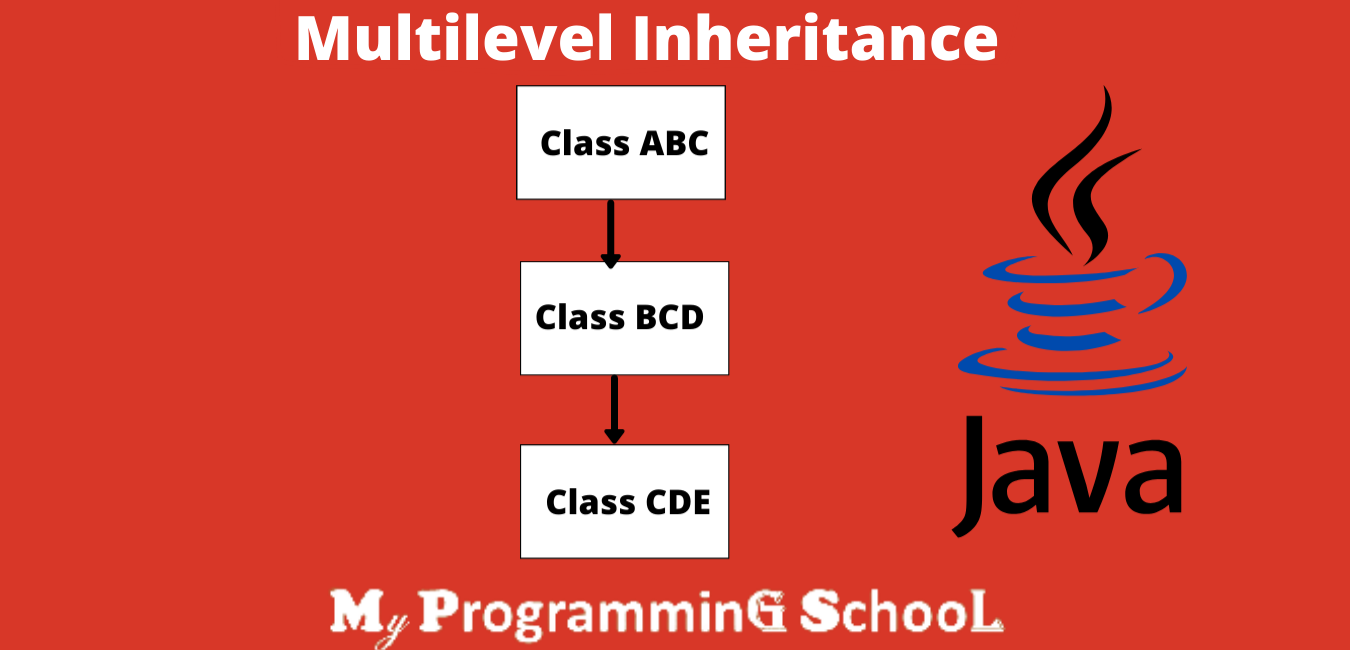
Example of Multilevel Inheritance
The example below is an example of multilevel inheritance:
class ABC { public void disA() { System.out.println("ClassABC"); } } class BCD extends ABC { public void disB() { System.out.println("ClassBCD"); } } class CDE extends BCD { public void disC() { System.out.println("ClassCDE"); } } public clas Multilevel extends CDE { public static void main(String args[]) { C c = new C(); c.disA(); c.disB(); c.disC(); } }
The output is:
Hierarchical Inheritance in Java
In hierarchical inheritance, one class acts as a parent class for multiple subclasses. The visual diagram for hierarchical inheritance is given below:
Diagram Representation of Hierarchical Inheritance
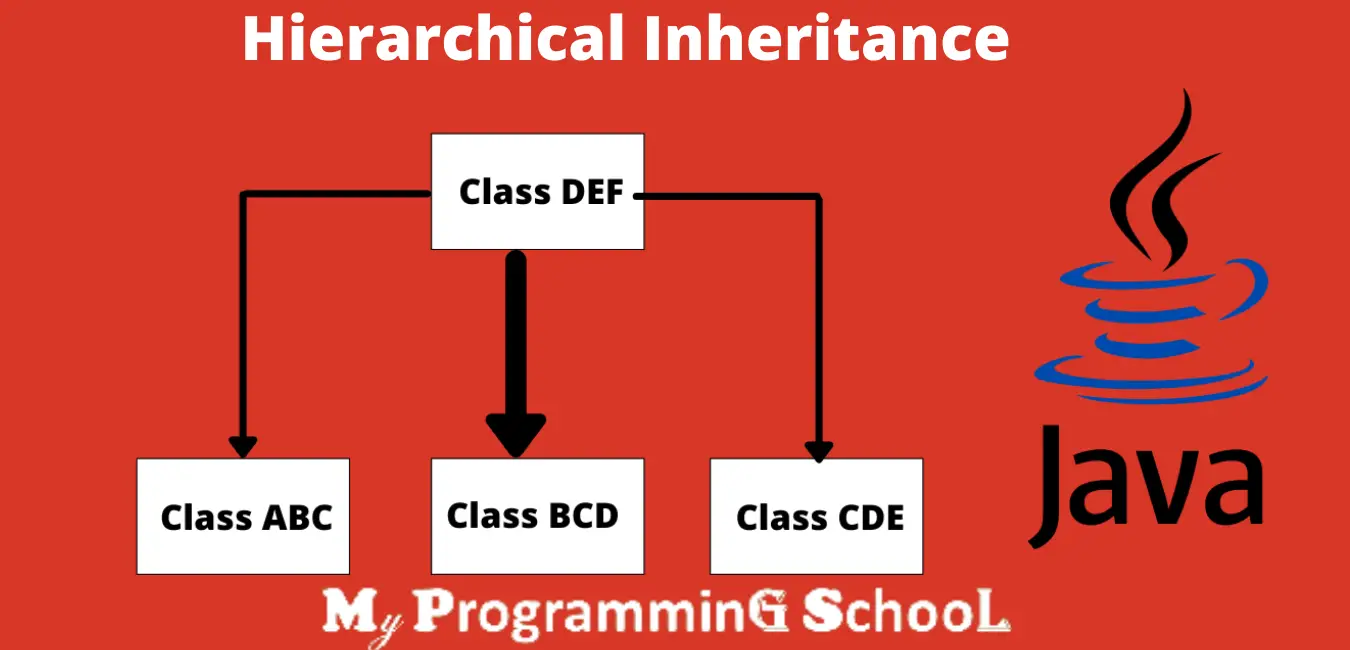
Example of Hierarchical Inheritance
class ABC
{
public void disA()
{
System.out.println("ClassABC");
}
}
class BCD extends ABC
{
public void disB()
{
System.out.println("ClassBCD");
}
}
class CDE extends ABC
{
public void disC()
{
System.out.println("ClassCDE");
}
}
public class Hierarchical extends CDE
{
public static void main(String args[])
{
B b = new B();
b.disB();
b.disA();
C c = new C();
c.disC();
c.disA();
}
}
In the example above class, ABC acts as the parent class for all the sub-classes.
