Producer Consumer Problem in Java Using Threads
Aim:
To write a Java program that correctly implements producer-consumer problems using the concept of inter-thread communication.
Description:
The producer-consumer issue (is also known as the bounded buffer issue) is a classic Java Example of a multi-process synchronization issue. The issue describes two processes, the producer and the consumer, who share a typical, fixed-size buffer used as a queue.
The producer’s responsibility is to create a piece of data, put it into the buffer and start once more. At the same time, the consumer is consuming the data (i.e., eliminating it from the buffer) each piece in turn. The issue is to ensure that the producer will do whatever it takes not to add data into the buffer if it’s full and that the consumer will make an effort not to remove data from an empty buffer.
Producer Consumer Solutions: Code
class Producer implements Runnable { Q q; Producer(Q q) { this.q =q; new Thread(this," producer").start(); } public void run() { int i= 0; while(true) { q.put(i++); if(i== 5) System.exit(0); } } } class Consumer implements Runnable { Q q; Consumer(Q q) { this.q= q; new Thread(this, "consumer").start(); } public void run() { while(true) q.get(); } } class Program { public static void main(String ar[]) { Q q= new Q(); new Producer(q); new Consumer(q); } } class Q { int n; boolean valueset= true; synchronized int get() { while(!valueset) { try { wait(); } catch(Exception e) { } } System.out.println("GET " +n); valueset= false; notify(); return n; } synchronized void put(int n) { while(valueset) { try { wait(); } catch(Exception e) { } } this.n= n; valueset =true; System.out.println("PUT " +n); notify(); } }
Sample output :
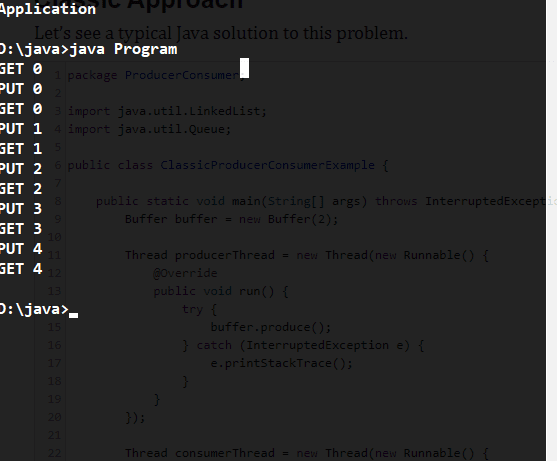
Recommended Post:
- Python Comment | Creating a Comment | multiline comment | example
- Python Dictionary Introduction | Why to use dictionary | with example
- How to do Sum or Addition in python
- Python Reverse number
- find the common number in python
- addition of number using for loop and providing user input data in python
- Python Count char in String
- Python Last Character from String
- Python Odd and Even | if the condition
- Python Greater or equal number
- Python PALINDROME NUMBER
- Python FIBONACCI SERIES
- Python Dictionary | Items method | Definition and usage
- Python Dictionary | Add data, POP, pop item, update method
- Python Dictionary | update() method
- Delete statement, Looping in the list In Python
- Odd and Even using Append in python
- Python | Simple Odd and Even number
Find the solution to the salesforce Question and many more