How to make a dice roll program in Java?
To Write a java program to make rolling a pair of dice 10,000 times and counts the number of times doubles of are rolled for each different pair of doubles. Hint: Math.random()
Description :
For this program, no inputs are required. The output of this program is number of times two dice show similar number when roll dice for 10,000 times.
Here we use, random() method defined in Math class. It picks one number from 1 to 6. The randomly selected numbers are compared, if they are equal, the count increases else it continues.
Algorithm:
- import java package
- import java.io.*;
- import java.util.*;
- Create class Rand
- Create main function
- public static void main(String ar[])
- Declare variable
- int d = 0;
- for( int i = 0; i <= 100; i++)
- Create object
- Random r = new Random();
- int d1 = r.nextInt(6) + 1;
- compare: if( d1 == d2)
- increment d++;
- Create object
- Print The number of times the two roll dice have the same number is
Source code :
import java.io.*;
import java.util.*;
class Rand
{
public static void main(String ar[])
{
int d=0;
for(int i=0;i<=100;i++)
{
Random r=new Random();
int d1=r.nextInt(6)+1;
int d2=r.nextInt(6)+1;
if(d1==d2)
d++;
}
System.out.println("The number of times the two roll dice have same number is "+d);
}
}
Sample output :
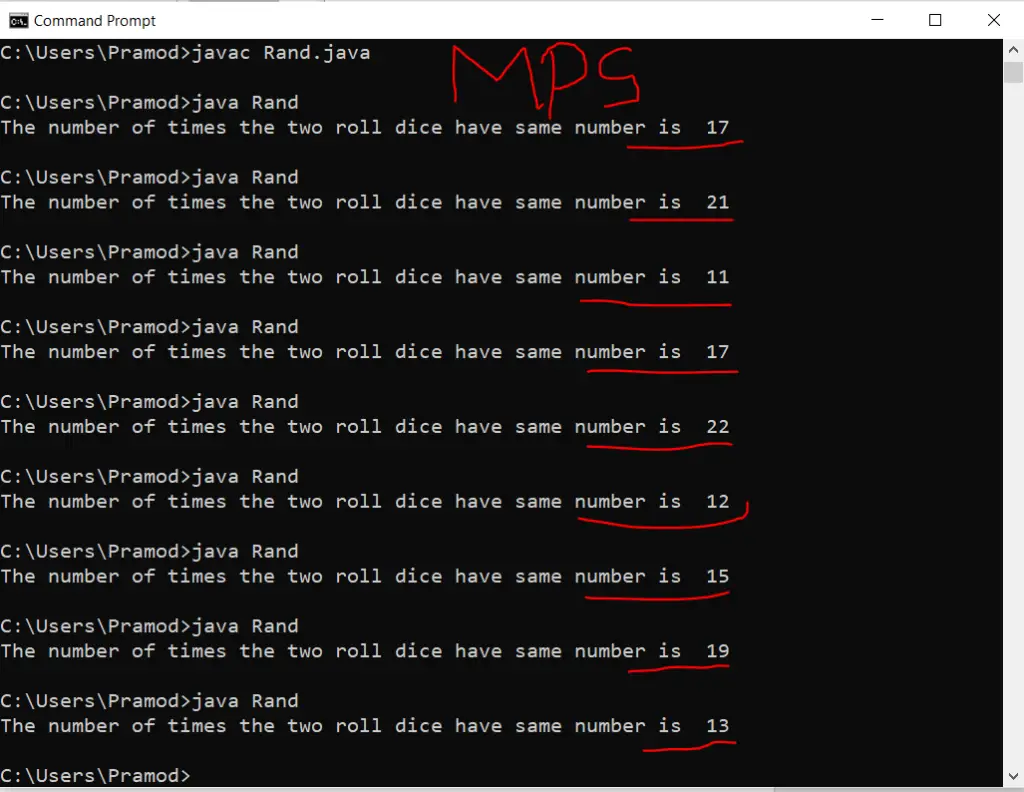
The number of times the two roll dice have same number is 3437
Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Write a java program to Checks whether a given string is a palindrome or not
Find the solution to the salesforce Question and many more
TAGS:
dice roller, dice roller dnd, dice roller online, dice roller calculator, dice roller java, dice roller online free, dice roller average, dice roller multiple