The output is :
Hybrid Inheritance in Java
Hybrid inheritance is formed as a result of the combination of both single and multiple Inheritance. As multiple Inheritance is not viable with classes, we achieve hybrid_inheritance through interfaces.
The visualization for hybrid inheritance is given below:
Diagram Representation of Hybrid Inheritance
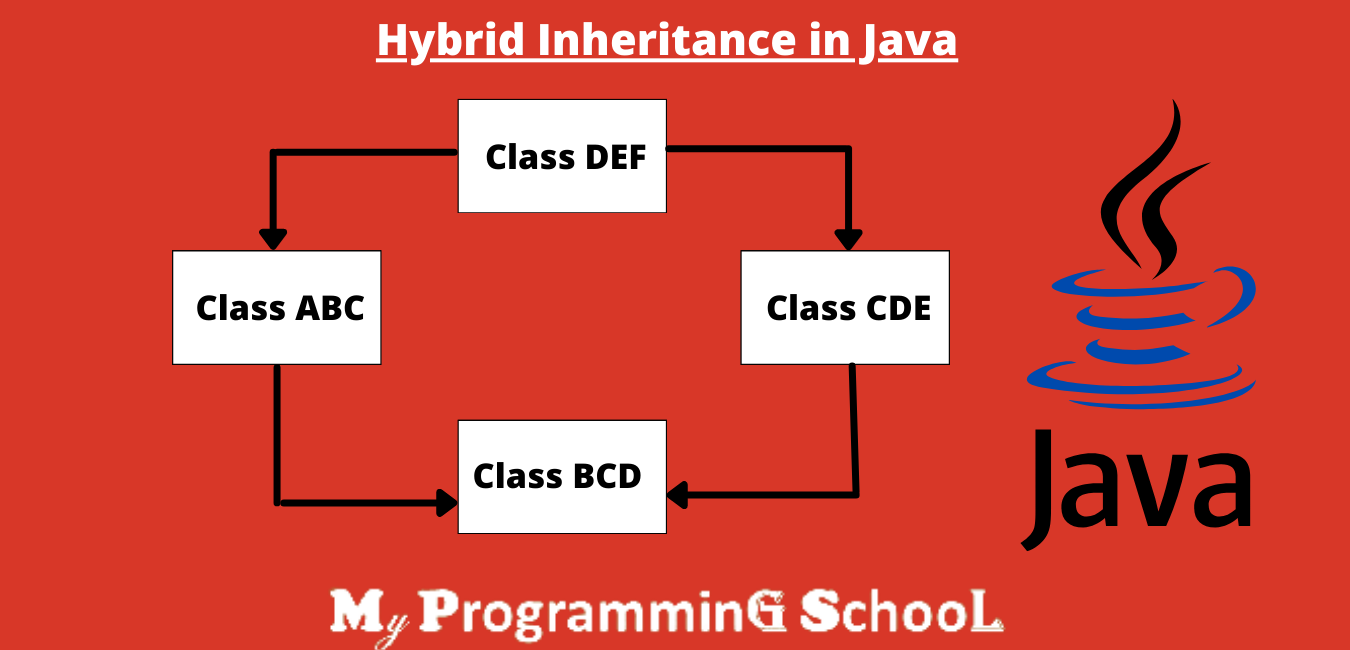
Example of Hybrid Inheritance
interface BCD
{
public void print();
}
interface CDE
{
public void print();
}
class ABC
{
public void disA()
{
System.out.println("ClassABC");
}
}
class DEF extends ABC implements BCD, CDE
{
public void print()
{
System.out.println("method print()");
}
public void disD()
{
System.out.println("ClassDEF");
}
}
public class Jeep extends DEF
{
public static void main(String args[])
{
D obj = new D();
obj.disD();
obj.print();
}
}
The example above Class DEF singly inherits the features of class ABC (single_inheritance) and at the same time extends both interfaces BCD and CDE (Multiple Inheritance)
The output is:
FAQ:
What are the types of inheritance in Java?
There are five types of inheritance in java those are:
Single Inheritance
Multiple Inheritance
Multilevel Inheritance
Hierarchical Inheritance
Hybrid Inheritance
Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order