How to create a thread in Java?
In this program, we will learn to create thread in java with a source and example.
Aim:
To write a Java program that creates three threads. The first thread displays “Good Morning” after every one second, the second thread displays “Hello” after every two seconds, and the third thread displays “Welcome” after every three seconds
Explanation:
In this, we will see how the threads will work in our project. The first thread displays Good Morning every one second, the second thread displays Hello every two seconds and the third thread displays Welcome every three seconds. The technique used to implement threads here is by extending the Thread class.
While executing a thread, if any interrupt occurs then there arises a java exception. In such cases, to handle these exceptions thread statements are kept in try-catch blocks.
Algorithm To Create Threads:
- step: import java.io.*;
- step: Create class A and extends Thread
- step: create string name.
- step: Create class function and pass argument:
- A(String tname)
- step: name = tname
- step: Print name: System.out.print(name)
- step: public void run()
- step: Again Print (name)
- step: Create another class Hellow Thread
- step: Create main function
- public static void main(String ar[])
- step: Create an object: A obj1= new A(“Good Morning”);
- step: Within try block create, Thread.sleep(1000); and close try block.
- step: Same you can follow. Create an object: A obj2= new A(“Hello”); and within a try block create thread.sleep(1000).
- step: End.
Source Code: To Create Thread in java
In the program, we will simply perform to print the message. When you the program please observe the output. It will take 1sec of the gap to print a message and if want to increase the time then you can easily increase the threads time.
import java.io.*;
class A extends Thread
{
String name;
A(String tname)
{
name=tname;
System.out.println(name);
}
public void run()
{
System.out.println(name);
}
}
class HelloThread
{
public static void main(String ar[])
{
A obj1= new A("Good Morning");
try
{
Thread.sleep(1000);
}
catch(Exception ie)
{
System.out.println("Interrupted");
}
A obj2= new A("Hello");
try
{
Thread.sleep(1000);
}
catch(Exception ie)
{
System.out.println("Interrupted");
}
A obj3= new A("Welcome");
try
{
Thread.sleep(1000);
}
catch(Exception ie)
{
System.out.println("Interrupted");
}
}
}
Sample output:
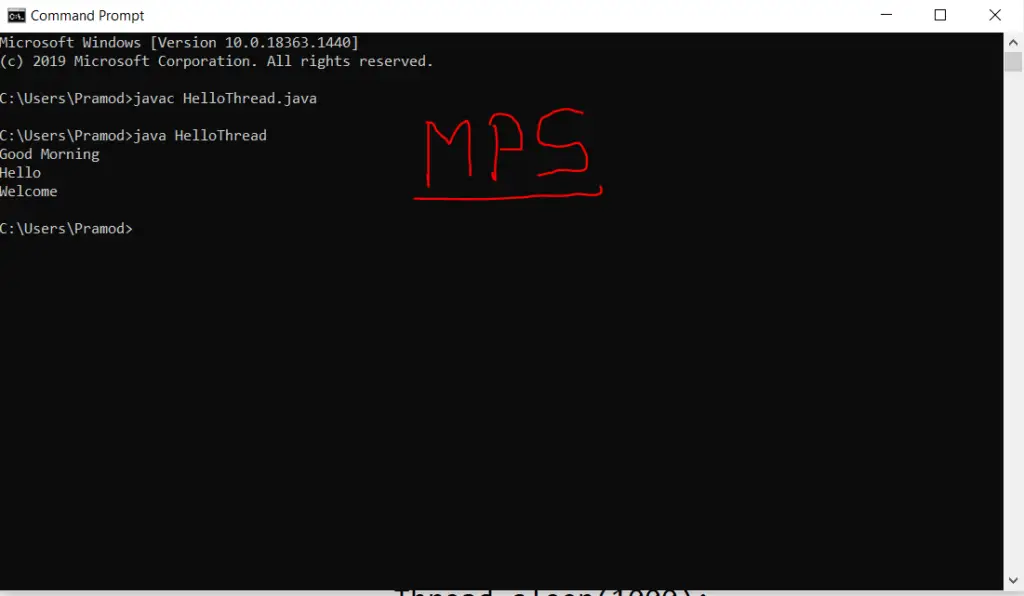
FAQ:
How do you create a thread in Java?
A Thread is created when the Runnable interface is implemented. Thread object should be created and start() method called. The main thread in java will start when the program will execute.
What is thread in Java with example?
In Java thread is nothing but it allows a program to perform more efficiently by doing multiple things at the same time. Threads can be used to complete complex tasks in the background without interrupting the main program.
What is use of thread in Java?
The use of threads in java is to make java applications faster while performing multiple tasks at a time. The thread will help to run multiple programs in a parallel way. Within a program, each of the tasks will run independently.
Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Write a java program to Checks whether a given string is a palindrome or not
- Write a java program to perform multiplication of two matrices
- write a java program that prints the Fibonacci series for a given number.
- Write a Java program that finds the factorial of a number
- Write a Java program that finds prime numbers between 1 to n
- Write a Java program that prints all real and imaginary solutions to the quadratic equation
- Odd and Even number in java | Algorithm
- Even number in java | algorithm with source code
- Java greater number using a loop
- Java Area of Rectangle
- What is a polygon? | Area of Triangle in java
- To calculate the Area of Circle in the java program
- Java Addition through user input
- Addition program in java with source code
Find the solution to the salesforce Question and many more