Python will be of no use without the Datatype. Python Data Types are classified into five categories which are: Numbers, Sequence, Boolean, Set, and Dictionary. From sequence type, we’ll be handling the Python Strings, the Python String literal, Python quotes, and also assigning python strings to variables.
That’s not all, you’ll have a clear understanding of how to access Python String elements and you’ll be enlightened on how to update python String to a new value. In conclusion, you’ll be given some basic python String operators.
You can know more about Python Data Types here
What is Python String?
Python String is a collection of data characters enclosed in quotes. You create a string enclosing collected characters in quotes. It can be single quotes, double quotes, or triple quotes. You execute String creation in Python similarly to assigning a value to a variable. After creation, you can run the string literal by the print( ) function. Python Strings are immutable which means that once a string is created it cannot be modified. Though some functions like replace, join or split can have a slight change on strings, it doesn’t change the primary arrangement, it only serves as a duplicate and falls back home.
Note: String is an in-built python class in Python represented by “str”
Example of strings from single quotes to triple quotes
message = 'This is a single quote string in Python' message = "This is a double quote string" message="'This is a triple quote string'"
The above message is one, anyways, we’ll talk more about triple quotes.
Python String Literal
Python String is made of characters, quotes, and functions. The characters and quotes join to form the string literal.
In the clearest terms, python String Literal are the characters involved in a code line. All the characters that are enclosed in either single quotes, double quotes, or triple quotes are known as String Literal. String Literal can be the numeric, alphabet, or alphanumeric
Examples of some Python String Literal
String Literal on Alphabet:
message = 'the good man with a good heart'
String Literal on number:
value = "1996, 20, 0, 1"
String Literal in alphanumerics:
message = "Simon, born 1999"
Note: the String characters and has been dealt on, next is the quote
Python Quotes
As we already know that quotes are used to form a string in Python, whether single quotes, double quotes, or triple quotes the results remain the same. However, there are times you should use a particular quote to achieve a purpose. Let’s understand the types of quotes in Python.
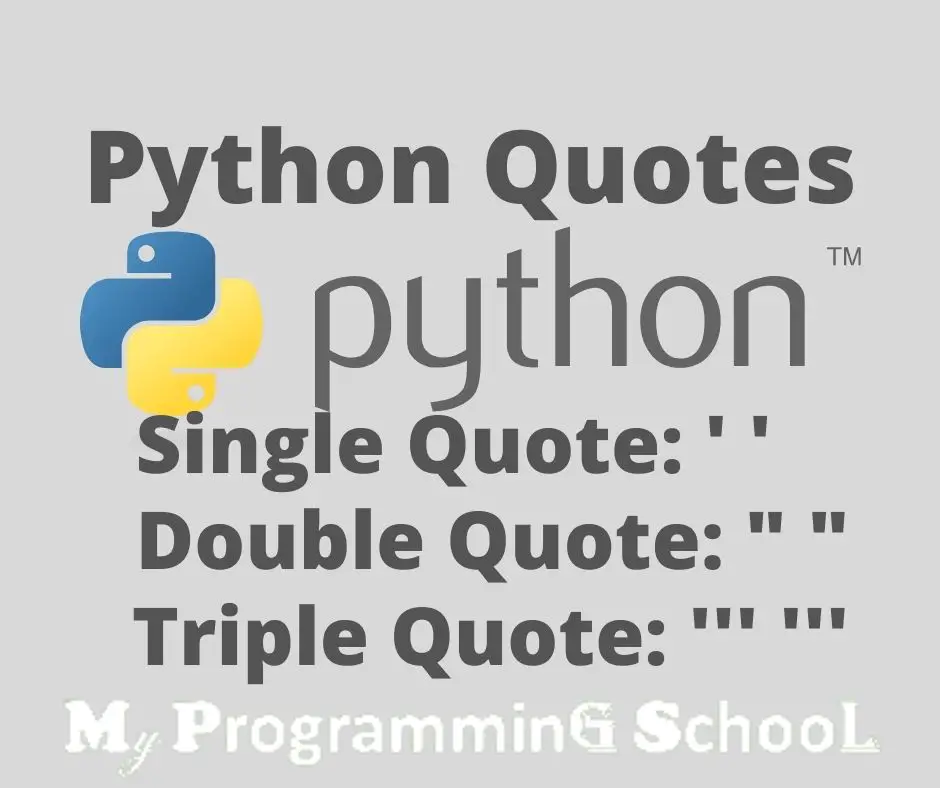
Python Single Quotes
This is how it works: if you want to enclose a double-quoted string within a string, the whole string literal should be enclosed in a single quote.
Example:
>>> var1='Welcome to "Phenomenal academy" Simon Gideon' >>> var1
'Welcome to "Python training" from Phenomenal academy'
From the above coding, Phenomenal academy is the double-quoted string within the whole String Literal, which applies to the second example
Python Double Quotes
Simply and clearly speaking, If you want to enclose a single quote string within a string literal, enclose the whole String within a double quote.
