Find the area of triangle and area of rectangle in java
To write a java program to create a superclass called Figure that receives the dimensions of two-dimensional objects. It also defines a method called area that computes the area of an object. The program derives two subclasses from Figure. The first is Rectangle and the second is Triangle. Each of the sub-class overridden area() so that it returns the area of a rectangle and a triangle respectively.
Description :
create a superclass called Figure that receives the dimensions of two-dimensional objects. It also defines a method called area that computes the area of a triangle of an object. The program derives two subclasses from Figure. The first is Rectangle and the second is Triangle. Each of the sub-class overridden area() so that it returns the area of a triangle and a rectangle respectively.
Java Program to Calculate Area of Triangle Source Code
import java.io. *; class Figure { int l, w; Figure(int a, int b) { l = a; w = b; } void area() { System.out.println("Area of the Figure"); } } class Triangle extends Figure { Triangle(int g, int h) { super(g, h); } void area() { System.out.println("Area of triangle " + (l * w) / 2); } } class A { public static void main(String ar[]) { Rectangle obj1 = new Rectangle(20, 20); obj1.area(); } }
Output: Area of Triangle
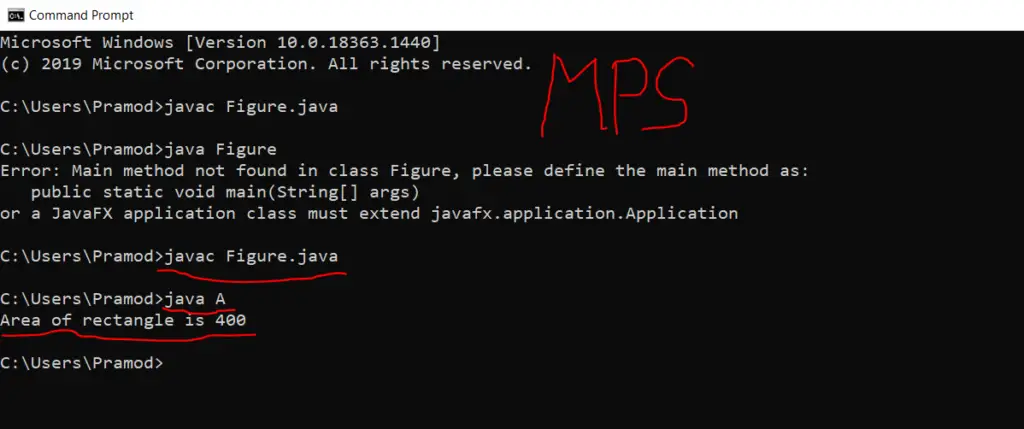
Java Program to Calculate Area of Rectangle Source Code
import java.io.*;
class Figure
{
int l, w;
Figure(int a, int b)
{
l = a;
w = b;
}
void area()
{
System.out.println("Area of the Figure");
}
}
class Rectangle extends Figure
{
Rectangle(int k,int l)
{
super(k,l);
}
void area()
{
System.out.println("Area of rectangle is "+l*w);
}
}
class A
{
public static void main(String ar[])
{
Triangle obj2 = new Triangle(20, 20);
obj2.area();
}
}
Output: Area of Rectangle
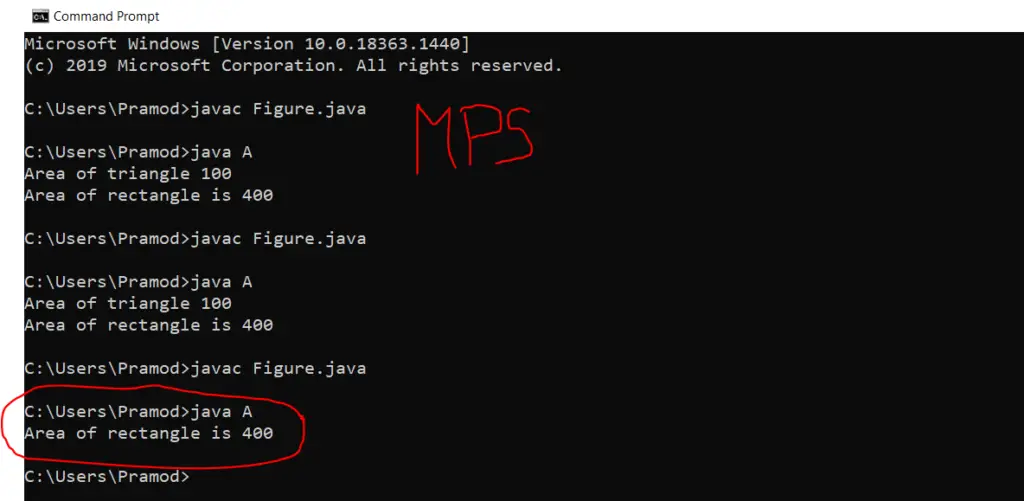
FAQ:
How do you find the area of a rectangle in Java?
To find the area of a rectangle, we have to know the formula to find the area of a rectangle and that is Area = ( l * w ), where L is the length and w is the width.
How do you find the area of a triangle in Java?
To find the area of a triangle, we have to know the formula to find the area of a triangle and that is Area = ( b * h ) / 2, Where b is the base and h is the height.
What is the formula for area of a Triangle?
The formula for the area of a triangle is: Area = ( b * h ) / 2, Where b is the base and h is the height.
What is the formula for area of a Rectangle?
The formula for the area of a rectangle is: Area = ( l * w ), where L is the length and w is the width.
Recommended Post:
- How to compare dates in java|algorithm with source code
- Java roll dice 10000 times with algorithm and source code
- Write a Java program that displays the number of characters, lines, and words in a text
- Write a Java program that reads a file and displays the file on the screen with a line number before each line
- Write a Java program that reads a file name from the user, then displays information about whether the file exists, readable, writable, type of file, and the length of the file in bytes
- Java program to make frequency count of vowels, consonants, special symbols, digits, words in a given text
- Write a Java program for sorting a given list of names in ascending order
- Write a java program to Checks whether a given string is a palindrome or not
- Write a java program to perform multiplication of two matrices
- write a java program that prints the Fibonacci series for a given number.
- Write a Java program that finds the factorial of a number
- Write a Java program that finds prime numbers between 1 to n
- Write a Java program that prints all real and imaginary solutions to the quadratic equation
- Odd and Even number in java | Algorithm
- Even number in java | algorithm with source code
- Java greater number using a loop
- Java Area of Rectangle
- What is a polygon? | Area of Triangle in java
- To calculate the Area of Circle in the java program
- Java Addition through user input
- Addition program in java with source code
Find the solution to the salesforce Question and many more