Example:
>>> var2="Welcome to 'Python training' from Phenomenal academy" >>> var2
"Welcome to 'Python training' from Simon"
Note: the first imputing is a double quote enclosing a single quote that responds to the rule of the quotes. Also applicable to the second.
Python Triple Quotes
Triple quotes are used to enclose a python string that covers multiple code lines. The characters involved in these new line coverage can be special characters, Verbatim NEWLINEs, and TABs. Triple String can be created by using triple single quotes (“‘) or triple double quotes (“””). Another thing to note is that
Example of triple quotes strings
''' 1 This string is covering 2 multiple lines 3 within three single 4 quotes on either side. '''
Example 2
""" 1 This string is covering 2 multiple lines 3 within three double 4 quotes on either side. """
Assigning String Values to Variables in Python
Strings on their own won’t run on python, it has to be assigned to a variable for the interpreter to read the message. To be able to assign values to Variables in Python accurately, you must understand its arrangement. The left operand is for the variables while the right operand is for the values assigned to the variables. There’s no command to affect a variable, just introduce the (=) and python will interpret it. You use the print( string) function to enable python to interpret the string.
Example of Python input
var1 = print("Simon says, How are you!") print(var1)
Python Shell
Simon says, How are you?
More interestingly, let’s talk about the things you shouldn’t joke with so long as Python String is concerned
Accessing String Elements:
String Elements are the binary codes that convert to give readability in Python Language. You can access the elements of strings using an index. Do the following to access string Elements
str = "Gideon Monday" print(str[0]) # p print(str[1]) # m
- Assign a value to a variable
- Write the first and second character of the string next to a comment # using a square box [ ] and indices inside
Also, using a negative index will prompt python to return the end of the string like this
str = "Gideon Monday" print(str[-1]) # y print(str[-2]) # a
How python will display the index
+---+---+---+---+---+---+---+---+---+---+---+---+---+ | G | i | d | e | o | n | | M | o | n | d | a | y | +---+---+---+---+---+---+---+---+---+---+---+---+---+ 0 1 2 3 4 5 6 7 8 9 10 11 12 -12 -11 -10 -9 -8 -7 -6 -5 -4 -3 -2 -1 0
Python String Length
Python String Length is simply how many characters make up a string literal. Keep in mind that the white space in between is included. To get the length of a string, you use the Len() function
Example:
Input
str = "Gideon Monday" str_len = len(str) print(str_len)
Output: 13
Python String Updating
String Update is simply reassigning a variable to another String
It is causing a variable to give another String literal as output
Example:
Input
#!/usr/bin/python3 var1 = 'Hello World!' print ("Updated String :- ", var1[:6] + 'Tanya!')
Output
Updated String:- Hello Tanya!
From the above, the world was upgraded to Tanya.
We save the best part for the last
There’s something that should ever go in handy with you in your python journey.
Python String Operators
Take a = ‘Hello’ and take b = ‘Tanya’
Then :
Operator | Meaning | Example |
+ | Concatenation – Adds values on either side of the operator | The result will be: Hello Tanya |
* | Repetition – creates new strings, concatenating multiple copies of the same string | a*2 will give -HelloHello |
[] | Slice – Gives the character from the given index | a[1] will give e |
[ : ] | Range Slice – Gives the characters from the given range | a[1:4] will give all |
in | Membership – Returns true if a character exists in the given string | H in a will give 1 |
not in | Membership – Returns true if a character does not exist in the given string | M not in a will give 1 |
r/R | Raw String – Overwrites the normal meaning of Escape characters. The syntax for raw strings is the same as for normal strings except for the raw string operator, the letter “r,” precedes the quotation marks. The “r” can be lowercase (r) or uppercase (R) and must be placed immediately preceding the first quote mark. | print r’\a’ prints \a and print R’\a’prints \a |
% | Format – Performs String formatting | For format and conversation, it’s broad |
Summary
Python String is a collection of data characters enclosed in quotes. You create a string enclosing collected characters in quotes. It can be single quotes, double quotes, or triple quotes.
Note: String is an in-built python class in Python represented by “str”
Python String is made of characters, quotes, and functions. The characters and quotes join to form the string literal.
As we already know that quotes are used to form a string in Python, whether single quotes, double quotes, or triple quotes the results remain the same.
Strings on their own won’t run on python, it has to be assigned to a variable for the interpreter to read the message.
To be able to assign values to Variables in Python accurately, you must understand its arrangement. The left operand is for the variables while the right operand is for the values assigned to the variables. There’s no command to affect a variable, just introduce the (=), and python will interpret it. You use the print( string) function to enable python to interpret the string
In this class, you’ve learned just enough to get you all out for python strings fully chested. As always the resolve, knowledge won’t practice itself, put your knowledge to practice. Get to work!